Bart Jacobs [Jacobs - Mastering Core Data With Swift: Update for Xcode 9 and Swift 4
Here you can read online Bart Jacobs [Jacobs - Mastering Core Data With Swift: Update for Xcode 9 and Swift 4 full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2017, publisher: leanpub.com, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
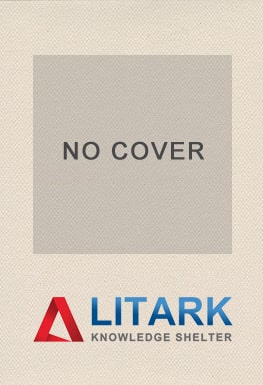
- Book:Mastering Core Data With Swift: Update for Xcode 9 and Swift 4
- Author:
- Publisher:leanpub.com
- Genre:
- Year:2017
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
Mastering Core Data With Swift: Update for Xcode 9 and Swift 4: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Mastering Core Data With Swift: Update for Xcode 9 and Swift 4" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Bart Jacobs [Jacobs: author's other books
Who wrote Mastering Core Data With Swift: Update for Xcode 9 and Swift 4? Find out the surname, the name of the author of the book and a list of all author's works by series.
Mastering Core Data With Swift: Update for Xcode 9 and Swift 4 — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Mastering Core Data With Swift: Update for Xcode 9 and Swift 4" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
This book is for sale at http://leanpub.com/mastering-core-data-with-swift
This version was published on 2017-07-13
* * * * *
This is a Leanpub book. Leanpub empowers authors and publishers with the Lean Publishing process. Lean Publishing is the act of publishing an in-progress ebook using lightweight tools and many iterations to get reader feedback, pivot until you have the right book and build traction once you do.
* * * * *
Welcome to Mastering Core Data With Swift. In this book, youll learn the ins and outs of Apples popular Core Data framework. Even though well be building an iOS application, the Core Data framework is available on iOS, tvOS, macOS, and watchOS, and the contents of this book apply to each of these platforms.
In this book, we use Xcode 9 and Swift 4. Xcode 8 and Swift 3 introduced a number of significant improvements that make working with Core Data more intuitive and more enjoyable. Make sure to have Xcode 8 or Xcode 9 installed to follow along. Everything you learn in this book applies to both Swift 3 and Swift 4.
Before we start writing code, we take a look at the Core Data framework itself. We find out what Core Data is and isnt, and we explore the heart of every Core Data application, the Core Data stack.
In this book, we build Notes, an iOS application that manages a list of notes. Notes is a simple iOS application, yet it contains all the ingredients we need to learn about the Core Data framework, from creating and deleting records to managing many-to-many relationships.
We also take a close look at the brains of a Core Data application, the data model. We discuss data model versioning and migrations. These concepts are essential for every Core Data application.
Core Data records are represented by managed objects. You learn how to create them, fetch them from a persistent store, and delete them if theyre no longer needed.
Mastering Core Data With Swift also covers a few more advanced topics. Even though these topics are more advanced, theyre essential if you work with Core Data. We talk in detail about the NSFetchedResultsController
class and, at the end of this book, I introduce you to the brand new NSPersistentContainer
class, a recent addition to the framework.
Last but not least, we take a deep dive into Core Data and concurrency, an often overlooked topic. This is another essential topic for anyone working with Core Data. Dont skip this.
Thats a lot to cover, but Im here to guide you along the way. If you have any feedback or questions, reach out to me via email (bart@cocoacasts.com) or Twitter (@_bartjacobs). Im here to help.
If youd like to follow along, I recommend downloading the source files that come with this book. The chapters that include code each have a starter project and a finished project. This makes it easy to follow along or pick a random chapter from the book. Click here to download the source files for this book.
If youre new to Core Data, then I recommend reading every chapter of the book. Over the years, I have taught thousands of developers about the Core Data framework. From that experience, I developed a roadmap for teaching Core Data. This book is the result of that roadmap.
Not everyone likes books. If you prefer video, then you may be interested in a video course in which I teach the Core Data framework. The content is virtually identical. The only difference is that you can see how I build Notes using the Core Data framework. You can find the video course on the Cocoacasts website.
Developers new to Core Data often dont take the time to learn about the framework. Not knowing what Core Data is, makes it hard and frustrating to wrap your head around the ins and outs of the framework. Id like to start by spending a few minutes exploring the nature of Core Data and, more importantly, explain to you what Core Data is and isnt.
Core Data is a framework developed and maintained by Apple. Its been around for more than a decade and first made its appearance on macOS with the release of OS X Tiger in 2005. In 2009, the company made the framework available on iOS with the release of iOS 3. Today, Core Data is available on iOS, tvOS, macOS, and watchOS.
Core Data is the M in MVC, the model layer of your application. Even though Core Data can persist data to disk, data persistence is actually an optional feature of the framework. Core Data is first and foremost a framework for managing an object graph.
Youve probably heard and read about Core Data before taking this course. That means that you may already know that Core Data is not a database and that it manages your applications object graph. Both statements are true. But what do they really mean?
Remember that Core Data is first and foremost an object graph manager. But what is an object graph?
An object graph is nothing more than a collection of objects that are connected with one another. The Core Data framework excels at managing complex object graphs.
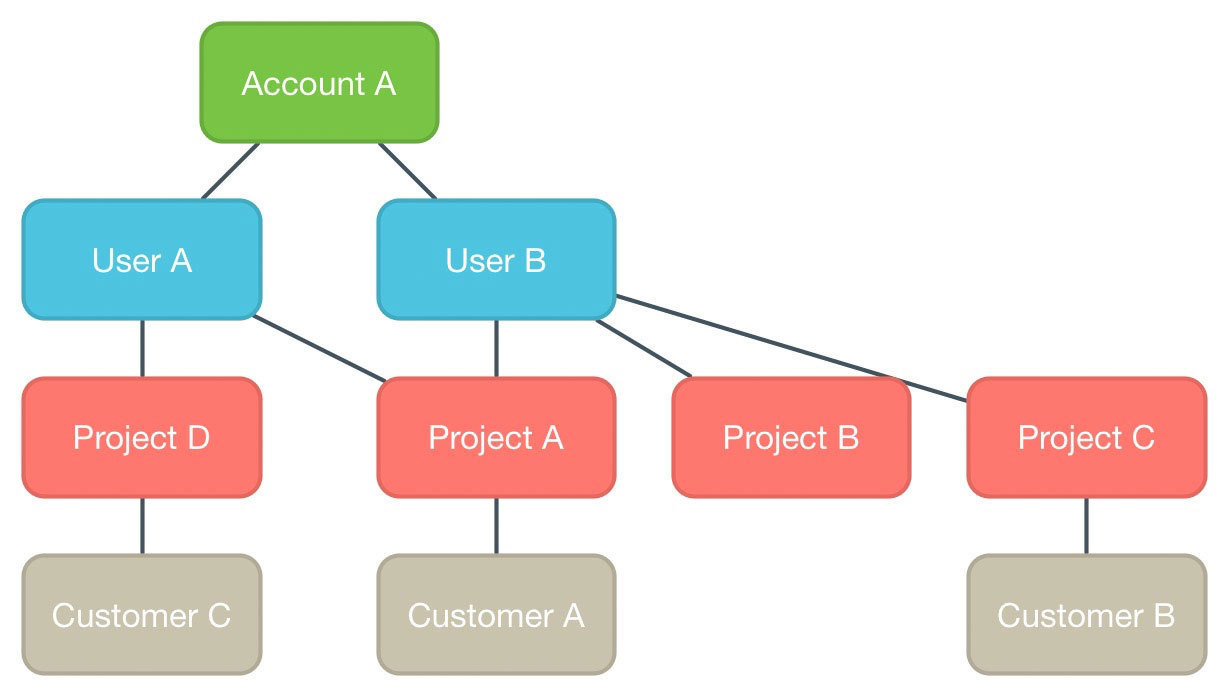
The Core Data framework takes care of managing the life cycle of the objects in the object graph. It can optionally persist the object graph to disk and it also offers a powerful interface for searching the object graph it manages.
But Core Data is much more than that. The framework adds a number of other compelling features, such as input validation, data model versioning, and change tracking.
Even though Core Data is a perfect fit for a wide range of applications, not every application should use Core Data.
If youre in need of a lightweight model layer, then Core Data shouldnt be your first choice. There are many, lightweight libraries that provide this type of functionality.
And if youre looking for a SQLite wrapper, then Core Data is also not what you need. For a lightweight, performant SQLite wrapper, I highly recommend Gus Muellers FMDB. This robust, mature library provides an object-oriented interface for interacting with SQLite.
Core Data is an excellent choice if you want a solution that manages the model layer of your application. Developers new to Core Data are often confused by the differences between SQLite and Core Data.
If you wonder whether you need Core Data or SQLite, youre asking the wrong question. Remember that Core Data is not a database.
SQLite is a lightweight database thats incredibly performant, and, therefore, a good fit for mobile applications. Even though SQLite is advertised as a relational database, its important to realize that the developer is in charge of maintaining the relationships between records stored in the database.
Core Data provides an abstraction that allows developers to interact with the model layer in an object-oriented manner. Every record you interact with is an object.
Font size:
Interval:
Bookmark:
Similar books «Mastering Core Data With Swift: Update for Xcode 9 and Swift 4»
Look at similar books to Mastering Core Data With Swift: Update for Xcode 9 and Swift 4. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Mastering Core Data With Swift: Update for Xcode 9 and Swift 4 and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.