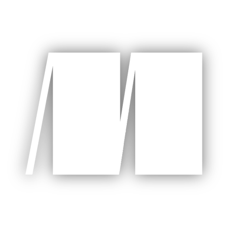
Haskell in Depth
Vitaly Bragilevsky
Foreword by Simon Peyton Jones
To comment go to liveBook
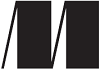
Manning
Shelter Island
For more information on this and other Manning titles go to
www.manning.com
Copyright
For online information and ordering of these and other Manning books, please visit www.manning.com. The publisher offers discounts on these books when ordered in quantity.
For more information, please contact
Special Sales Department
Manning Publications Co.
20 Baldwin Road
PO Box 761
Shelter Island, NY 11964
Email: orders@manning.com
2021 by Manning Publications Co. All rights reserved.
No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by means electronic, mechanical, photocopying, or otherwise, without prior written permission of the publisher.
Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in the book, and Manning Publications was aware of a trademark claim, the designations have been printed in initial caps or all caps.
Recognizing the importance of preserving what has been written, it is Mannings policy to have the books we publish printed on acid-free paper, and we exert our best efforts to that end. Recognizing also our responsibility to conserve the resources of our planet, Manning books are printed on paper that is at least 15 percent recycled and processed without the use of elemental chlorine.
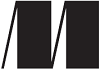
| Manning Publications Co. 20 Baldwin Road Technical PO Box 761 Shelter Island, NY 11964 |
Development editor: | Jenny Stout |
Technical development editor: | Marcello Seri |
Review editor: | Aleksandar Dragosavljevi |
Production editor: | Lori Weidert |
Copy editor: | Katie Tennant |
Proofreader: | Melody Dolab |
Technical proofreader: | Alexander Vershilov |
Typesetter: | Gordan Salinovi |
Cover designer: | Marija Tudor |
ISBN: 9781617295409
dedication
To my mother
front matter
foreword
Many introductory books on Haskell are out there, as well as lots of online tutorials, so the first steps in learning Haskell are readily available. But what happens after that? Haskell has a low floor (anyone can learn elementary Haskell) but a stratospherically high ceiling. Haskell is a uniquely malleable medium: its support for abstraction, thorough algebraic data types, higher kinds, type classes, type families, and so on is remarkable. But this power and flexibility can be daunting. What are we to make of the following:
traverse :: Applicative f => (a -> f b) -> t a -> f (t b)
What are f
and t
? What on earth does this function do? What is Applicative
, anyway? Its all too abstract!
Becoming a power user of Haskell means getting a grip on abstractions like these, not as a piece of theory, but as living, breathing code that does remarkably useful stuff. As we learn these abstractions and see how they work, we realise they are not baked inthey are just librariesso we can build new abstractions of our own, implemented in libraries.
This book exposes you to many of these techniques. It covers many of the more sophisticated parts of the language: not just type classes, but existentials, GADTs, type families, kinds and kind polymorphism, deriving, metaprogramming, and so on. It describes many of the key abstractions (Functor
, Applicative
, Traversable
, etc.) and a carefully chosen set of libraries (for parsing, database, web frameworks, streaming, and data-type-generic programming). As well as being useful in their own right, each part illustrates in a concrete way how Haskells features can be combined in powerful and unexpected ways.
Finally, the book covers aspects of software engineering. How do you design a functional program? How do you test it? How do you benchmark it? What error handling is appropriate? These classic issues show up in rather different guises when you are thinking about functional programming.
Functional programming lets you think big thoughts. It reduces the brain-to-code distance by allowing you to program at a very high level. We are still learning what those high-level abstractions should be. This book will help put you in the vanguard of that journey.
Simon Peyton Jones, Senior Principal researcher at Microsoft Research, Cambridge, England
preface
The history of Haskell started more than 30 years ago, in 1987 (see A History of Haskell: Being Lazy with Class at https://www.microsoft.com/en-us/research/publication/ a-history-of-haskell-being-lazy-with-class/ for many exciting details). Nowadays, Haskell is a mature programming language. It is full of features and has a stable implementation, the Glasgow Haskell Compiler, a helpful and friendly community, and a big ecosystem.
Paraphrasing the Haskell 2010 Language Report ( https://www.haskell.org/ onlinereport/haskell2010/ ), which is an effective standard description of the Haskell language, we can give it the following definition.
Haskell is a general-purpose, purely functional programming language featuring higher-order functions, nonstrict semantics, static polymorphic typing, user-defined algebraic data types, pattern matching, a module system, a monadic I/O system, and a rich set of primitive data types (including lists, arrays, arbitrary- and fixed-precision integers, and floating-point numbers).
This definition is feature centric but gives a little information about how to use all these features professionally. Haskell is by far not the most popular programming language in the world, however. Two unfortunate myths contribute a lot to its limited adoption:
I believe that both of these claims are false. In fact, we can use Haskell in production without learning and doing math by ourselves. The truth is that the deep mathematical concepts behind the language itself give us a tool that can be used to write flexible, expressive, and performant code that is resilient to frequent changes in requirements, well suited to massive refactoring, and less prone to mistakes. If you like these software qualities, then Haskell is definitely for you and your team.
When talking about any programming language in general and its use in industry, we usually discuss the following components:
Language features, programming style, and how they affect one another
The set of libraries (packages) available to developers and their distribution
The tooling that forms a convenient programming environment
Figure 1 presents these components for the Haskell programming language. They form a language ecosystem and make building software for the real world possible.
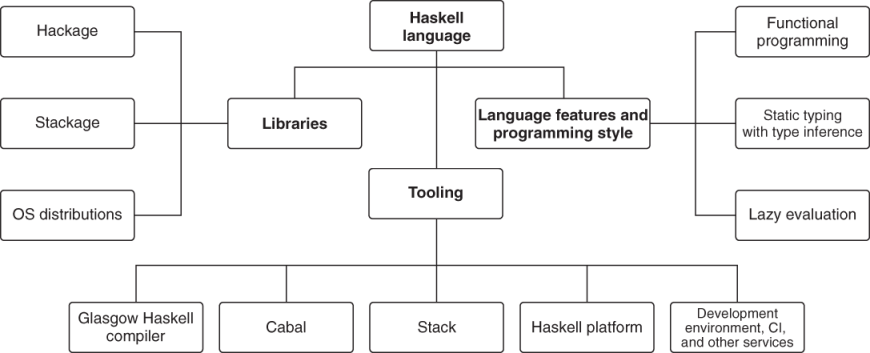
Next page