Cay S. Horstmann - Core Java for the Impatient, 3rd Edition
Here you can read online Cay S. Horstmann - Core Java for the Impatient, 3rd Edition full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2022, publisher: Addison-Wesley Professional, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
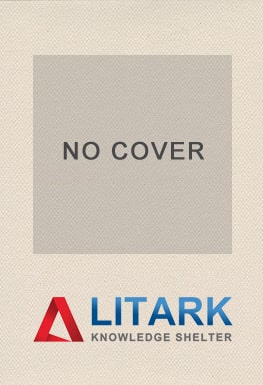
- Book:Core Java for the Impatient, 3rd Edition
- Author:
- Publisher:Addison-Wesley Professional
- Genre:
- Year:2022
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
Core Java for the Impatient, 3rd Edition: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Core Java for the Impatient, 3rd Edition" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Core Java for the Impatient, 3rd Edition — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Core Java for the Impatient, 3rd Edition" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
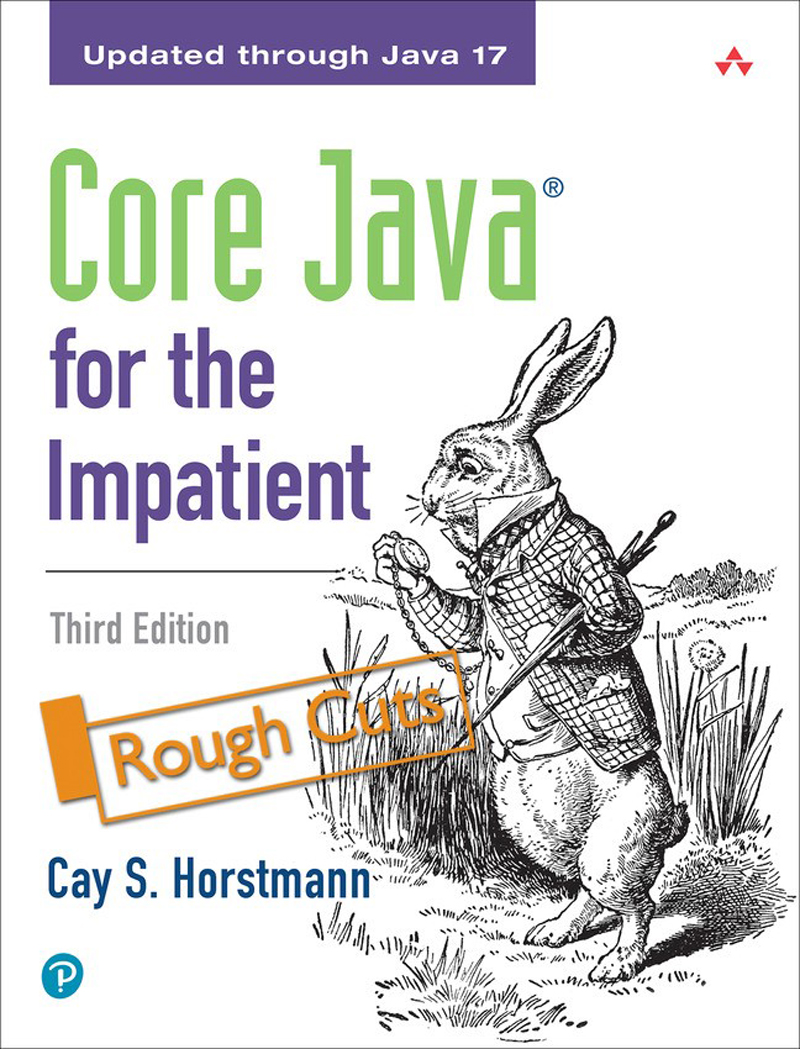
Third Edition
Cay S. Horstmann
Addison-Wesley Professional
Java is now well over twenty years old. The classic book, Core Java, covers, in meticulous detail, not just the language but all core libraries and a multitude of changes between versions, spanning two volumes and over 2,000 pages. However, if you just want to be productive with modern Java, there is a much faster, easier pathway for learning the language and core libraries. In this book, I dont retrace history and dont dwell on features of past versions. I show you the good parts of Java as it exists today, so you can put your knowledge to work quickly.
As with my previous Impatient books, I quickly cut to the chase, showing you what you need to know to solve a programming problem without lecturing about the superiority of one paradigm over another. I also present the information in small chunks, organized so that you can quickly retrieve it when needed.
Assuming you are proficient in some other programming language, such as C++, JavaScript, Objective C, PHP, or Ruby, with this book you will learn how to become a competent Java programmer. I cover all aspects of Java that a developer needs to know today, including the powerful concepts of lambda expressions and streams, as well as modern constructs such as records and sealed classes.
A key reason to use Java is to tackle concurrent programming. With parallel algorithms and threadsafe data structures readily available in the Java library, the way application programmers should handle concurrent programming has completely changed. I provide fresh coverage, showing you how to use the powerful library features instead of error-prone low-level constructs.
Traditionally, books on Java have focused on user interface programmingbut nowadays, few developers produce user interfaces on desktop computers. If you intend to use Java for server-side programming or Android programming, you will be able to use this book effectively without being distracted by desktop GUI code.
Finally, this book is written for application programmers, not for a college course and not for systems wizards. The book covers issues that application programmers need to wrestle with, such as logging and working with filesbut you wont learn how to implement a linked list by hand or how to write a web server.
I hope you enjoy this rapid-fire introduction into modern Java, and I hope it will make your work with Java productive and enjoyable.
If you find errors or have suggestions for improvement, please visit http://horstmann.com/javaimpatient
, head for the errata page, and leave a comment. On that site, you will also find a link to an archive file containing all code examples from the book.
This content is currently in development.
This content is currently in development.
In this chapter, you will learn about the basic data types and control structures of the Java language. I assume that you are an experienced programmer in some other language and that you are familiar with concepts such as variables, loops, function calls, and arrays, but perhaps with a different syntax. This chapter will get you up to speed on the Java way. I will also give you some tips on the most useful parts of the Java API for manipulating common data types.
The key points of this chapter are:
1. In Java, all methods are declared in a class. You invoke a nonstatic method on an object of the class to which the method belongs.
2. Static methods are not invoked on objects. Program execution starts with the static main
method.
3. Java has eight primitive types: four signed integral types, two floating-point types, char
, and boolean
.
4. The Java operators and control structures are very similar to those of C or JavaScript.
5. There are four forms of switch
: expressions and statements with and without fall through.
6. The Math
class provides common mathematical functions.
7. String
objects are sequences of characters or, more precisely, Unicode code points in the UTF-16 encoding.
8. Use the text box syntax to declare multiline string literals.
9. With the System.out
object, you can display output in a terminal window. A Scanner
tied to System.in
lets you read terminal input.
10. Arrays and collections can be used to collect elements of the same type.
When learning any new programming language, it is traditional to start with a program that displays the message Hello, World!. That is what we will do in the following sections.
Without further ado, here is the Hello, World program in Java.
package ch01.sec01;// Our first Java programpublic class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); }}Lets examine this program:
Java is an object-oriented language. In your program, you manipulate (mostly) objects by having them do work. Each object that you manipulate belongs to a specific class, and we say that the object is an instance of that class. A class defines what an objects state can be and and what it can do. In Java, all code is defined inside classes. We will look at objects and classes in detail in . This program is made up of a single class
HelloWorld
.
main
is a method, that is, a function declared inside a class. The main
method is the first method that is called when the program runs. It is declared as static
to indicate that the method does not operate on any objects. (When main
gets called, there are only a handful of predefined objects, and none of them are instances of the HelloWorld
class.) The method is declared as void
to indicate that it does not return any value. See for the meaning of the parameter declaration String[] args
.
In Java, you can declare many features as
public
or private
, and there are a couple of other visibility levels as well. Here, we declare the HelloWorld
class and the main
method as public
, which is the most common arrangement for classes and methods.
A package is a set of related classes. It is a good idea to place each class in a package so you can group related classes together and avoid conflicts when multiple classes have the same name. In this book, well use chapter and section numbers as package names. The full name of our class is
ch01.sec01.HelloWorld
. has more to say about packages and package naming conventions.
The line starting with
//
is a comment. All characters between //
and the end of the line are ignored by the compiler and are meant for human readers only.
Font size:
Interval:
Bookmark:
Similar books «Core Java for the Impatient, 3rd Edition»
Look at similar books to Core Java for the Impatient, 3rd Edition. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Core Java for the Impatient, 3rd Edition and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.