If youd like to report any bugs, typos, or suggestions just email us at: .
For further help dealing with issues, refer to How to Get the Most Out of This Book.
If youd like to be notified of updates to the book on Twitter, follow @fullstackio
Did you like the book? Did you find it helpful? Wed love to add your face to our list of testimonials on the website! Email us at: .
Foreword
Web development is often seen as a crazy world where the way you develop software is by throwing hacks on top of hacks. I believe that React breaks from this pattern and instead has been designed from first principle which gives you a solid foundation to build on.
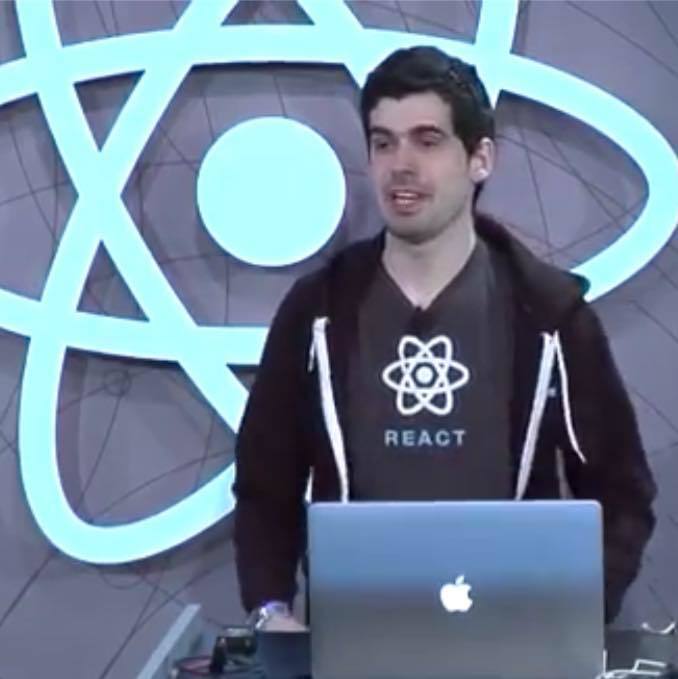
Christopher Chedeau - Front-end Engineer at Facebook
A major source of bugs for front-end applications was around synchronizing the data model with the DOM. It is very hard to make sure that whenever data changes, everything in the UI is updated with it.
Reacts first innovation was to introduce a pure-JavaScript representation of the DOM and implement diffing in userland and then use events which send simple commands: create, update, delete.
With React, by conceptually re-rendering everything whenever anything changes, not only do you have code that is safe by default, it is also less work as you only need to write the creation path: updates are taken care of for you.
Browsers have, for a long time, been incompatible in various ways due to the large API surface area of what they have to support to make the DOM work. Not only does React provide a great way to solve browser differences, but it enables use cases that were never before possible for a front-end library, such as server-side rendering and the ability to implement rendering targets like native iOS, Android, and even hardware components.
But the most important thing about React and the main reason why you should read this book: not only will you use it to make great applications for your users, it will also make you a better developer. Libraries come and go all the time and React is likely going to be no exception. What makes it special is that it teaches you concepts that can be reused throughout your entire career.
You will become better at JavaScript because React doesnt come with a templating system. Instead, React pushes you to use the full power of JavaScript to build your user interface.
You are going to practice using parts of functional programming with map
and filter
and also encouraged to use the latest features of JavaScript (including ES6). By not abstracting away data management, React will force you to think about how to architect your app and encourage you to consider concepts like immutability.
Im very proud that the community built around React is not afraid of rethinking best practices. The community challenges the status quo in many areas. My advice to you is to read this excellent book to learn and understand the fundamentals of React. Learning new concepts may feel strange but give it 5 minutes and practice them until you feel comfortable.
Then, try to break the rules. There is no one best way to build software and React is no exception. React actually embraces this fact by providing you with escape hatches when you want to do things outside of the React-way.
Come up with crazy ideas and who knows, maybe you are going to invent the successor to React!
Christopher Chedeau@vjeuxFront-end Engineer at Facebook
How to Get the Most Out of This Book
Overview
This book aims to be the single most useful resource on learning React. By the time youre done reading this book, you (and your team) will have everything you need to build reliable, powerful React apps.
React core is lean and powerful. After the first few chapters, youll have a solid understanding of Reacts fundamentals and will be able to build a wide array of rich, interactive web apps with the framework.
But beyond Reacts core, there are many tools in its ecosystem that you might find helpful for building production apps. Things like client-side routing between pages, managing complex state, and heavy API interaction at scale.
This book consists of two parts.
In Part I, we cover all the fundamentals with a progressive, example-driven approach. Youll create your first apps, learn how to write components, start handling user interaction, manage rich forms, and even interact with servers.
We bookend the first part by exploring the inner workings of Create React App (Facebooks tool for running React apps), writing automated unit tests, and building a multi-page app that uses client-side routing.
Part II of this book moves into more advanced concepts that youll see used in large, production applications. These concepts explore strategies for data architecture, transport, and management:
Redux is a state management paradigm based on Facebooks Flux architecture. Redux provides a structure for large state trees and allows you to decouple user interaction in your app from state changes.
GraphQL is a powerful, typed, REST API alternative where the client describes the data it needs. We also cover how to write your own GraphQL servers for your own data.
Relay is the glue between GraphQL and React. Relay is a data-fetching library that makes it easy to write flexible, performant apps without a lot of data-fetching code.
Finally, in the last chapter, well talk about how to write native, cross-platform mobile apps using React Native.
There are a few guidelines we want to give you in order to get the most out of this book.
First, know that you do not need to read this book linearly from cover-to-cover. However, weve ordered the contents of the book in a way we feel fits the order you should learn the concepts. We encourage you to learn all the concepts in Part I of the book first before diving into concepts in Part II.