McLaughlin - What is Node.js?
Here you can read online McLaughlin - What is Node.js? full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2011, publisher: OReilly Media, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
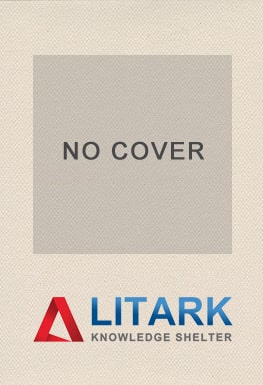
- Book:What is Node.js?
- Author:
- Publisher:OReilly Media
- Genre:
- Year:2011
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
What is Node.js?: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "What is Node.js?" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Node.js. Its the latest in a long line of Are you cool enough to use me? programmingnlanguages, APIs, and toolkits. In that sense, it lands squarely in the tradition of Rails,nand Ajax, and Hadoop, and even to some degree iPhone programming and HTML5. Get up to speed on Node with this concise overview.
What is Node.js? — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "What is Node.js?" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Copyright 2011 O'Reilly Media, Inc.
Learning Node might take a little effort, but its going to pay off. Why? Because youre afforded solutions to your web application problems that require only JavaScript to solve.
By Brett McLaughlin
Sections
Node.js. Its the latest in a long line of Are you cool enough to use me? programming languages, APIs, and toolkits. In that sense, it lands squarely in the tradition of Rails, and Ajax, and Hadoop, and even to some degree iPhone programming and HTML5. Go to a big technical conference, and youll almost certainly find a few talks on Node.js, although most will fly far over the head of the common mortal programmer.
Dig a little deeper, and youll hear that Node.js (or, as its more briefly called by many, simply Node) is a server-side solution for JavaScript, and in particular, for receiving and responding to HTTP requests. If that doesnt completely boggle your mind, by the time the conversation heats up with discussion of ports, sockets, and threads, youll tend to glaze over. Is this really JavaScript? In fact, why in the world would anyone want to run JavaScript outside of a browser, let alone the server?
The good news is that youre hearing (and thinking) about the right things. Node really is concerned with network programming and server-side request/response processing. The bad news is that like Rails, Ajax, and Hadoop before it, theres precious little clear information available. There will be, in time as there now is for these other cool frameworks that have matured but why wait for a book or tutorial when you might be able to use Node today, and dramatically improve the maintainability of your code and even the ease with which you bring on programmers?
Node is like most technologies that are new to the masses, but old hat to the experienced few: its opaque and weird to most but completely usable for a small group. The result is that if youve never worked with Node, youre going to need to start with some pretty basic server-side scripts. Take your time making sure you know whats going on, because while this is JavaScript, its not operating like the client-side JavaScript youre used to. In fact, youre going to have to twist your JavaScript brain around event loops and waiting and even a bit of network theory.
Unfortunately, this means that if youve been working and playing with Node for a year or two, much of this article is going to seem pedestrian and overly simplistic. Youll look for things like using Node on the client, or heavy theory discussions on evented I/O and reactor patterns, and npm
. The reality is that while thats all interesting and advances Node to some pretty epic status its incomprehensible to someone just getting started out. Given that, maybe you should pass this piece on to your co-workers who dont know Node, and then when theyre buying into Nodes usefulness, start to bring them along on the more advanced Node use cases.
First things first: you need to realize that Node is intended to be used for running standalone JavaScript programs. This isnt a file referenced by a piece of HTML and running in a browser. Its a file sitting on a file system, executed by the Node
program, running as what amounts to a daemon, listening on a particular port.
The classic example here is Hello World, detailed on the Node website. Almost everyone starts with Hello World, though, so check that out on your own, and skip straight to something a lot more interesting: a server that can send static files, not just a single line of text:
var sys = require("sys"), http = require("http"), url = require("url"), path = require("path"), fs = require("fs");http.createServer(function(request, response) { var uri = url.parse(request.url).pathname; var filename = path.join(process.cwd(), uri); path.exists(filename, function(exists) { if(!exists) { response.writeHead(404, {"Content-Type": "text/plain"}); response.end("404 Not Found\n"); return; } fs.readFile(filename, "binary", function(err, file) { if(err) { response.writeHead(500, {"Content-Type": "text/plain"}); response.end(err + "\n"); return; } response.writeHead(200); response.end(file, "binary"); }); });}).listen(8080);console.log("Server running at http://localhost:8080/");Thanks much to Mike Amundsen for the pointer to similar code. This particular example was posted by Devon Govett on the Nettuts+ training blog, although its been updated for the current version of Node in a number of places. Devons entire tutorial post is actually a great companion piece on getting up to speed on Node once you have a handle on the basics.
If youre new to Node, type this code into a text file and save the file as NodeFileServer.js . Then head out to the Node website and download Node or check it out from the git repository. Youll need to build the code from source; if youre new to Unix, make
, and configure
, then check out the online build instructions for help.
Dont worry that youve put aside NodeFileServer.js for a moment; youll come back to it and more JavaScript shortly. For now, soak in the realization that youve just run through the classic Unix configuration and build process:
./configuremakemake installThat should come with another realization: Node itself isnt JavaScript. Node is a program for running JavaScript, but isnt JavaScript itself. In fact, Node is a C program. Do a directory listing on the Node/src directory and youll see something like this:
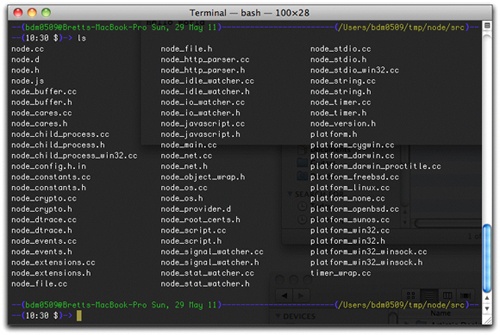
For all of you thinking that JavaScript is a poor language in which to be writing server-side tools, youre half right. Yes, JavaScript is not equipped to deal with operating system-level sockets and network connectivity. But Node isnt written in JavaScript; its written in C, a language perfectly capable of doing the grunt work and heavy lifting required for networking. JavaScript is perfectly capable of sending instructions to a C program that can be carried out in the dungeons of your OS. In fact, JavaScript is far more accessible than C to most programmers something worth noting now, and that will come up again and again in the reasons for looking seriously at Node.
The primary usage of Node further reflects that while Node works with JavaScript, it isnt itself JavaScript. You run it from the command line:
(bdm0509@Bretts-MacBook-Pro Sun, 29 May 11) (/Users/bdm0509/tmp/Node/src) (09:09 $)-> export PATH=$HOME/local/Node/bin:$PATH (bdm0509@Bretts-MacBook-Pro Sun, 29 May 11) (/Users/bdm0509/tmp/Node/src) (09:09 $)-> cd ~/examples (bdm0509@Bretts-MacBook-Pro Sun, 29 May 11) (/Users/bdm0509/examples) (09:09 $)-> Node NodeFileServer.jsServer running at http://127.0.0.1:1337/And there you have it. While theres a lot more to be said about that status line and whats really going on at port 1337 the big news here is that Node is a program that you feed JavaScript. What Node then does with that JavaScript isnt worth much ink; to some degree, just accept that what it does, it
Font size:
Interval:
Bookmark:
Similar books «What is Node.js?»
Look at similar books to What is Node.js?. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book What is Node.js? and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.