C# Design Pattern Essentials
by Tony Bevis
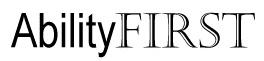
Copyright Tony Bevis, 2012
The right of Tony Bevis to be identified as the author of this work has been asserted by him in accordance with the Copyright, Design and Patents Act, 1988.
All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form, or by any means, electronic, mechanical, photocopying, recording, or otherwise, without the prior written consent of the publisher.
Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in this book, and we were aware of a trademark claim, the designations have been printed with initial capital letters or in all capital letters. C# and all C# related trademarks and logos are trademarks or registered trademarks of Microsoft Corporation in the United States and other countries. The publisher is independent of Microsoft.
The publisher and author have taken care in the preparation of this book, but make no expressed or implied warranty of any kind and assume no responsibility for errors or omissions. No liability is assumed for incidental or consequential damages in connection with or arising out of the use of the information or programs contained herein. The software code samples included within this book are for illustrative and educational purposes only, and are not intended for use in any actual software application or system.
Published by:
Ability First Limited, Dragon Enterprise Centre, 28 Stephenson Road, Leigh-on-Sea, Essex SS9 5LY, United Kingdom
www.abilityfirst.co.uk/books
Published: November 2012
ISBN: 978-0-9565758-7-6 (e-book version)
This book has been adapted from the paperback version (ISBN: 978-0-9565758-6-9)
Cover image by Ivan Polushkin, copyright Fotolia.
This book is dedicated to The Gang of Four.
Preface
This book is an introductory guide to the world of object-oriented software design patterns. The examples and code extracts have been deliberately kept simple, allowing you to concentrate on understanding the concepts and application of each pattern rather than having to wade through irrelevant source code.
The book assumes that you have at least a basic knowledge of the C# programming language, including understanding what is meant by the terms encapsulation, inheritance and polymorphism, and that you know how to write classes and interfaces. By the end of this book you should be able to apply that knowledge to the design of complex applications, where objects from multiple classes need to interact to accomplish particular goals.
The patterns described within comprise all 23 of the patterns in the seminal work of Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides; Design Patterns: Elements of Reusable Object-Oriented Software (Addison-Wesley, 1995). There are also four additional patterns described including Model-View-Controller (MVC), now a mainstay of graphical applications. For the most part, each chapter is self-contained, and you can therefore dip into the patterns in any order. However, it is recommended that you read Chapter 1 What are Design Patterns? first to familiarise yourself with the common theme and the object-oriented principles that the patterns utilise.
This book also makes use of a simplified implementation of Unified Modeling Language (UML) diagrams to show how the classes that comprise a pattern are structured. If you are unfamiliar with UML diagrams then you may wish to refer to Appendix A before you begin.
Prerequisite knowledge
In order to make use of this book you should have a basic understanding of both the C# language and of object-oriented principles. In particular, you should know how to create classes, interfaces and enums, and understand the terms encapsulation, inheritance, composition and polymorphism.
How this book is organised
Part I introduces the idea of design patterns, and lays the foundation for some simple core classes that comprise the common theme used throughout this book.
Part II describes the five creational patterns, that is, those that help manage the instantiation of objects.
Part III describes the seven structural patterns, that is, those that help manage how classes are organised and interrelate.
Part IV describes the eleven behavioural patterns, that is, those that help manage what the classes actually do.
Part V describes four additional patterns you should find useful in practical applications.
Part VI contains a single chapter that develops a simple 3-tier application that uses some of the more commonly used design patterns.
Part VII contains the appendixes, which includes a brief explanation of the Unified Modeling Language (UML) diagram formats for those unfamiliar with UML, and a quick reference for each of the 23 main patterns.
Conventions used in this book
C# code that you need to enter, or results that are shown as output, is shown in a fized-width font as follows:
anObject.DoSomething();
anotherObject.DoThis();
Often, a piece of additional or modified code is provided, and the parts that are new or changed are indicated in bold:
anObject.D oSomethingElseInstead() ;
anObject.AlsoDoThis();
anotherObject.doThis();
Names of classes, objects or C# statements will appear in the text using a fixed-width font such as MyClass or someObject , for example.
For reasons of brevity, using and namespace statements are omitted from most of the code samples in this book.
The books resources
You can also download all of the C# source code from this book from our website:
http://www.abilityfirst.co.uk/books
Note to readers
This book is an adaptation of Java Design Pattern Essentials - Second Edition by the same author. It is possible that some of the text and code samples may reflect this adaptation in terms of the style and terminology differences between the languages. Readers' feedback is more than welcome.
Part I. Introduction
This part introduces the idea of design patterns, and lays the foundation for some simple core classes that comprise the common theme used throughout this book.
1. What are Design Patterns?
Imagine that you have just been assigned the task of designing a software system. Your customer tells you that he needs to model the Gadgets his factory makes and that each Gadget comprises the same component parts but those parts are a bit different for each type of Gadget. And he also makes Gizmos, where each Gizmo comes with a selection of optional extras any combination of which can be chosen. And he also needs a unique sequential serial number stamped on each item made.
Just how would you go about designing these classes?
The chances are that no matter what problem domain you are working in, somebody else has had to design a similar solution in the past. Not necessarily for Gadgets and Gizmos of course, but conceptually similar in terms of objectives and structure. In other words there's a good chance that a generic solution or approach already exists, and all you need to do is to apply that approach to solve your design conundrum.
This is what Design Patterns are for. They describe generic solutions to software design problems. Once versed in patterns, you might think to yourself "those Gadgets could be modelled using the Abstract Factory pattern, the Gizmos using the Decorator pattern, and the serial number generation using the Singleton pattern."
How this book uses patterns
This book gives worked examples for each of the 23 patterns described in the classic reference work Design Patterns: Elements of Reusable Object-Oriented Software (Gamma, 1995) plus four additional useful patterns, including Model-View-Controller (MVC).
Next page