Copyright
Python Programming by Example
Agus Kurniawan
1st Edition, 2015
Copyright 2015 Agus Kurniawan
* Cover photo is credit to Fajar Ramadhany, Bataviasoft, http://bataviasoft.com/.
Table of Contents
Preface
This book is a brief reference to the Python programming language. It describes all the elements of the language and illustrates their use with code examples.
Agus Kurniawan
Depok, November 2015
1. Development Environment
1.1 Installation
Python is a widely used general-purpose, high-level programming language. Installation of Python application is easy. For Windows, Linux and Mac Platform, you download setup file from Python website, https://www.python.org/downloads/. Download and run it. Follow installation commands.
If you're working on Windows platform, you can run setup file and follow instruction.
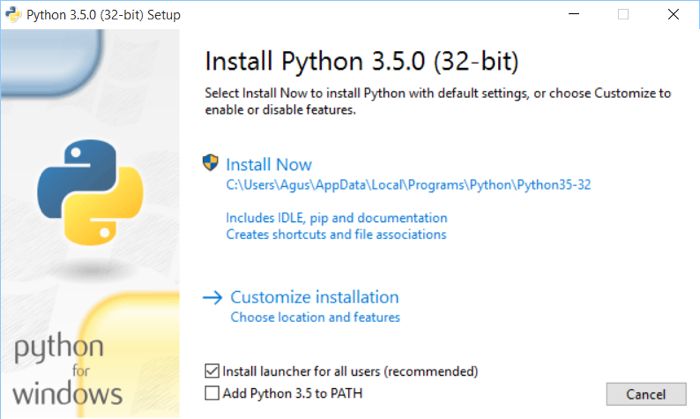
1.2 Development Tools
Basically, you can use any text editor to write Python code. The following is a list of text editor:
- vim
- nano
- PyCharm, https://www.jetbrains.com/pycharm/
- Intellij IDEA, https://www.jetbrains.com/idea/
- Sublime text, http://www.sublimetext.com/
- Visual Studio, https://www.visualstudio.com
In this book, I use PyCharm for development tool. Jetbrains provides community and Education licenses for PyCharm.
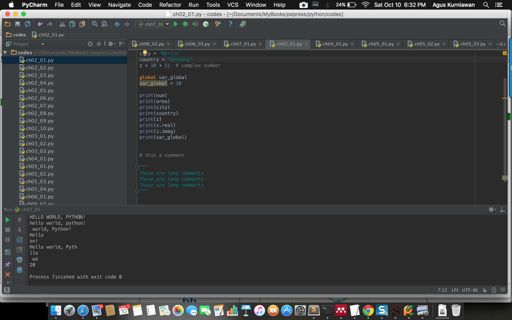
1.3 Python Shell
After installed Python, you obtain Python shell on your platform. You can type this command on Terminal or Command Prompt for Windows Platform.
python
This is Python 2.x. Then, you get Python shell, shown in Figure below.
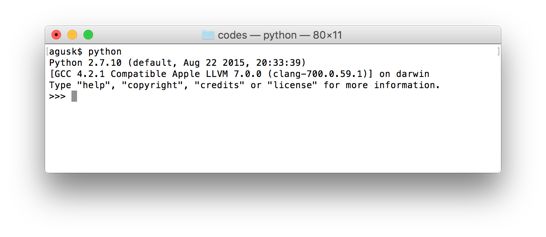
If you installed Python 3.x, you can Python shell by typing this command.
python3
This is Python 3.x. Then, you get Python shell, shown in Figure below.
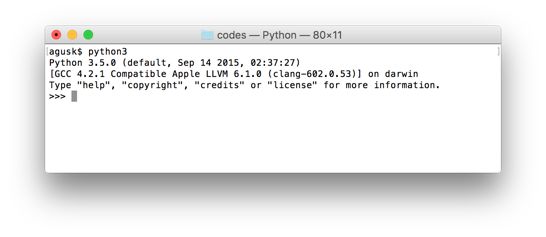
Output program on Windows platform.
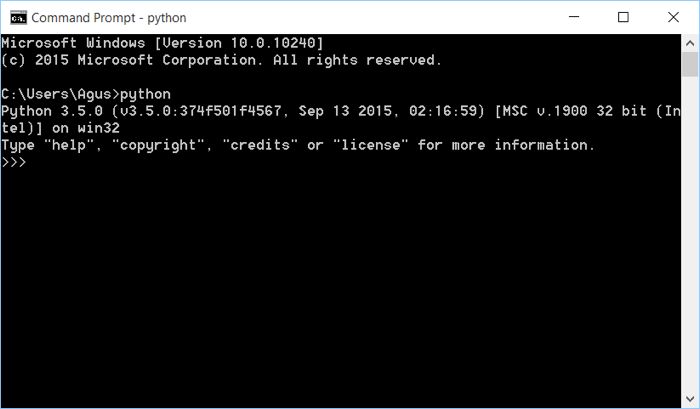
After you call Python shell, you obtain the shell. It shows >>> on Terminal.
Try to do the following command.
>>> a = 3>>> b = 5>>> print a>>> c = a * b>>> print c
In Python 3.x, print a is replaced by print(a).
The following is a sample output of program.
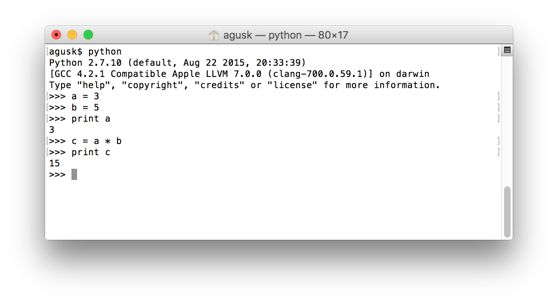
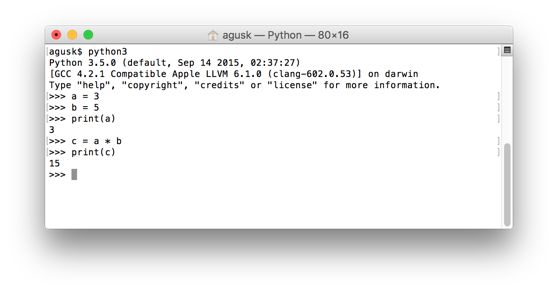
A sample output for Windows platform.
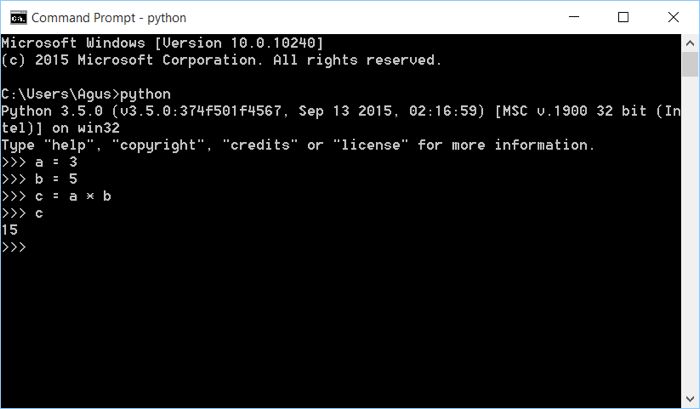
1.4 Running Python Application from Files
You can run your program by writing them on a file. For instance, you create a file, called ch01_01.py, and write this script.
print ( 'hello world from python' )
To run the program, you can type this command on Terminal.
python ch01_01.py
If you want to run the program under Python 3.x, type this command on Terminal.
python3 ch01_01.py
Program output:
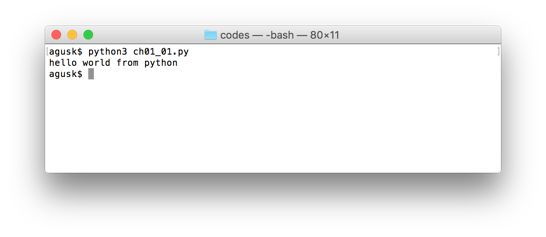
2. Python Programming Language
This chapter explains the basic of Python programming language.
2.1 Common Rule
Python language doesn't write ";" at the end of syntax like you do it on C/C++ languages. Here is the syntax rule of Python:
syntax_code1syntax_code2syntax_code3
2.2 Variables
In this section, we explore how to define a variable and assign its value. By default, we define variables on Python with assigning value .
# declare variables num = area = 58.7 city = 'Berlin' country = "Germany" z = + j # complex number
If you want to declare variables without assigning values, you can set it using None.
# declare variable without initializing value counter = None index = None
Write these codes for testing.
# declare variables num = area = 58.7 city = 'Berlin' country = "Germany" z = + j # complex number # declare variable without initializing value counter = None index = None global var_globalvar_global = print (num) print (area) print (city) print (country) print (z) print (z . real) print (z . imag) print (var_global)
Save these scripts into a file, called ch02_01.py.
Now you can type this file using Python 3.x.
$ python3 ch02_01.py
A sample of program output can seen in Figure below.
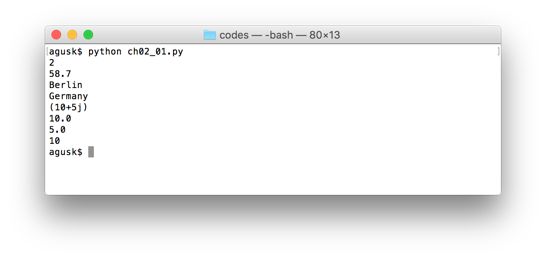
2.3 Comment
You may explain how to work on your code with writing comments. To do it, you can use # and """ syntax. Here is sample code:
# this a comment """ These are long comments These are long comments These are long comments """
2.4 Arithmetic Operations
Python supports the same four basic arithmetic operations such as addition, subtraction, multiplication, and division. For testing, create a file, called ch02_02.py.
The following is the code illustration for basic arithmetic in ch02_02.py:
a = 2.3 b = c = a + b print (c)c = a - b print (c)c = a * b print (c)c = a / b print (c)
Save and run this program.
python3 ch02_02.py
A sample of program output:
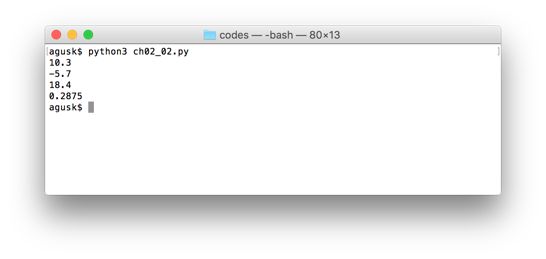
2.5 Mathematical Functions
Python provides math library. If youre working with Python 2.x, you can read this library on https://docs.python.org/2/library/math.html . For Python 3.x, you can read math library on https://docs.python.org/3/library/math.html .
Create a file, called ch02_03.py. Write the following code.
from math import * a = 1.8 b = 2.5 c = pow (a, b) print (c)c = sqrt(b) print (c)c = sin(a) print (c) print (pi)
Save and run the program.
python3 ch02_03.py
A sample of program output:
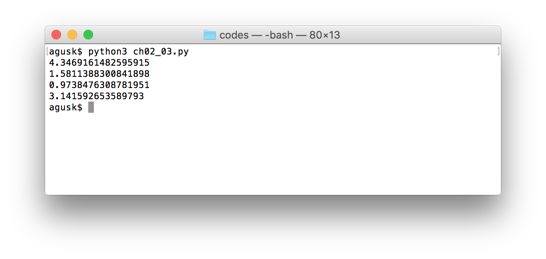
2.6 Increment and Decrement
Python doesn't has special syntax for increment and decrement. We can define increment and decrement as follows.
- ++ syntax for increment. a++ can be defined as a = a + 1
- -- syntax for decrement. a-- can be defined as a = a - 1
For testing, create a file, called