Ian Ozsvald - High Performance Python
Here you can read online Ian Ozsvald - High Performance Python full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2018, publisher: OReilly Media, Inc., genre: Home and family. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
- Book:High Performance Python
- Author:
- Publisher:OReilly Media, Inc.
- Genre:
- Year:2018
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
High Performance Python: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "High Performance Python" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
High Performance Python — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "High Performance Python" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
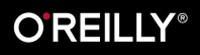
Beijing Cambridge Farnham Kln Sebastopol Tokyo
Python is easy to learn. Youre probably here because now that your code runscorrectly, you need it to run faster. You like the fact that your code is easyto modify and you can iterate with ideas quickly. The trade-off between easy todevelop and runs as quickly as I need is a well understood and bemoanedphenomenon. There are solutions.
Some people have a serial process that has to run faster. Others have problemsthat could take advantage of multi-core architectures, clusters or GPUs. Someneed scalable systems that can process more or less as expediency and fundsallow, without losing reliability. Others will realise that their codingtechniques, often borrowed from other languages, perhaps arent as natural asexamples they see from others.
In this book we will cover the above topics, giving practical guidance tounderstanding bottlenecks and then producing faster and more scalable solutions.We also include some war stories from those who went ahead of you, who took theknocks so you dont have to.
Python is well-suited for rapid development, production deployments and scalablesystems. The ecosystem is full of people who are working to make it scale onyour behalf, leaving you more time to focus on the more challenging tasks aroundyou.
Youve used Python for long enough to have an idea about why certain things areslow and to have seen technologies like Cython, numpy and PyPy being discussedas possible solutions. You might also have programmed with other languages andso know that theres more than one way to solve a performance problem.
While this book is primarily aimed at people with a CPU-bound problem, we alsolook at data transfer and memory bound solutions. Typically these problems arefaced by scientists, engineers, quants and academics.
We also look at problems that a web developer might face including the movementof data and the use of Just in Time compilers like PyPy for easy-win performancegains.
It might help if you have a background in C (or C++ or maybe Java), but it isnta pre-requisite. Pythons most common interpreter (CPython - the standard younormally get if you type python
at the command line) is written in C and sothe hooks and libraries all expose the gory inner C machinery. There are lots ofother techniques that we cover that dont assume any knowledge of C.
You might also have a lower level knowledge of the CPU, memory architecture anddata buses but again thats not strictly necessary.
This book is meant for intermediate to advanced Python programmers. Motivatednovice Python programmers may be able to follow along as well, but we recommendhaving a solid Python foundation.
We dont cover storage-system optimization, if you have a SQL or NoSQLbottleneck then this book probably wont help you.
Your authors have been working with large volumes of data, a requirement for Iwant the answers faster! and a need for scalable architectures for many yearsin both industry and academia. Well try to impart our hard-won experience tosave you from making the mistakes that weve made.
At the start of each chapter well list questions that the following text shouldanswer (and if it doesnt - tell us and well fix that for the next revision!).
We cover the following:
- A background on the machinery of a computer so you know whats happening behind the scenes
- Profiling - learn to figure out what is slow or eating all your RAM so you know where to focus your efforts
- Pure Python approaches - use Python and its modules effectively
- Matrices with numpy - use the beloved
numpy
library like a beast - Compilation and Just In Time computing - processing faster by compiling down to machine code, make sure youre guided by the results of profiling
- Concurrency - ways to move data efficiently
- multiprocessing - learn the various ways to use the built-in
multiprocessing
library for parallel computing, efficiently sharenumpy
matrices and learn about inter-process communication (IPC) - Cluster computing - convert your
multiprocessing
code to run on a local or remote cluster for both research and production systems - Using less RAM - approaches to solving large problems without buying a humungous computer
- Lessons from the Field - lessons encoded in war stories from those who took the blows so you dont have to
Python 2.7 is the dominant version of Python for scientific and engineeringcomputing. 64-bit is dominant in this field along with *nix environments (oftenLinux or Mac). 64-bit lets you address larger amounts of RAM. *nix lets youbuild applications that can be deployed and configured in well-understood wayswith well-understood behaviors.
If youre a Windows user then youll have to buckle-up. Most of what we showwill work just fine, some things are OS specific and youll have to research aWindows solution. The biggest difficulty a Windows user might face is theinstallation of modules, research in sites like StackOverflow should give youthe solutions you need. If youre on Windows then having a virtual machine (e.g.using VirtualBox) with a running Linux installation might help you to experimentmore freely.
Windows users should definitely look at a packaged solution like those available through Anaconda, Canopy, Python(x,y), or Sage. These same distributions will make the lives of Linux and Mac users far simpler too.
Python 3 is the future of Python and everyone is moving towards it. Python 2.7 will nonetheless be around for manyyears to come (some installations still use Python 2.4 from 2004), the retirement date for Python 2.7 has been set to 2020.
The shift toPython 3.3+ has caused enough headaches for library developers that people havebeen slow to port their code (with good reason), therefore people have been slowto adopt Python 3. This is mainly due to the complexities of switching from a mix of string and unicode datatypes in complicated applications to the unicode and byte implementation in Python 3.
Typically when you want reproducible results based on a set of trustedlibraries, you dont want to be at the bleeding edge. High performance Pythondevelopers are likely to be using and trusting Python 2.7 for years to come.
Most of the code in this book will run with little alteration for Python 3.3+(the most significant change will be with print
turning from a statement intoa function). In a few places we specifically look at improvements that Python3.3+ provide. One item that might catch you out is the fact that /
means integer division in Python 2.7 but it becomes float division in Python 3. Of course - being a good developer your well-constructed unit test suite will already be testing your important code paths and so youll be alerted by your unit tests if this needs to be addressed in your code.
scipy
and numpy
have been Python 3 compatible since late in 2010, matplotlib
was compatible from 2012, scikit-learn
was compatible in 2013 and NLTK
is expected to be compatible in 2014. Django has been compatible since 2013. Their transition notes are available in their repositories and newsgroups, it is worth reviewing the processes they used if youre going to migrate older code to Python 3.
Font size:
Interval:
Bookmark:
Similar books «High Performance Python»
Look at similar books to High Performance Python. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book High Performance Python and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.