Moulton - iOS Apps with REST APIs
Here you can read online Moulton - iOS Apps with REST APIs full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2015, publisher: leanpub.com, genre: Home and family. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
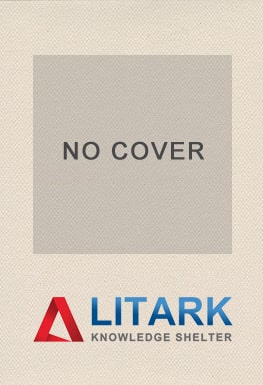
- Book:iOS Apps with REST APIs
- Author:
- Publisher:leanpub.com
- Genre:
- Year:2015
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
iOS Apps with REST APIs: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "iOS Apps with REST APIs" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Moulton: author's other books
Who wrote iOS Apps with REST APIs? Find out the surname, the name of the author of the book and a list of all author's works by series.
iOS Apps with REST APIs — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "iOS Apps with REST APIs" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
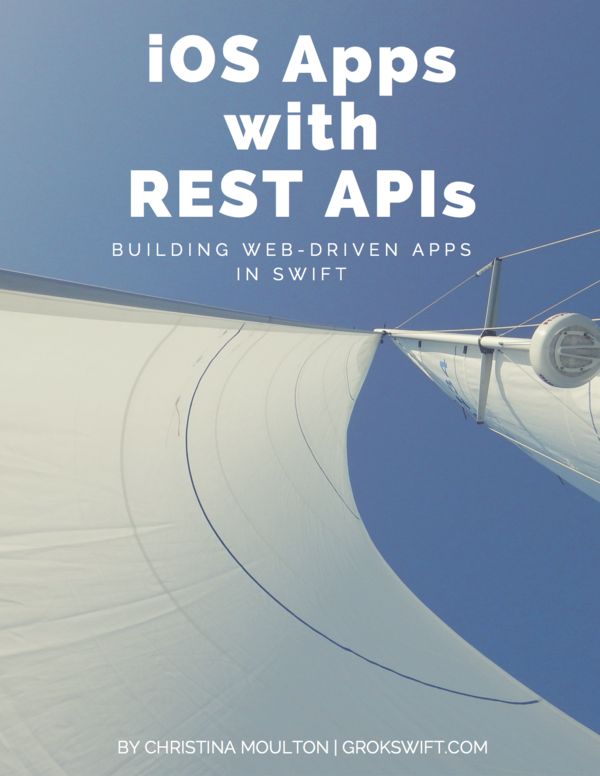
This book is for sale at http://leanpub.com/iosappswithrest
This version was published on 2016-05-07
* * * * *
This is a Leanpub book. Leanpub empowers authors and publishers with the Lean Publishing process. Lean Publishing is the act of publishing an in-progress ebook using lightweight tools and many iterations to get reader feedback, pivot until you have the right book and build traction once you do.
* * * * *
Without a few key people this book wouldnt have happened. Most of all, thanks to Jeff Moulton for putting up with my excessive focus on coding & writing, even while living on a 34 sailboat. Jeff also took the cover photo.
Thanks also to:
- My Twitter peeps for support & favs
- @BugKrusha & the iOS Developers community
- GitHub
- LeanPub
- Everyone who gave feedback or asked questions about the book or GrokSwift.com. Every little bit helps make the book better for you.
This book and the code in it have been improved by the review and recommendations of Michael Langford acting as Technical Editor.
You need to build an iOS app around your teams API or integrate a third party API. You need a quick, clear guide to demystify Xcode and Swift. No esoteric details about Core Anything or mathematical analysis of flatMap
. Only the nitty gritty that you need to get real work done now: pulling data from your web services into an iOS app, without tossing your MacBook or Mac Mini through a window.
You just need the bare facts on how to get CRUD done on iOS. Thats what this book will do for you.
After reading this book youll be able to:
- Analyze a JSON response from a web service call and write Swift code to parse it into model objects
- Display those model objects in a table view so that when the user launches the app they have a nice list to scroll through
- Add authentication to use web service calls that require OAuth 2.0, a username/password, or a token
- Transition from the main table view to a detail view for each object, possibly making another web service call to get more info about the object
- Let users add, modify and delete objects (as long as your web service supports it)
- Hook in to more web service calls to extend you app, like adding user profiles or letting users submit comments or attach photos to objects
To achieve those goals well build out an app based on the GitHub API, focusing on gists. (If youre not familiar with gists, theyre basically just text snippets, often code written a GitHub user.) Your model objects might be bus routes, customers, chat messages, or whatever kind of object is core to your app. Well start by figuring out how to make API calls in Swift then well start building out our app one feature at a time:
- Show a list of all public gists in a table view
- Load more results when the user scrolls down
- Let them pull to refresh to get the latest public gists
- Load images from URLs into table view cells
- Use OAuth 2.0 for authentication to get lists of private and starred gists
- Have a detail view for each gist showing the text
- Allow users to add new gists, star and unstar gists, and delete gists
- Handle not having an internet connection with warnings to the user and saving the gists on the device
- Software developers getting started with iOS but experienced in other languages
- Front-end devs looking to implement native UIs for iOS apps (no CSS, oh noes!)
- Back-end devs tasked with getting the data into the users hands on iOS
- Android, Windows Phone, Blackberry, Tizen, Symbian & Palm OS devs looking to expand their web service backed apps to iOS
- Anyone whose boss is standing over their shoulder asking why the API data isnt showing up in the table view yet
- Complete newcomers to programming, you should have a decent grasp of at least one object-oriented programming language or have completed several intro to iOS tutorials
- Designers, managers, UX pros, Its a programming book. All the monospace font inserts will probably drive you crazy.
- Cross-platform developers dedicated to their tools (including HTML5 & Xamarin), this is all Swift & native UI, all the time
- Programmers building apps that have little or no web service interaction
- Game devs, unless youre tying in a REST-like API
This book is mostly written as a tutorial in implementing the gists app. Depending on how you learn best and how urgently you need to implement your own app, there are two different approaches you might take:
- Work through the tutorials as written, creating an app for GitHub Gists. Youll understand how that app works and later be able to apply it to your own apps.
- Read through the tutorials but implement them for your own app and API. Throughout the text Ill point out where youll need to analyze your own requirements and API to help you figure out how to modify the example code to work with your API. Those tips will look like this:
List the tasks or user stories for your app. Compare them to the list for the gists app, focusing on the number of different objects (like stars, users, and gists) and the types of action taken (like viewing a list, viewing an objects details, adding, deleting, etc.).
Well start with that task in the next chapter. Well analyze our requirements and figure out just what were going to build. Then well start building the gists app, right after an introduction to making network calls and parsing JSON in Swift.
Like anything in tech there are plenty of buzzwords around web services. For a while it was really trendy to say your web services were RESTful. If you want to read the theory behind it, head over to Wikipedia. For our purposes in this book, all we mean by REST web service or even when we say web service or API is that we can send an HTTP request and we get back some data in a format thats easy to use in our app. Usually the response will be in JSON.
Web services are wonderful since they let you use existing systems in your own apps. Theres always a bit of a learning curve with any web service that youre using for the first time since every one has its own quirks. Most of the integration is similar enough that we can generalize how to integrate them into our iOS apps.
If you want an argument about whether or not a web service is really RESTful youre not going to find it here. Weve got work that just needs to get done.
In this book were going to deal with web services that return JSON. JSON is hugely common these days so its probably what youll be dealing with. Of course, there are other return types out there, like XML. This book wont cover responses in anything but JSON but it will encapsulate the JSON parsing so that you can replace it with whatever you need to without having to touch a ton of code. If you are dealing with XML response you should look at NSXMLParser.
Font size:
Interval:
Bookmark:
Similar books «iOS Apps with REST APIs»
Look at similar books to iOS Apps with REST APIs. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book iOS Apps with REST APIs and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.