Chapter 7: Integrating Bootstrap with React: a Guide for Developers
by Manjunath M
Integrating Bootstrap with React allows React developers to use Bootstraps state-of-the-art grid system and its various other components.
In this tutorial, were going to:
- explore tools and techniques for building a user interface with Bootstraps look and feel for a React-powered web application
- use reactstrap to build a React contact list application.
React is one of the most popular JavaScript technologies for interactive web applications. Its popularity derives from its component-based modular approach, composability and fast re-rendering algorithm.
However, being a view library, React doesnt have any built-in mechanism that can help us create designs that are responsive, sleek and intuitive. Thats where a front-end design framework like Bootstrap steps in.
Why You Cant Just Include Bootstrap Components with React
Combining Bootstrap with React isnt as easy as adding
to an
index.html
file. This is because Bootstrap depends on jQuery for powering certain UI components. jQuery uses a direct DOM manipulation approach thats contradictory to Reacts declarative approach.
If we need Bootstrap just for the responsive 12 column grid, or the components that dont use jQuery, we can simply resort to the vanilla Bootstrap stylesheet. Otherwise, there are many libraries that take care of bridging the gap between React and Bootstrap. Well compare both methods so that well be in a better position to choose the approach that works for our specific case.
Lets get started!
Setting up a Bootstrap Stylesheet with React
Lets use the Create React App CLI to create a React project, which requires zero configuration to get started.
We install Create React App and run the following command to start a new project and serve the development build:
$ create-react-app react-and-bootstrap$ cd react-and-bootstrap$ npm start
Heres the directory structure created by Create React App:
. package.json public favicon.ico index.html manifest.json README.md src App.css App.js App.test.js index.css index.js logo.svg registerServiceWorker.js yarn.lock
Next, lets download the latest version of the Bootstrap library from Bootstraps official site. The package includes both the compiled and minified versions of the CSS and JavaScript. Well just copy the CSS and place it inside the public/
directory. For projects that just need the grid, there is a grid-specific stylesheet included too.
Next, we create a new directory for CSS inside public/
, paste bootstrap.min.css
in our newly created css
directory, and link it from public/index.html
:
Alternatively, we can use a CDN to fetch the minified CSS:
Lets have a look at what works and what doesnt with this setup.
Using Vanilla Bootstrap with React
Once we add the Bootstrap stylesheet to a React project, we can use the regular Bootstrap classes inside JSX code. Lets head over to the Bootstrap demo site and copy over some random example code, just to check everything is working as it should:
Live Code
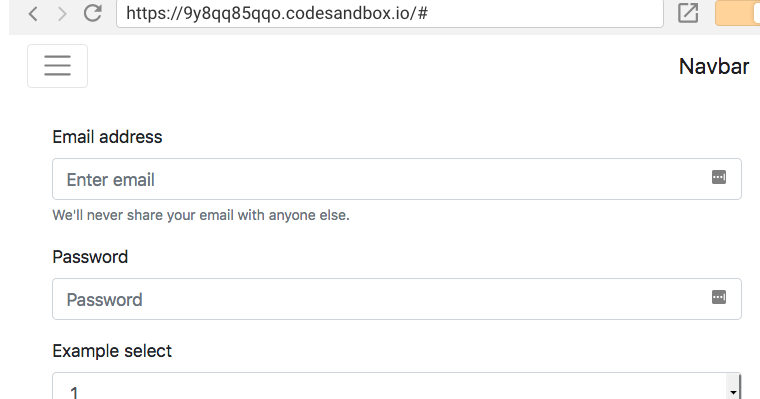
Check out the sample site here.
As we can see, the form looks good, but the NavBar doesnt. Thats because the NavBar depends on the Collapse jQuery plugin, which we havent imported. Apart from being unable to toggle navigation links, we cant use features like dropdown menus, closable alerts and modal windows, to name a few.
Wouldnt it be awesome if we could import Bootstrap as React components to make the most out of React? For instance, imagine if we could use a combination of Grid, Row and Column components to organize the page instead of the pesky HTML classes:
One of three columns
One of three columns
One of three columns
One of three columns One of three columns One of three columns
Fortunately, we dont have to implement our own library to serve this purpose, as there are some popular solutions already available. Lets take a look at some of them.
An Overview of Third-party Libraries for React and Bootstrap
There are a few libraries that try to create a React-specific implementation of Bootstrap so that we can use JSX components while working with Bootstrap styles. Ive compiled a list of a few popular Bootstrap modules that we can use with our React projects.
React-Bootstrap is by far one of the most popular libraries for adding Bootstrap components into React. However, at the time of writing this tutorial, it targets Bootstrap v3 and not the latest version of Bootstrap. Its in active development and the API might break when a newer version is released.
reactstrap is another library that gives us the ability to use Bootstrap components in React. Unlike React-Bootstrap, reactstrap is built for the latest version of Bootstrap. The module includes components for typography, icons, forms, buttons, tables, layout grids, and navigation. Its also in active development and its a nice alternative for building React-powered Bootstrap apps.
There are a few other libraries like React-UI, and domain-specific modules like React-bootstrap-table and CoreUI-React Admin Panel on GitHub, which provide extended support for creating awesome UIs using React.
The rest of this tutorial will focus on reactstrap, because its the only popular module that uses the latest version of Bootstrap.
Setting up the reactstrap Library
We start by installing the reactstrap library using npm:
npm install --save reactstrap@next
Now we can import the relevant components from the module as follows:
import { Container, Row, Col} from 'reactstrap';
However, the library wont work as we might expect it to yet. As explained on the reactstrap website, the reason for this is that reactstrap doesnt include Bootstrap CSS, so we need to add it ourselves:
npm install --save bootstrap
Next, we import Bootstrap CSS in the src/index.js
file:
import 'bootstrap/dist/css/bootstrap.css';
Bootstrap Grid Basics
Bootstrap has a responsive, mobile first and fluid grid system that allows up to 12 columns per page. To use the grid, well need to import the Container, Row and Col components.
The Container accepts a fluid
property that converts a fixed-width layout into a full-width layout. Essentially, it adds the corresponding .container-fluid
Bootstrap class to the grid.
As for
s, we can configure them to accept props such as
xs
,
md
,
sm
and
lg
that are equivalent to Bootstraps
col-*
classes for exmple,
Alternatively, we can pass an object to the props with optional properties like size
, order
and offset
. The size
property describes the number of columns, whereas order
lets us order the columns and accepts a value from 1 to 12. The offset
property moves the columns to the right.
The code below demonstrates some of the Grid features in reactstrap:
Live Code
Grid features in Reactstrap.
Bootstrap Style Components in React
Now that we have reactstrap at our fingertips, there are tons of Bootstrap 4 components that we can use with React. Covering them all is beyond the scope of this tutorial, but lets try a few important components and use them in an actual project say, a contact list app.