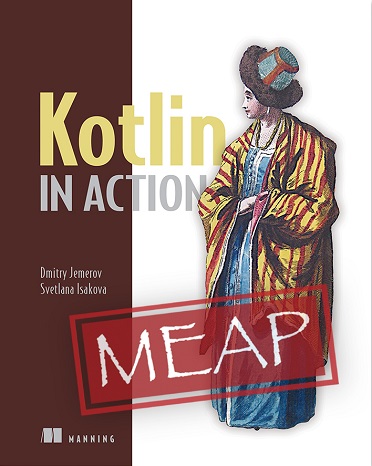
MEAP VERSION 11

ABOUT THIS MEAP
This book file has been tested with iBooks 1.5 on iOS 5, and the Kindle App for iPad 2.9. If you are using an earlier version of iBooks and are experiencing problems, please try upgrading iBooks.
To get a full-screen view of images:
- iBooks: Double-tap an image.
- Kindle app: Use two fingers to stretch the image.
You can download the most up-to-date version of your electronic books from your Manning Account at .
Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders.
https://forums.manning.com/forums/kotlin-in-action
WELCOME
Thanks for purchasing the MEAP for Kotlin in Action ! We're looking forward to introducing you to Kotlin, which is a new programming language that is a pragmatic, safe, concise, and interoperable alternative to Java. With Kotlin, you can implement your projects with less code, a higher level of abstraction, and fewer annoyances.
We assume that you're already familiar with Java, either as a server-side developer or as an Android application developer. Switching from Java to Kotlin is a smooth process that does not involve a steep learning curve, and there are many tools that can help. We expect that it won't take you long to become productive with the language.
One of the strong points of Kotlin is its strong interoperability with Java. If you want to try Kotlin for yourself, you can do this in a new project, but you also have an option of introducing it into your existing Java codebase. You can also easily try features of the language in an interactive console or an online playground.
The book consists of three parts. The first part will teach you the basic syntax of the language. The second part will focus on features that allow you to build reusable abstractions, higher-level functions, libraries, and even entire domain specific languages. Finally, the third part will focus on details of applying Kotlin in real-world projects, such as build system integration, Android support and concurrent programming.
Both of us have been part of the team working on Kotlin for the past five years, so you can be confident that you're receiving authoritative information straight from the source. And of course, as Kotlin evolves towards 1.0 and beyond that, we'll ensure that the information in the MEAP book always corresponds to the current state of the language.
We're looking forward to your feedback on the text that we have so far. If anything is confusing, unclear or seems to be missing entirely, please don't hesitate to leave your comments in the Author Online forum .
Dmitry Jemerov and Svetlana Isakova

BRIEF TABLE OF CONTENTS
Part 1: Introduction
Part 2: Embracing Kotlin
Appendixes:
A
B
C
KOTLIN: WHAT AND WHY
This chapter covers:
A basic demonstration of Kotlin
The main traits of the Kotlin language
Possibilities for Android and server-side development
What distinguishes Kotlin from other languages
Writing and running code in Kotlin
1.1 A TASTE OF KOTLIN
First, what is Kotlin all about? Its a new programming language targeting the Java platform. Kotlin is concise, safe, pragmatic, and focused on interoperability with Java code. It can be used almost everywhere Java is used today - for server-side development, Android apps, and much more. Kotlin works great with all existing Java libraries and frameworks and runs with the same level of performance as Java.
Lets start with a small example to demonstrate what Kotlin looks like. This example defines a Person class, creates a collection of people, finds the oldest one, and prints the result. Even in this small piece of code, you can see many interesting features of Kotlin; weve highlighted some of them so you can easily find them later in the book. The code is explained briefly, but please dont worry if something isnt clear right away. Well discuss everything in detail later.
If youd like to try running this example, the easiest option is to use the online playground at https://try.kotl.in/ . Type in the example and click the Run button, and the code will be executed. Heres the code:
data class Person(val name: String,
val age: Int? = null)
fun main(args: Array) {
val persons = listOf(Person("Alice"),
Person("Bob", age = 29))
val oldest = persons.maxBy { it.age ?: 0 }
println("The oldest is: $oldest")
}
// The oldest is: Person(name=Bob, age=29)
'data' class |
nullable type (Int?); default value for argument |
top-level function |
named argument |
lambda expression; "elvis" operator |
string template |
autogenerated 'toString' |
You declare a simple data class with two properties: name and age . The age property is null by default (if it isnt specified). When creating the list of people, you omit Alices age, so the default value null is used. Then you use the function maxBy to find the oldest person in the list. The lambda expression passed to the function takes one parameter, and you use it as the default name of that parameter. The Elvis operator ?: returns zero if age is null . Because Alices age isnt specified, the Elvis operator replaces it with zero, so Bob wins the prize of being the oldest person.
Do you like what youve seen? Read on to learn more and become a Kotlin expert. We hope that soon youll see such code in your own projects, not only in this book.
1.2 KOTLINS PRIMARY TRAITS
You probably already have an idea what kind of language Kotlin is. Lets look at its key attributes in more detail. First of all, lets see what kinds of applications you can build with Kotlin.
1.2.1 Target platforms: server-side, Android, anywhere Java runs
The primary goal of Kotlin is to provide a more concise, more productive, safer alternative to Java thats suitable in all contexts where Java is used today. Java is an extremely popular language, and its used in a broad variety of environments, from smart cards (Java Card technology) to the largest data centers run by Google, Twitter, LinkedIn, and other internet-scale companies. In most of these places, using Kotlin can help developers achieve their goals with less code and fewer annoyances along the way.
The most common areas to use Kotlin are these:
Building server-side code (typically, backends of web applications)
Building mobile applications that run on Android devices
But Kotlin works in other contexts as well. For example, you can use the Intel Multi-OS Engine .
In addition to Java, Kotlin can also be compiled to JavaScript, allowing you to run Kotlin code in the browser. But as of this writing, JavaScript support is still being explored and prototyped at JetBrains, so its out of scope for this book. Other platforms are also under consideration for future versions of the language.
As you can see, Kotlins target is quite broad. Kotlin doesnt focus on a single problem domain or address a single type of challenge faced by software developers today. Instead, it provides across-the-board productivity improvements for all tasks that come up during the development process. It gives you an excellent level of integration with libraries that support specific domains or programming paradigms. Lets look next at the key qualities of Kotlin as a programming language.
Next page