Grey - Programming the Network with Perl
Here you can read online Grey - Programming the Network with Perl full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2021, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
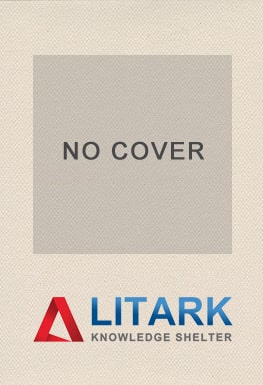
Programming the Network with Perl: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Programming the Network with Perl" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Programming the Network with Perl — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Programming the Network with Perl" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Programming the Network with PERL
Dean Grey
Programming the Network with PERL
Meet Perl
This chapter introduces Perl to the non-Perl programmer. Competent Perl pro- grammers need only skim through this material.
The main objective of this chapter is to provide sufficient Perl to allow read- ers to comfortably work through the rest of Programming the Network with Perl . Another is to show that Perl is a rather special programming technology: inter- esting, powerful, useful and fun.
Newcomers to Perl are advised to work through a good introductory Perl text. See the Print Resources section at the end of this chapter for suggestions.
For the purposes of this chapter, it is assumed that the reader is already a programmer. Veterans of the C type languages may find much of Perl familiar. However, although Perl may look a lot like C, its behaviour is oftentimes a lit- tle strange, and can consequently be quite unlike C. Fans of other programming languages will, initially, just find Perl to be strange. Perl does not set out to be strange, but it is sometimes so unlike the mainstream programming languages that the differences are seen as strangeness in the eyes of traditional program- ming folk. Which leads into the first bit of Perl strangeness: default behaviour .
1.1 Perls Default Behaviour
Unlike other programming languages, Perl assumes a lot. It does a lot of things by default, and unless told otherwise, a Perl program will inherit this default behaviour. Think of this as Perls way of giving programmers something for noth- ing. This is unlike the vast majority of programming languages, which generally assume nothing, and where nothing is free.
1.1.1 Our first Perl program
A simple example will help to illustrate this default behaviour:
#! /usr/bin/perl -w
while (<>)
{
print;
}
The first line (all that #! stuff) looks a little strange, so let us conveniently ignore it, for now. The core of this program is the while loop, which is printing something with a print statement. Just what is getting printed is not clear, but as the print statement is in a loop, the assumption is that the printing is happening over and over again (as long as the condition of the while loop is true). But, what is getting printed?
The answer lies in the bit of code that has not been explained yet. No, not the strange first line that is still too strange to discuss it is the other bit, the <> bit. If you are reading this and saying that cannot do much, then welcome to the wonderful world of Perl!
The <> is a Perl operator which, unless Perl is told otherwise, hooks a program up to standard input and, when it appears in code, returns a line from standard input to the program. Magically, the line of input comes into the program at the top of the while loop, then makes its way (magically, again) to the print statement and gets printed!
Now, if a selection of lines is fed to this program, they will all get printed one after the other (remember: the print statement is within a loop, so the code keeps executing while some condition is true). In this case, the program keeps going while there are input lines to process. To get lines into the program, pass them in from the keyboard (the hard way), or from a file (the easy way).
Use the vi editor to create a file containing the Perl code as shown above. For
want of a better name (and a distinct lack of imagination), let us call this program
firs t . At the Linux command prompt, enter the following command to test the programs functionality:
perl first /etc/passwd
The program should print each line from the /etc/passwd system file to the screen, one line at a time, until it runs out of lines.
If something other than the content of the file appears, check to see if the Perl code has been typed in correctly. You can ask Perl to check code for syntax errors using the -c command-line switch. That is, typing perl -c first will check the first program for errors, but not run it.
Borrowed rather shamelessly from Chapter of Nigel Chapmans book: Perl: The Programmers
Companion . See the Print Resources section at the end of this chapter.
Perls Default Behaviour
So, this program will, by default, use the filename from the command-line as standard input to the program. Perl finds the file, opens it, keeps reading from the file one line at a time until there are no more lines to read, then closes the file when the program ends. And all this behaviour happens by default .
As if that were not enough, the program actually does more. If it is provided with more than one filename on the command-line, as follows:
perl first /etc/passwd /etc/inittab .bash_profile
it not only prints all the lines from /etc/passwd but, when it is done, moves onto /etc/inittab and prints all the lines in that file, before moving onto
.bash_profile and printing any lines contained therein. Again, all this occurs by default, free of charge, no questions asked!
A good question to ask at this point is: while the program is running, just where does Perl put the line that it reads in with <> and then prints out with prin t ? We can answer this question after introducing another bit of Perl strangeness: the default variable .
1.1.2 Perls default variable
Meet the default variable $ _ , the most used and abused variable in all of Perl. The general rule-of-thumb is this: if a piece of Perl code expects a variable to
be used and one is not provided, out of pieces of code will use $ _ .
This is exactly what happens with <> and print in the first program. The
code could have been written as follows:
#! /usr/bin/perl -w
while ($_ = <>)
{
print $_;
}
To re-emphasize, this code is functionally identical to the code in the first pro- gram (albeit, somewhat more explicit).
1.1.3 The strange first line explained
All that remains of the first program is an explanation of the strange #! line. This line is actually doing two things. As the # symbol in Perl indicates the start of a comment that extends to the end of the current line, the strange line is, first and foremost, a comment that is ignored by the Perl interpreter. Secondly, if the very first character of a file is # followed by a ! , then the line takes on special meaning on Linux (and UNIX-like) systems. The #! combination tells the command processor to run the command identified by the rest of the line (in this
case, it is the /usr/bin/perl -w part), and then send the rest of the file to it as standard input. So, make the first program executable on Linux using the following command:
chmod +x first
and then invoke the program as follows:
./first /etc/passwd /etc/inittab .bash_profile
Linux will find the Perl interpreter (rather conveniently called per l ) located in the
/usr/bin directory and run the contents of the first file through it. This is a
cool feature of Linux (and UNIX), but of lesser importance if running on Mac OS
(prior to X) or one of the Windows varieties.
The -w part of the Perl command is a switch asking Perl to compile the code with extra warnings enabled. Until you know what you are doing, the advice is to always run Perl with the -w switch.
Oh, by the way (and in case you hadnt noticed), Perl statements end with the ; character, and blocks of code are enclosed in curly braces, { and }. Note, too, that Perl is case sensitive (so be careful). And Perl is an interpreter , which means that each time a program is run through Perl, the interpreter scans the code for errors, converts the code to Perls internal bytecode format, optimizes the bytecode, then runs it. If this all sounds slow, do not worry, in the big scheme of things, it really is not. Once a Perl program is actually running inside the interpreter, its performance compares favourably with the traditional compiled languages.
Next pageFont size:
Interval:
Bookmark:
Similar books «Programming the Network with Perl»
Look at similar books to Programming the Network with Perl. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Programming the Network with Perl and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.