Tapir Liu - Go Generics 101 (2022/08/29)
Here you can read online Tapir Liu - Go Generics 101 (2022/08/29) full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
Go Generics 101 (2022/08/29): summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Go Generics 101 (2022/08/29)" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Go Generics 101 (2022/08/29) — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Go Generics 101 (2022/08/29)" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
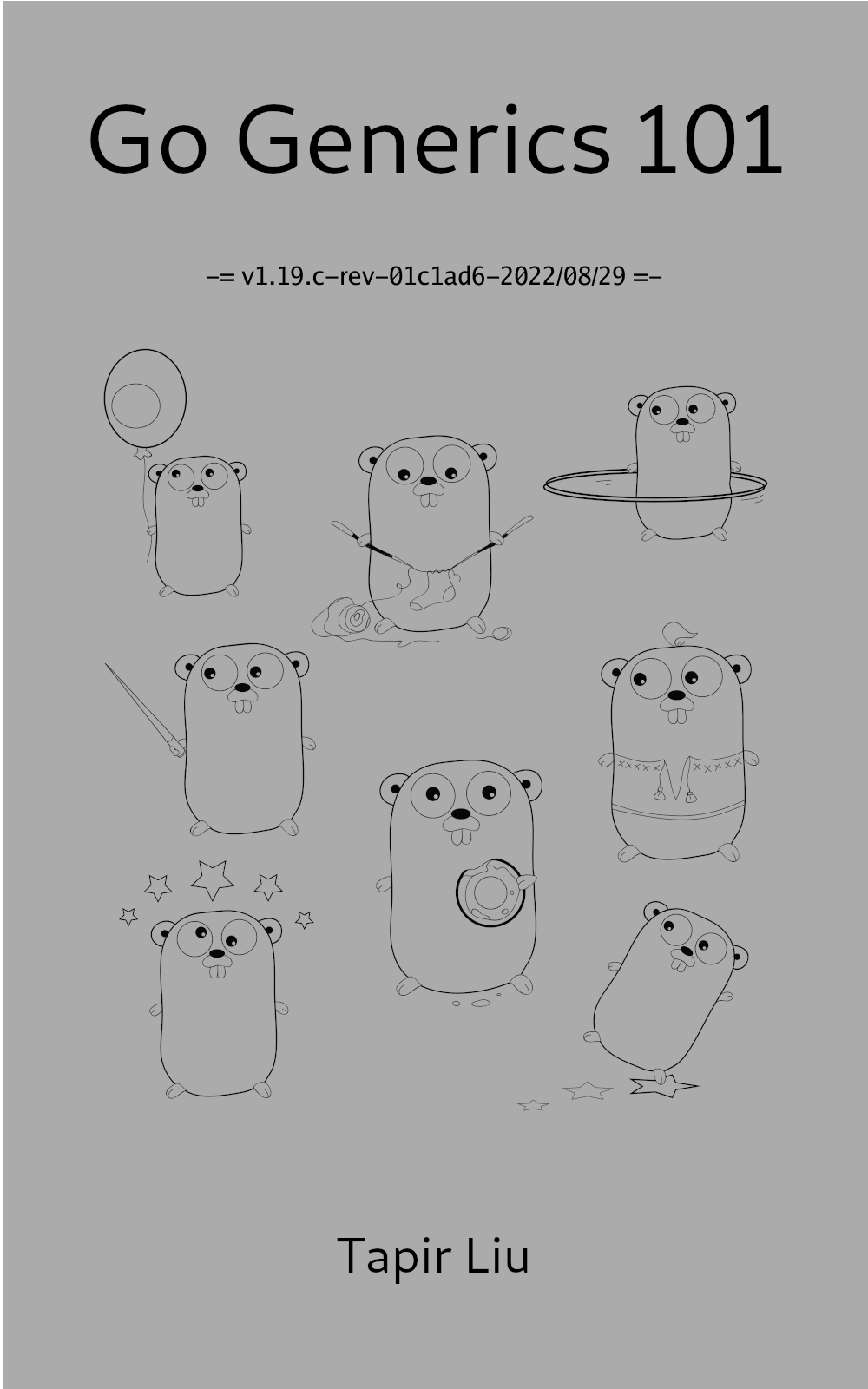
Firstly, thanks to the whole Go community. An active and responsive community guarantees the completion this book.
Specially, I want to give thanks to the following people who helped me understand some implementation details in the official standard compiler and runtime: Robert Griesemer, Ian Lance Taylor, Axel Wagner, Keith Randall, findleyr, Bob Glickstein, Jan Mercl, Brian Candler, Awad Diar, etc. I'm sorry if I forgot mentioning somebody in above lists. There are so many kind and creative gophers in the Go community that I must have missed out on someone.
I also would like to thank all gophers who ever made influences on the Go 101 book, be it directly or indirectly, intentionally or unintentionally.
Thanks to Olexandr Shalakhin for the permission to use one of the wonderful gopher icon designs as the cover image. And thanks to Renee French for designing the lovely gopher cartoon character.
Thanks to the authors of the following open source software and libraries used in building this book:
- golang, https://go.dev/
- gomarkdown, https://github.com/gomarkdown/markdown
- goini, https://github.com/zieckey/goini
- go-epub, https://github.com/bmaupin/go-epub
- pandoc, https://pandoc.org
- calibre, https://calibre-ebook.com/
- GIMP, https://www.gimp.org
Thanks the gophers who ever reported mistakes in this book or made corrections for this book:ivanburak, Caio Leonhardtetc.
Starting from version 1.18 (the current latest version), Go supports custom generics.
This book talks about the custom generics feature of Go programming language.The content in this book includes:
- custom generic syntax
- type constraints and type parameters
- type arguments and type inference
- how to write valid custom generic code
- current implementation/design restrictions
A reader needs to be familiar with Go general programming to read this book.In particular, readers of this book should be familiar withGo type system,including Go built-in generics, which and Go custom generics are two different systems.
Currently, the book mainly focuses on the syntax of, and concepts in, custom generics.More practical examples will be provided when I get more experiences of using custom generics.
The main purpose of custom generics is to avoid code repetitions,or in other words, to increase code reusability.
For some situations, generics could also lead to cleaner code and simpler APIs(not always).
For some situations, generics could also improve code execution performance(again not always).
Before version 1.18, for many Go programmers, the lack of custom generics caused pains in Go programming under some situations.
Indeed, the pains caused by the lack of custom generics were alleviated to a certain extend by the following facts.
- Since version 1.0, Go has been supported built-in generics, which include some built-in generic type kinds (such as map and channel) and generic functions (
new
,make
,len
,close
, etc). - Go supports reflection well (through interfaces and the
reflect
standard package). - Repetitive code could be generated automatically by using some tools (such as the
//go:generate
comment directive supported by the official Go toolchain).
However, the pains are still there for many use cases.The demand for custom generics became stronger and stronger.In the end, the Go core team decided to support custom generics in Go.
For all sorts of reasons, including considerations of syntax/semantics backward compatibility and implementation difficulties, the Go core team settled down on the type parameters proposal to implement custom generics.
The first Go version supporting custom generics is 1.18.
The type parameters proposal tries to solve many code reuse problems, but not all.And please note that, not all the features mentioned in the parameters proposal have been implemented yet currently (Go 1.19). The custom generics design and implementation will continue to evolve and get improved in future Go versions. And please note that the proposal is not the ceiling of Go custom generics.
Despite the restrictions (temporary or permanent ones) in the current Go custom generics design and implementation,I also have found there are some details which are handled gracefully and beautifully in the implementation.
Although Go custom generics couldn't solve all code reuse problems,personally, I believe Go custom generics will be used widely in future Go programming.
In the custom generics world, a type may be declared as a generic type,and a function may be declared as generic function.In addition, generic types are defined types, so they may have methods.
The declaration of a generic type, generic function, or method of a generic typecontains a type parameter list part, which is the main difference fromthe declaration of an ordinary type, function, or method.
Firstly, let's view an example to show how generic types look like.It might be not a perfect example, but it does show the usefulness of custom generic types.
package mainimport "sync"type Lockable[T any] struct { sync.Mutex Data T}func main() { var n Lockable[uint32] n.Lock() n.Data++ n.Unlock() var f Lockable[float64] f.Lock() f.Data += 1.23 f.Unlock() var b Lockable[bool] b.Lock() b.Data = !b.Data b.Unlock() var bs Lockable[[]byte] bs.Lock() bs.Data = append(bs.Data, "Go"...) bs.Unlock()}
In the above example, Lockable
is a generic type.Comparing to non-generic types, there is an extra part, a type parameter list,in the declaration (specification, more precisely speaking) of a generic type.Here, the type parameter list of the Lockable
generic type is [T any]
.
A type parameter list may contain one and more type parameter declarationswhich are enclosed in square brackets and separated by commas.Each parameter declaration is composed of a type parameter name and a (type) constraint.For the above example, T
is the type parameter name and any
is the constraint of T
.
Please note that any
is a new predeclared identifier introduced in Go 1.18.It is an alias of the blank interface type interface{}
.We should have known that all types implements the blank interface type.
(Note, generally, Go 101 books don't say a type alias is a type.They just say a type alias denotes a type.But for convenience, the Go Generics 101 book often says any
is a type.)
We could view constraints as types of (type parameter) types.All type constraints are actually interface types.Constraints are the core of custom generics andwill be explained in detail in the next chapter.
T
denotes a type parameter type.Its scope begins after the name of the declared generic typeand ends at the end of the specification of the generic type.Here it is used as the type of the Data
field.
Since Go 1.18, value types fall into two categories:
- type parameter type;
- ordinary types.
Before Go 1.18, all values types are ordinary types.
A generic type is a defined type.It must be instantiated to be used as value types.The notation Lockable[uint32]
is called an instantiated type (of the generic type Lockable
).In the notation,
Font size:
Interval:
Bookmark:
Similar books «Go Generics 101 (2022/08/29)»
Look at similar books to Go Generics 101 (2022/08/29). We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Go Generics 101 (2022/08/29) and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.