Tapir Liu - Go Details & Tips 101 (2022/08/29)
Here you can read online Tapir Liu - Go Details & Tips 101 (2022/08/29) full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
Go Details & Tips 101 (2022/08/29): summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Go Details & Tips 101 (2022/08/29)" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Go Details & Tips 101 (2022/08/29) — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Go Details & Tips 101 (2022/08/29)" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
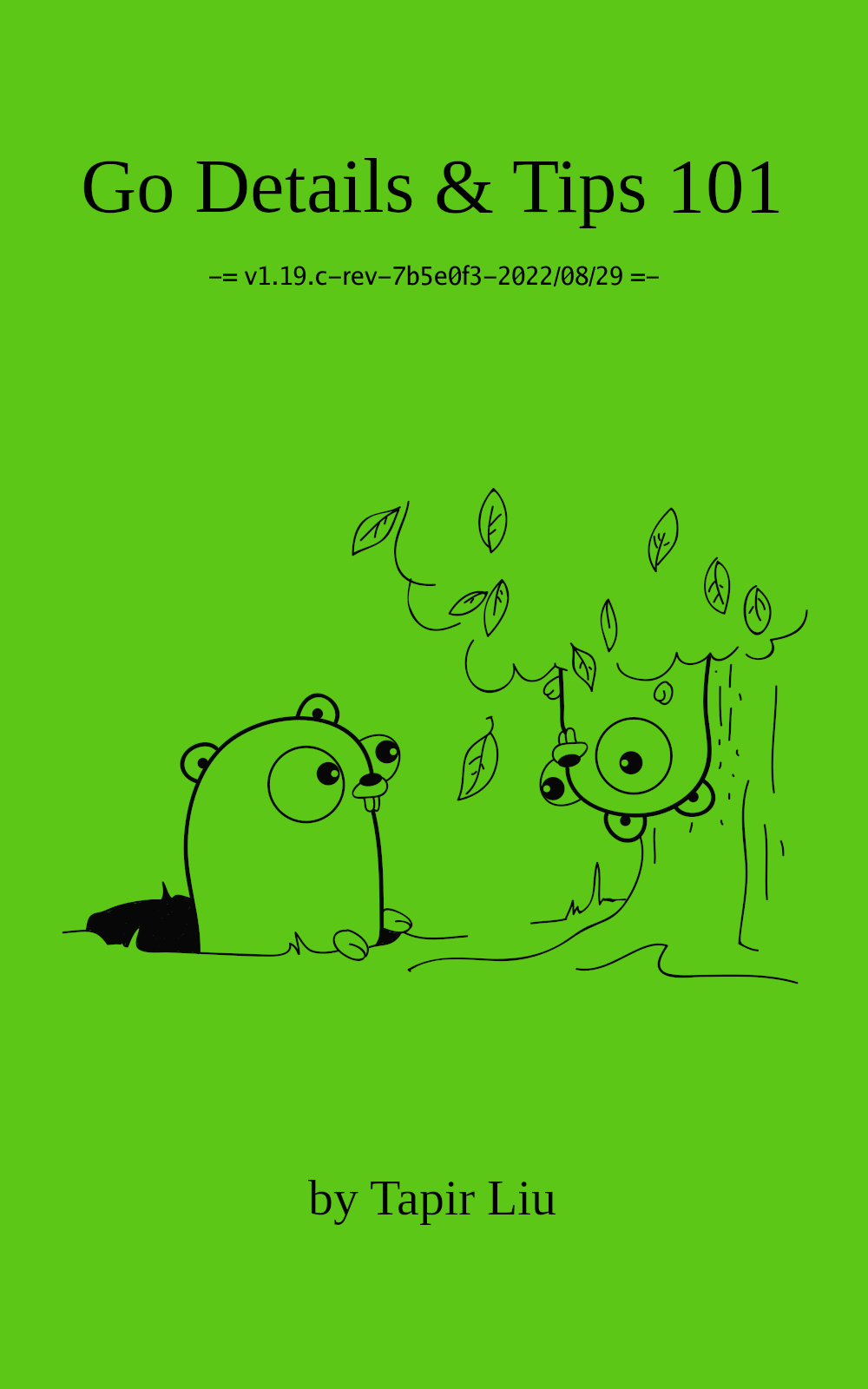
Some of the details and tips in this book are collected from the Internet,some ones are found by myself.I will try to list the source of a detail if it is possible.But I'm sorry that it is impossible task to do this for every detail.
Thanks to Olexandr Shalakhin for the permission to use one of the wonderful gopher icon designs in the cover image. And thanks to Renee French for designing the lovely gopher cartoon character.
Thanks to the authors of the following open source software and libraries, which are used in building this book:
- golang, https://go.dev/
- gomarkdown, https://github.com/gomarkdown/markdown
- goini, https://github.com/zieckey/goini
- go-epub, https://github.com/bmaupin/go-epub
- pandoc, https://pandoc.org
- calibre, https://calibre-ebook.com/
- GIMP, https://www.gimp.org
Thanks to all contributors for improving this book, includingcortes-, Yang Yang,etc.
This book collects many details and provides several tips in Go programming.The details and tips are categorized into
- syntax and semantics related
- conversions related
- comparisons related
- runtime related
- standard packages related
Most of the details are Go specific, but several of them are language independent.
Tapir is the author of this book.He also wrote the Go 101 book.He is planning to write some other Go 101 series books.Please look forward to.
Tapir was ever (maybe will be again) an indie game developer.You can find his games here: tapirgames.com.
Welcome to improve this book by submitting corrections to Go 101 issue list (https://github.com/go101/go101) for all kinds of mistakes, such as typos, grammar errors, wording inaccuracies, wrong explanations, description flaws, code bugs, etc.
It is also welcome to send your feedback to the Go 101 twitter account: @go100and1 (https://twitter.com/go100and1).
The size of a struct type without non-zero-size fields is zero.The size of an array type which length is zero or which element size is zero is also zero.These could be proved by the following program,which prints three zeros.
package mainimport "unsafe"type A [0][256]inttype S struct { x A y [1<<30]A z [1<<30]struct{}}type T [1<<30]Sfunc main() { var a A var s S var t T println(unsafe.Sizeof(a)) // 0 println(unsafe.Sizeof(s)) // 0 println(unsafe.Sizeof(t)) // 0}
In Go, sizes are often denoted as int
values.That means the largest possible length of an array is MaxInt, which value is 2^63-1 on 64-bit OSes.However, the lengths of arrays with non-zero element sizes are hard limited by the official standard Go compiler and runtime.
An example:
var x [1<<63-1]struct{} // okayvar y [2000000000+1]byte // compilation errorvar z = make([]byte, 1<<49) // panic: runtime error: makeslice: len out of range
In the current official standard Go compiler implementation (version 1.19),all local zero-size values allocated on heap share the same address.For example, the following prints false
twice, then prints true
twice.
package mainvar g *[0]intvar a, b [0]int//go:noinlinefunc f() *[0]int { return new([0]int)}func main() { // x and y are allocated on stack. var x, y, z, w [0]int // Make z and w escape to heap. g = &z; g = &w println(&b == &a) // false println(&x == &y) // false println(&z == &w) // true println(&z == f()) // true}
Please note that, the outputs of the above program depend on specific compilers.The outputs might be different for future official standard Go compiler versions.
In the following code, the size of the type Tz
is larger than the type Ty
.
package mainimport "unsafe"type Ty struct { _ [0]func() y int64}type Tz struct { z int64 _ [0]func()}func main() { var y Ty var z Tz println(unsafe.Sizeof(y)) // 8 println(unsafe.Sizeof(z)) // 16}
Why the size of the type Tz
is larger?
In the current standard Go runtime implementation, as long as a memory block is referenced by at least one alive pointer, that memory block will not be viewed as garbage and will not be collected.
All the fields of an addressable struct value can be taken addresses. If the size of the final field in a non-zero-size struct value is zero, then taking the address of the final field in the struct value will return an address which is beyond the allocated memory block for the struct value. The returned address may point to another allocated memory block which closely follows the one allocated for the non-zero-size struct value. As long as the returned address is stored in an alive pointer value, the other allocated memory block will not get garbage collected, which may cause memory leaking.
To avoid the kind of memory leak problems, the standard Go compiler will ensure that taking the address of the final field in a non-zero-size struct will never return an address which is beyond the allocated memory block for the struct. The standard Go compiler implements this by padding some bytes after the final zero-size field when needed.
So at least one byte is padded after the final (zero) field of the type Tz
.This is why the size of the type Tz
is larger than Ty
.
In fact, on 64-bit OSes, 8 bytes are padded after the final (zero) field of Tz
.To explain this, we should know two facts in the official standard compiler implementation:
- The alignment guarantee of a struct type is the largest alignment guarantee of its fields.
- A size of a type is always a multiple of the alignment guarantee of the type.
The first fact explains why the alignment guarantee of the type Tz
is 8 (which is the alignment guarantee of the builtin int64
type).The second fact explains why the size of the type Tz
is 16.
Source: https://github.com/golang/go/issues/9401
for i in 0..N
in some other languagesWe could use a for-range
loop to simulate for i in 0..N
loops in some other languages,like the following code shows.
package mainconst N = 8var n = 8func main() { for i := range [N]struct{}{} { println(i) } for i := range [N][0]int{} { println(i) } for i := range make([][0]int, n) { println(i) }}
The steps of the first two loops must be known at compile time, whereas the last one has not this requirement. But the last one allocates a little more memory (on stack, for the slice header).
For example, each slice in the following code is created in a different way.
package mainfunc main() { var s0 = make([]int, 100) var s1 = []int{99: 0} var s2 = (&[100]int{})[:] var s3 = new([100]int)[:] // 100 100 100 100 println(len(s0), len(s1), len(s2), len(s3))}
for i, v = range aContainer
actually iterates a copy of aContainer
For example, the following program will print 123
instead of 189
.
package mainfunc main() { var a = [...]int{1, 2, 3} for i, n := range a { if i == 0 { a[1], a[2] = 8, 9 } print(n) }}
Next pageFont size:
Interval:
Bookmark:
Similar books «Go Details & Tips 101 (2022/08/29)»
Look at similar books to Go Details & Tips 101 (2022/08/29). We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Go Details & Tips 101 (2022/08/29) and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.