Apple Inc. - Using Swift with Cocoa and Objective-C
Here you can read online Apple Inc. - Using Swift with Cocoa and Objective-C full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2014, publisher: Apple Inc., genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
Using Swift with Cocoa and Objective-C: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Using Swift with Cocoa and Objective-C" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Using Swift with Cocoa and Objective-C — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Using Swift with Cocoa and Objective-C" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Swift is designed to provide seamless compatibility with Cocoa and Objective-C. You can use Objective-C APIs (ranging from system frameworks to your own custom code) in Swift, and you can use Swift APIs in Objective-C. This compatibility makes Swift an easy, convenient, and powerful tool to integrate into your Cocoa app development workflow.
This guide covers three important aspects of this compatibility that you can use to your advantage when developing Cocoa apps:
Interoperability lets you interface between Swift and Objective-C code, allowing you to use Swift classes in Objective-C and to take advantage of familiar Cocoa classes, patterns, and practices when writing Swift code.
Mix and match allows you to create mixed-language apps containing both Swift and Objective-C files that can communicate with each other.
Migration from existing Objective-C code to Swift is made easy with interoperability and mix and match, making it possible to replace parts of your Objective-C apps with the latest Swift features.
Before you get started learning about these features, you need a basic understanding of how to set up a Swift environment in which you can access Cocoa system frameworks.
To start experimenting with accessing Cocoa frameworks in Swift, create a Swift-based app from one of the Xcode templates.
To create a Swift project in Xcode
Choose File > New > Project > (iOS or OS X) > Application > your template of choice.
Click the Language pop-up menu and choose Swift.
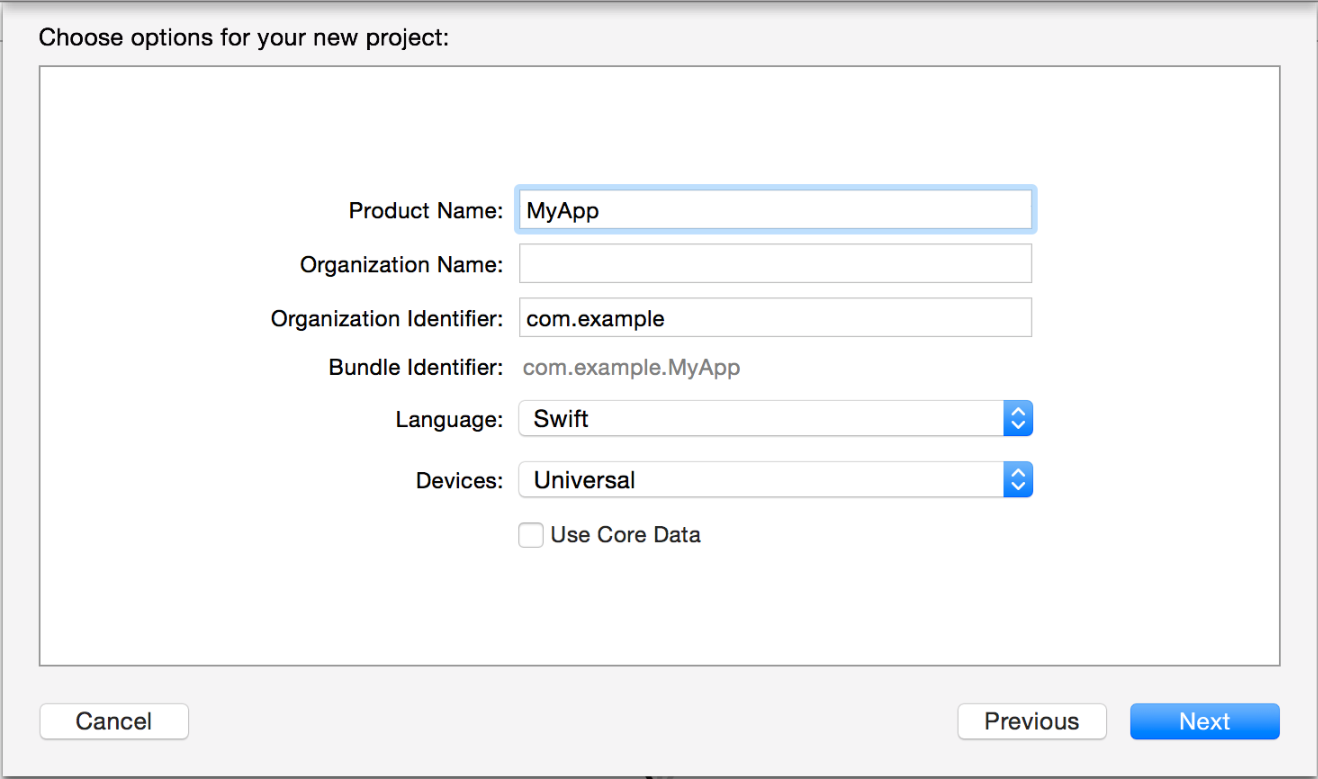
A Swift projects structure is nearly identical to an Objective-C project, with one important distinction: Swift has no header files. There is no explicit delineation between the implementation and the interface, so all the information about a particular class resides in a single .swift
file.
From here, you can start experimenting by writing Swift code in the app delegate, or you can create a new Swift class file by choosing File > New > File > (iOS or OS X) > Source > Swift.
After you have your Xcode project set up, you can import any framework from the Cocoa platform to start working with Objective-C from Swift.
Any Objective-C framework (or C library) thats accessible as a module can be imported directly into Swift. This includes all of the Objective-C system frameworkssuch as Foundation, UIKit, and SpriteKitas well as common C libraries supplied with the system. For example, to import Foundation, simply add this import statement to the top of the Swift file youre working in:
Swift
import
Foundation
This import makes all of the Foundation APIsincluding NSDate
, NSURL
, NSMutableData
, and all of their methods, properties, and categoriesdirectly available in Swift.
The import process is straightforward. Objective-C frameworks vend APIs in header files. In Swift, those header files are compiled down to Objective-C modules, which are then imported into Swift as Swift APIs. The importing determines how functions, classes, methods, and types declared in Objective-C code appear in Swift. For functions and methods, this process affects the types of their arguments and return values. For types, the process of importing can do the following things:
Remap certain Objective-C types to their equivalents in Swift, like
id
toAnyObject
Remap certain Objective-C core types to their alternatives in Swift, like
NSString
toString
Remap certain Objective-C concepts to matching concepts in Swift, like pointers to optionals
In Interoperability, youll learn more about these mappings and about how to leverage them in your Swift code.
The model for importing Swift into Objective-C is similar to the one used for importing Objective-C into Swift. Swift vends its APIssuch as from a frameworkas Swift modules. Alongside these Swift modules are generated Objective-C headers. These headers vend the APIs that can be mapped back to Objective-C. Some Swift APIs do not map back to Objective-C because they leverage language features that are not available in Objective-C. For more information on using Swift in Objective-C, see .
Note
You cannot import C++ code directly into Swift. Instead, create an Objective-C or C wrapper for C++ code.
Interoperability is the ability to interface between Swift and Objective-C in either direction, letting you access and use pieces of code written in one language in a file of the other language. As you begin to integrate Swift into your app development workflow, its a good idea to understand how you can leverage interoperability to redefine, improve, and enhance the way you write Cocoa apps.
One important aspect of interoperability is that it lets you work with Objective-C APIs when writing Swift code. After you import an Objective-C framework, you can instantiate classes from it and interact with them using native Swift syntax.
To instantiate an Objective-C class in Swift, you call one of its initializers with Swift syntax. When Objective-C init
methods come over to Swift, they take on native Swift initializer syntax. The init prefix gets sliced off and becomes a keyword to indicate that the method is an initializer. For init
methods that begin with initWith, the With also gets sliced off. The first letter of the selector piece that had init or initWith split off from it becomes lowercase, and that selector piece is treated as the name of the first argument. The rest of the selector pieces also correspond to argument names. Each selector piece goes inside the parentheses and is required at the call site.
For example, where in Objective-C you would do this:
Objective-C
UITableView * myTableView = [[ UITableView alloc ] initWithFrame : CGRectZero style : UITableViewStyleGrouped ];
In Swift, you do this:
Swift
let
myTableView
:UITableView
=UITableView
(frame
:CGRectZero
,style
: .Grouped
)
You dont need to call alloc
; Swift correctly handles this for you. Notice that init doesnt appear anywhere when calling the Swift-style initializer.
You can be explicit in typing the object during initialization, or you can omit the type. Swifts type inference correctly determines the type of the object.
Swift
let
myTextField
=UITextField
(frame
:CGRect
(x
:0.0
,y
:0.0
,width
:200.0
,height
:40.0
))
These UITableView
and UITextField
objects have the same familiar functionality that they have in Objective-C. You can use them in the same way you would in Objective-C, accessing any properties and calling any methods defined on the respective classes.
For consistency and simplicity, Objective-C factory methods get mapped as convenience initializers in Swift. This mapping allows them to be used with the same concise, clear syntax as initializers. For example, whereas in Objective-C you would call this factory method like this:
Font size:
Interval:
Bookmark:
Similar books «Using Swift with Cocoa and Objective-C»
Look at similar books to Using Swift with Cocoa and Objective-C. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Using Swift with Cocoa and Objective-C and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.