Modern Assembly Language Programming with the ARM Processor
First Edition
Larry D. Pyeatt

Copyright
Newnes is an imprint of Elsevier
The Boulevard, Langford Lane, Kidlington, Oxford OX5 1GB, UK
50 Hampshire Street, 5th Floor, Cambridge, MA 02139, USA
Copyright 2016 Elsevier Inc. All rights reserved.
No part of this publication may be reproduced or transmitted in any form or by any means, electronic or mechanical, including photocopying, recording, or any information storage and retrieval system, without permission in writing from the publisher. Details on how to seek permission, further information about the Publishers permissions policies and our arrangements with organizations such as the Copyright Clearance Center and the Copyright Licensing Agency, can be found at our website: www.elsevier.com/permissions.
This book and the individual contributions contained in it are protected under copyright by the Publisher (other than as may be noted herein).
Notices
Knowledge and best practice in this field are constantly changing. As new research and experience broaden our understanding, changes in research methods, professional practices, or medical treatment may become necessary.
Practitioners and researchers must always rely on their own experience and knowledge in evaluating and using any information, methods, compounds, or experiments described herein. In using such information or methods they should be mindful of their own safety and the safety of others, including parties for whom they have a professional responsibility.
To the fullest extent of the law, neither the Publisher nor the authors, contributors, or editors, assume any liability for any injury and/or damage to persons or property as a matter of products liability, negligence or otherwise, or from any use or operation of any methods, products, instructions, or ideas contained in the material herein.
Library of Congress Cataloging-in-Publication Data
A catalog record for this book is available from the Library of Congress
British Library Cataloging-in-Publication Data
A catalogue record for this book is available from the British Library
ISBN: 978-0-12-803698-3
For information on all Newnes publications visit our website at https://www.elsevier.com/
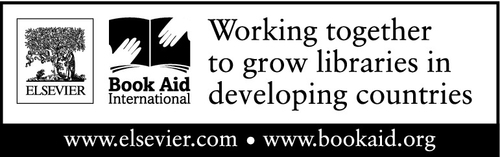
Publisher: Joe Hayton
Acquisition Editor: Tim Pitts
Editorial Project Manager: Charlotte Kent
Production Project Manager: Julie-Ann Stansfield
Designer: Mark Rogers
Typeset by SPi Global, India
List of Tables
Table 1.2 The first 21 integers (starting with 0) in various bases
Table 1.3 The ASCII control characters
Table 1.4 The ASCII printable characters
Table 1.5 Binary equivalents for each character in Hello World
Table 1.6 Binary, hexadecimal, and decimal equivalents for each character in Hello World
Table 1.7 Interpreting a hexadecimal string as ASCII
Table 1.8 Variations of the ISO 8859 standard
Table 1.9 UTF-8 encoding of the ISO/IEC 10646 code points
Table 3.1 Flag bits in the CPSR register
Table 3.2 ARM condition modifiers
Table 3.3 Legal and illegal values for #
Table 3.4 ARM addressing modes
Table 3.5 ARM shift and rotate operations
Table 4.1 Shift and rotate operations in Operand2
Table 4.2 Formats for Operand2
Table 8.1 Format for IEEE 754 half-precision
Table 8.2 Result formats for each term
Table 8.3 Shifts required for each term
Table 8.4 Performance of sine function with various implementations
Table 9.1 Condition code meanings for ARM and VFP
Table 9.2 Performance of sine function with various implementations
Table 10.1 Parameter combinations for loading and storing a single structure
Table 10.2 Parameter combinations for loading multiple structures
Table 10.3 Parameter combinations for loading copies of a structure
Table 10.4 Performance of sine function with various implementations
Table 11.1 Raspberry Pi GPIO register map
Table 11.2 GPIO pin function select bits
Table 11.3 GPPUD control codes
Table 11.4 Raspberry Pi expansion header useful alternate functions
Table 11.5 Number of pins available on each of the AllWinner A10/A20 PIO ports
Table 11.6 Registers in the AllWinner GPIO device
Table 11.7 Allwinner A10/A20 GPIO pin function select bits
Table 11.8 Pull-up and pull-down resistor control codes
Table 11.9 pcDuino GPIO pins and function select code assignments.
Table 12.1 Raspberry Pi PWM register map
Table 12.2 Raspberry Pi PWM control register bits
Table 12.3 Prescaler bits in the pcDuino PWM device
Table 12.4 pcDuino PWM register map
Table 12.5 pcDuino PWM control register bits
Table 13.1 Clock sources available for the clocks provided by the clock manager
Table 13.2 Some registers in the clock manager device
Table 13.3 Bit fields in the clock manager control registers
Table 13.4 Bit fields in the clock manager divisor registers
Table 13.5 Clock signals in the AllWinner A10/A20 SOC
Table 13.6 Raspberry Pi UART0 register map
Table 13.7 Raspberry Pi UART data register
Table 13.8 Raspberry Pi UART receive status register/error clear register
Table 13.9 Raspberry Pi UART flags register bits
Table 13.10 Raspberry Pi UART integer baud rate divisor
Table 13.11 Raspberry Pi UART fractional baud rate divisor
Table 13.12 Raspberry Pi UART line control register bits
Table 13.13 Raspberry Pi UART control register bits
Table 13.14 pcDuino UART addresses
Table 13.15 pcDuino UART register offsets
Table 13.16 pcDuno UART receive buffer register
Table 13.17 pcDuno UART transmit holding register
Table 13.18 pcDuno UART divisor latch low register
Table 13.19 pcDuno UART divisor latch high register
Table 13.20 pcDuno UART FIFO control register
Table 13.21 pcDuno UART line control register
Table 13.22 pcDuno UART line status register
Table 13.23 pcDuno UART status register
Table 13.24 pcDuno UART transmit FIFO level register
Table 13.25 pcDuno UART receive FIFO level register
Table 13.26 pcDuno UART transmit halt register
Table 14.1 The ARM user and system registers
Table 14.2 Mode bits in the PSR
Table 14.3 ARM vector table
List of Figures
Figure 1.2 Stages of a typical compilation sequence
Figure 1.3 Tables used for converting between binary, octal, and hex
Figure 1.4 Four different representations for binary integers
Figure 1.5 Complement tables for bases ten and two
Figure 1.6 A section of memory
Figure 1.7 Typical memory layout for a program with a 32-bit address space
Figure 2.1 Equivalent static variable declarations in assembly and C
Figure 3.1 The ARM processor architecture
Figure 3.2 The ARM user program registers
Figure 3.3 The ARM process status register
Figure 5.1 ARM user program registers
Figure 6.1 Binary tree of word frequencies
Figure 6.2 Binary tree of word frequencies with index added
Figure 6.3 Binary tree of word frequencies with sorted index