Why this book?
There are many ways to build the backend for an iOS application but you only need one. And depending on the complexity of the API you are going to create, different solutions work best for different applications.
Just as Rails makes it possible to set up a basic web application in a matter of minutes, Rails makes it possible to set up a basic API in a matter of minutes. But deciding how to structure your API isn't easy. While experimenting with all of the options is a fun weekend project, sometimes you just want to get going. This book will help you do just that. While your API will no doubt require some tweaking while fleshing out your iOS app, the approach we will be taking is to define and build the API first, and then consume this API through our iOS app.
The Rails portions of iOS on Rails will guide you through what we have found to be a robust, clean, flexible way of building out a JSON API with Rails. We provide code samples for GET, POST, and PATCH requests. In addition, we will explore some of the alternative approaches that we didn't choose and explain why we made the choices that we did.
The iOS portion of the book will then walk, step-by-step, through creating an iOS application that works with the Rails API you just created. The iOS application will use each endpoint to post up objects and get back necessary data for the user. Our model objects in the iOS app will correspond with the model objects in the database, and be populated with response data from the API.
Who is this book for?
This book is for a developer who wants to build an iOS application with a Rails backend. It's also a book for both a Rails developer and an iOS developer to share and use in concert to create an app quickly and with more flexibility to change it than a backend-as-a-service provider like StackMob or Parse.
The approach shared in this book is the result of our own experiments as Rails and iOS developers working together to build an application. The Rails portions of this book assume a basic working knowledge of how to build a web application with Rails as well as the Ruby programming language. The iOS portions of this book assume experience with object oriented programming and a basic familiarity with the Objective-C programming language.
This book is intended to be used as a guide rather than a recipe. While our aim is to give you all of the tools necessary to build great Rails APIs and iOS clients, it does not cover the fundamentals of Ruby, Rails or Objective-C. That being said, if any part of the book strikes you as incomplete or confusing, we are always happy to receive pull requests and issue submissions on GitHub.
Introduction to our example application and setup
Example application
We will use a fake example application called Humon to explain and demonstrate the concepts throughout this book. Humon is an app that lets you find nearby events.
In the Humon application, a user can have many events as an event owner. An event has geolocation information (latitude and longitude), which allows us to plot it on a map. A user has and belongs to many events through attendances. A user can only have one attendance per event.
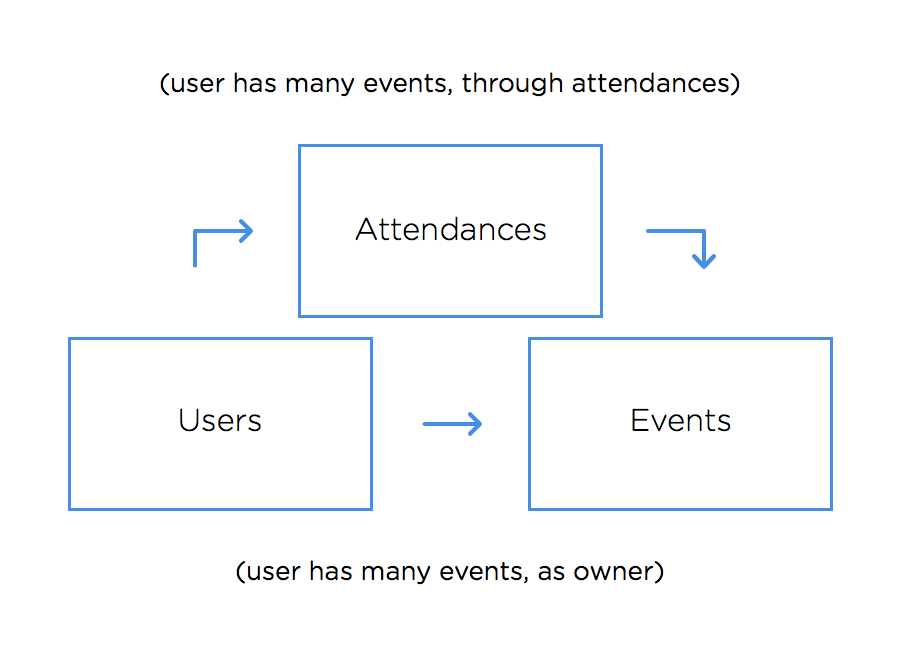
Humon database representation
The Humon application does not ask for a username or password. Instead, we will use an ID unique to the device ('device token') to track unique users. The iOS portion of the book will discuss where this token comes from. For now, all you need to know is that users are identified by their devices. This approach does not allow for multiple users per device or a single account across devices, but it does enable users to start using the application immediately. Our desire to create the simplest application possible led us to choose immediate usability over a more complex authentication system.
We will provide code snippets in context, but you can also view the entire example application in GitHub to see how it is structured.
Setting up our project
We used Suspenders, a Rails 4 template with thoughtbot's standard defaults, to start our project. Starting a Rails app with Suspenders is simple, just follow the instructions in the README
.
While Suspenders is not required to follow along with this book, it does conveniently include all of the gems we will use to test-drive our API, including: Factory Girl, RSpec, and Shoulda Matchers. So if you choose not to use Suspenders to start your project, make sure you add those gems to your Gemfile
.
Parsing incoming JSON requests
MultiJson is an adapter for JSON parsers. Another adapter familiar to Rails developers is ActiveRecord. Just as ActiveRecord provides a common interface to database drivers like Postgres and MySQL, MultiJson provides a common interface to JSON parsers like Oj and Yajl. We get MultiJson for free with Rails because MultiJson is a dependency of ActiveSupport.
For parsing JSON, we chose the Oj gem. To use the Oj gem in your Rails application, simply add it to your Gemfile and install it with the bundle install command. We chose Oj because it is a really fast JSON parser. From the MultiJson README:
"When loading, libraries are ordered by speed. First Oj, then Yajl, then the JSON gem, then JSON pure. If no other JSON library is available, MultiJSON falls back to OkJson, a simple, vendorable JSON parser."
Generating outgoing JSON responses
There is no shortage of methods to render a JSON response. We looked into Active Model Serializers, RABL, and simply using the Rails .as_json
method. In the end we chose Jbuilder for rendering JSON because of its excellent community support (repo is maintained under the Rails organization) and the consistency of its view logic to other kinds of Rails views such as Haml, ERB, and Builder.
With Jbuilder, we render the JSON from Rails controllers like any other view. We're able to use partials like any other Rails view template to compose JSON. There's also a cache!
method that has the same method signature as ActionView::Helpers::CacheHelper
and uses Rails.cache
under the hood. We will delve into the details of views and caching in later chapters.
Versioning
Before we get started with building out our API, we must think about how we are going to handle versioning. While web developers can deploy as often as they want and users see the newly deployed code with every browser page refresh, iOS developers have both a lag time before Apple approves the new version of an app and a lag time before users update to the newest application version available. iOS applications reference the same API endpoints until a new release of the application has been downloaded. If you want to continue to support users with older versions of the iOS application you are building, you must maintain the same general JSON data structures on the backend for those users.
You will of course discover new and better ways of structuring your JSON responses as time goes on and your application grows. Once that happens, the easiest way to support old versions of the application and allow newer versions to use different JSON structures is to release a new API version.