JavaScript
The Ultimate Beginners Guide!
Andrew Johansen
Copyright 2015 by Andrew Johansen - All rights reserved.
This document is geared towards providing exact and reliable information in regards to the topic and issue covered. The publication is sold with the idea that the publisher is not required to render accounting, officially permitted, or otherwise, qualified services. If advice is necessary, legal or professional, a practiced individual in the profession should be ordered.
- From a Declaration of Principles which was accepted and approved equally by a Committee of the American Bar Association and a Committee of Publishers and Associations.
In no way is it legal to reproduce, duplicate, or transmit any part of this document in either electronic means or in printed format. Recording of this publication is strictly prohibited and any storage of this document is not allowed unless with written permission from the publisher. All rights reserved.
The information provided herein is stated to be truthful and consistent, in that any liability, in terms of inattention or otherwise, by any usage or abuse of any policies, processes, or directions contained within is the solitary and utter responsibility of the recipient reader. Under no circumstances will any legal responsibility or blame be held against the publisher for any reparation, damages, or monetary loss due to the information herein, either directly or indirectly.
Respective authors own all copyrights not held by the publisher.
The information herein is offered for informational purposes solely, and is universal as so. The presentation of the information is without contract or any type of guarantee assurance.
The trademarks that are used are without any consent, and the publication of the trademark is without permission or backing by the trademark owner. All trademarks and brands within this book are for clarifying purposes only and are the owned by the owners themselves, not affiliated with this document.
Table of Contents
Introduction
Chapter 1: JavaScript General Information
Chapter 2: The Basic Elements of the JavaScript Language
Chapter 3: JavaScripts Control Flow Statements
Chapter 4: The Different Types of Loops in JavaScript
Chapter 5: JavaScript Functions
Chapter 6: Events
Chapter 7: JavaScript and Cookies
Chapter 8: Page Redirection
Chapter 9: Dialog Boxes
Chapter 10: How to Use the Void Keyword
Chapter: 11: Debugging
Conclusion
Introduction
I want to thank you and congratulate you for getting my book
JavaScript: The Ultimate Beginners Guide!
This book will help you become an effective JavaScript user. If you want to learn the basics of the JavaScript language quickly and easily, then you must get this book now.
This book will explain important facts regarding the JavaScript language. It doesnt contain any irrelevant information. Each page holds valuable lessons, instructions and examples. After reading this book, youll be familiar with objects, functions, variables, statements and other JavaScript elements.
Thanks again for purchasing this book, I hope you enjoy it!
Chapter 1: General Information
JavaScript is an object-oriented scripting language that you can use in different platforms. Within host environments (e.g. web browsers), you may use JavaScript to gain programmatic control over an object.
This scripting language comes with a rich standard library. This library holds objects (e.g. Math, Date, Array, etc.) and core language elements (e.g. statements, operators, control structures, etc.). As of now, programmers extend JavaScripts basic features by adding extra objects. They do this to improve the languages effectiveness in completing certain tasks.
Here are two of the most popular variants of JavaScript:
- Server-side JavaScript This variant is designed to help programmers run JavaScript on a web server. For instance, server-side extensions help applications to interact with databases, conduct file changes and provide a continuous flow of information.
- Client-side JavaScript This form of JavaScript is created for browsers and their respective DOM (i.e. Document Object Model). For instance, client-side JavaScript helps applications to save elements into an HTML document and react to user actions (e.g. form input, mouse clicks, page navigation, etc.).
JavaScript and the Java Language
Java and JavaScript are two different programming languages. JavaScript has some similarities with Java (e.g. naming conventions, expression syntax, control-flow constructs, etc.). However, it doesnt have two of Javas distinctive characteristics: powerful type checking and static typing.
According to many people, these languages are completely unrelated. Netscape (the company who developed JavaScript) changed the name of the language from LiveScript to JavaScript to exploit the popularity enjoyed by Java; thus, the similarity between the names of these languages emerged because of marketing considerations.
At this point, lets discuss the main differences between these programming languages. This piece of information will help you in improving your knowledge about JavaScript and general programming.
JavaScript This is a free-form computer language. When using JavaScript, you dont have to declare all of the methods, classes and variables. You dont have to worry about the types of methods that youll use (i.e. whether they are private, public or protected). Additionally, you wont have to implement any interface for JavaScript.
Java This class-based computer language is created for type safety and quick implementation. This means that you have to use classes and their respective methods to create your programs. Javas data typing and class inheritance require strict object hierarchies. Simply put, the Java language is more complicated than JavaScript.
How to Get Started?
You dont have to download files or install programs into your computer just to use JavaScript. You can simply access your favorite web browser to create your own JavaScript applications. These days, Firefox is one of the best web browsers when it comes to JavaScript programming. Because of that, this book will focus on the tools available in Firefox browsers. If your computer doesnt have Firefox, you are advised to visit this site: https://www.mozilla.org . This browser is free and easy to use; thus, you can easily master the basics of JavaScript without spending any money.
Once you have downloaded Firefox, youll see two powerful tools created specifically for JavaScript programming. These tools are:
1. Web Console
This tool displays information about the webpage loaded on the screen. It also has a command line feature that you may use to run JavaScript statements on the loaded page.
To access Web Console, you just have to launch Firefox, click on Tools, and choose Web Console from the menu titled Developer. After activating Web Console, youll see a command line at the bottom of your screen. You may enter JavaScript expressions into that command line, then the pane at the middle of the screen will show you the results of the expressions you entered.
This feature shows results immediately youll quickly know if you have entered codes incorrectly.
Heres a screenshot of the Web Console tool:
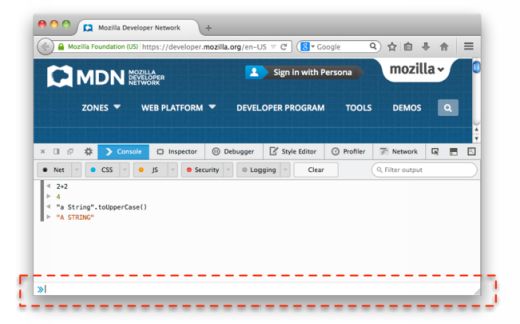
2. Scratchpad
Firefoxs Web Console is excellent for running single JavaScript expressions. Although you can use it to run multiple lines, youll find this task extremely inconvenient. Additionally, you cant use that tool to save your code snippets. Fortunately, Firefox has another tool that solves the problems discussed above. This tool is called Scratchpad.
Next page