Quick JavaScript Interview Questions
Copyright Blurb
All rights reserved. No part of this book may be reproduced in any form or by any electronic or mechanical means including information storage and retrieval systems except in the case ofbrief quotations in articles or reviews without the permission inwriting from its publisher, Sandeep Kumar Patel.
All brand names and product names used in this book aretrademarks, registered trademarks, or trade names of their respective holders. I am not associated with any product or vendor in this book.
Published By
Sandeep Kumar Patel.
Table of Contents
Quick JavaScript Interview QuestionsTable of Contents
Chapter 1 Inheritance
Chapter 2 Questions on Event
Chapter 3 Closure
Chapter 5 Questions on DOM
Chapter 6 Date Object
Chapter 7 Regular Expression
Chapter 8 Questions on Canvas APIChapter 9 Geo Location API
Chapter 10 Web Workers
Chapter 11 Local Storage
Chapter 12 File API
Chapter 13 Web RTC
Chapter 14 Drag & Drop API
Chapter 15 App Cache API
Chapter 16 Server Sent EventsChapter 17 Miscellaneous QuestionsAbout The Author
One Last Thing...
Chapter 1 Inheritance
Q. How to create a class?
ANSWER
JavaScript does not have a class definition. To mimic classes in JavaScript functions can be used to declare a class.
Example:
Lets create a student class in JavaScript which takes two parameters name and roll as property. The code will look like below,
function Student(name,roll){this.name = name;
this.roll = roll;
}
Q. How to create an object?
ANSWER
An object in JavaScript can be created using two ways:
New Keyword:
To create a student object from the above student class we can call the Student function using new keyword.
var student1 = new Student(sandeep,2)
Anonymous Object:
Anonymous objects can be created using pair of curly braces containing property name and value pairs.
Var rose = {color: red}
Q. How to declare a private and a public member?
Private members are declared using
var keyword and constructorfunction.
function Student(name,roll){var id= ABCD123;
this.name = name;
this.roll = roll;
}
When a Student object will be created the propertied name and roll will be accessible using dot operator but id will not be accessible as it behaves as a private member and return undefined on call.
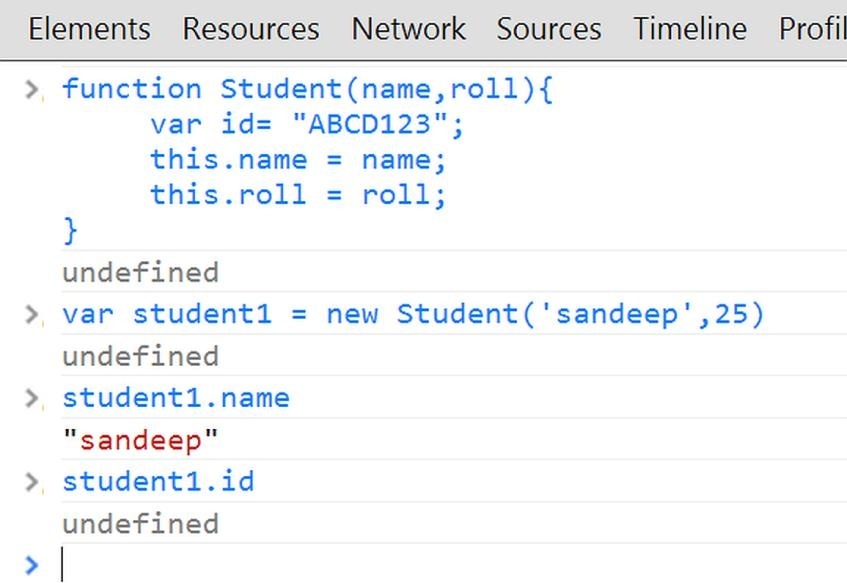
The above chrome console is showing a student1 object is created.name property is accessible as it is showing sandeep onstudent1.name call. So name is a public property for the student object. But id property is not accessible and returned undefined onstudent1.id call. This shows id is a private property in student1 object.
Q. What is prototype property?
By Using Prototype we can add new members to an existing object. Every JavaScript object has this property internally. Initially it is anempty object.
Example:function Student(name,roll){
this.name = name;
this.roll = roll;
}
var student1 = new Student('sangeeta',30);Student.prototype.mark = 100;
Checkout the below chrome console for the use of protype.
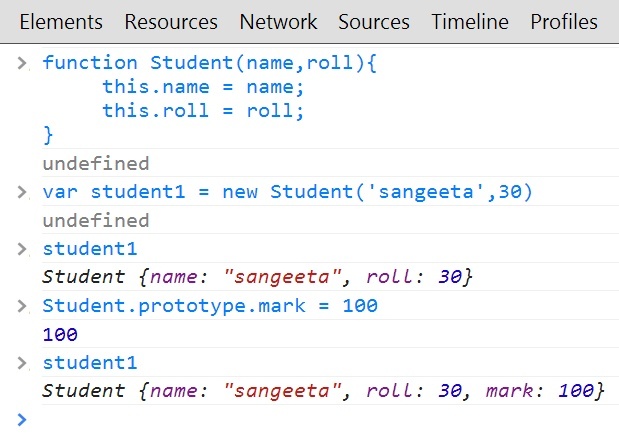
Initially the student1 object has only two properties name and roll. By using prototype a new property mark has been added to student object with a value of .Now the console shows that the markproperty is also added to the existing student1 object.
Q. What is constructor property?
ANSWER
Constructor property of an object maintains a reference to its creator function.
Example:
Let us checkout an example by creating a student object and calling the constructor property on it.
function Student(name, mark){
this.name=name;
this.mark =mark;
}
var student1 = new Student('sandeep',123);console.log(student1.constructor);
Checkout the following screen shot for above code in chromeconsole. The console log is printing the referenced function by student1 object.
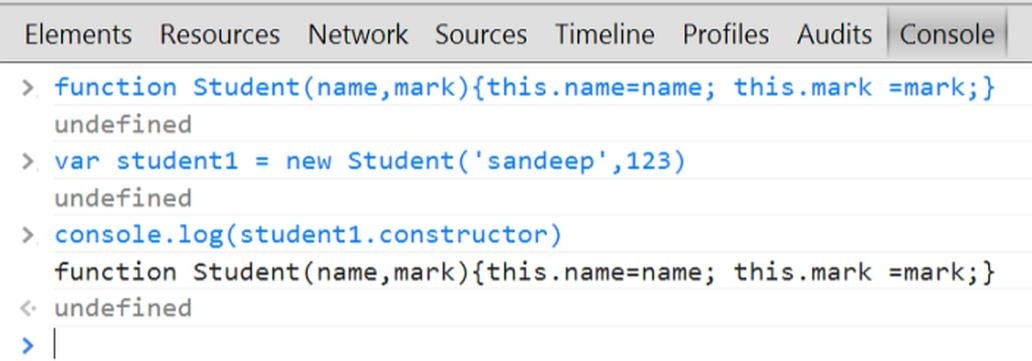
Q. How to call other class methods?
ANSWER
Using call() and apply() method we can use methods from differentcontext to the current context. It is really helpful in reusability ofcode and context binding.
call() : It is used to calls a function with a given this value and arguments provided individually.
apply(): It is used to call a function with a given this valueand arguments provided as an array.
Below code has two function getTypeOfNumber() andgetTypeOfAllNumber(). The details pf these functions are below.getTypeOfNumber : This method takes single number as
parameter and return the type either Even or Odd.
getTypeOfAllNumber : This method takes array of numbers
as parameter and return the types in an array with Even or
Odd.
var MyNumber = {
getTypeOfNumber : function(number){
var type = (number % 2 === 0) ? "Even" : "Odd";return type;
},
getTypeOfAllNumber : function (){
var result = [],i=0;
for (; i < arguments.length; i++){
var type =
MyNumber.getTypeOfNumber.call(null,arguments[i]);
result.push(type)
}
return result;
}
};
var typeOfNumber =
MyNumber.getTypeOfNumber.call(null,) console.log(typeOfNumber)
var typeOfAllNumber =
MyNumber.getTypeOfAllNumber.apply(null,[2,4,5,78,21])
console.log(typeOfAllNumber)
Below screenshot shows output of the above code Firebug console.
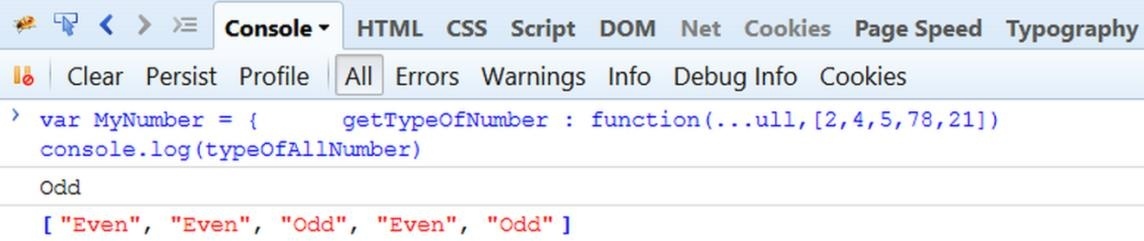
Q. Explain method overriding with an Example?
ANSWER
We can override any inbuilt method of JavaScript by declaring its definition again. The existing definition is accessible to override by the prototype property. Consider the below example, split() is anbuilt-in method for a string object .Its default behaviour to breakthe specified string to an array and is a member function of Stringclass. But we have overridden its definition using its prototypeproperty.
Below screenshot shows the inbuilt behaviour of
split() method. Ithas divided the string into an array of element.

The following screenshot shows the new overridden definition of
split () method. It is now normally returns string
I am overriden.
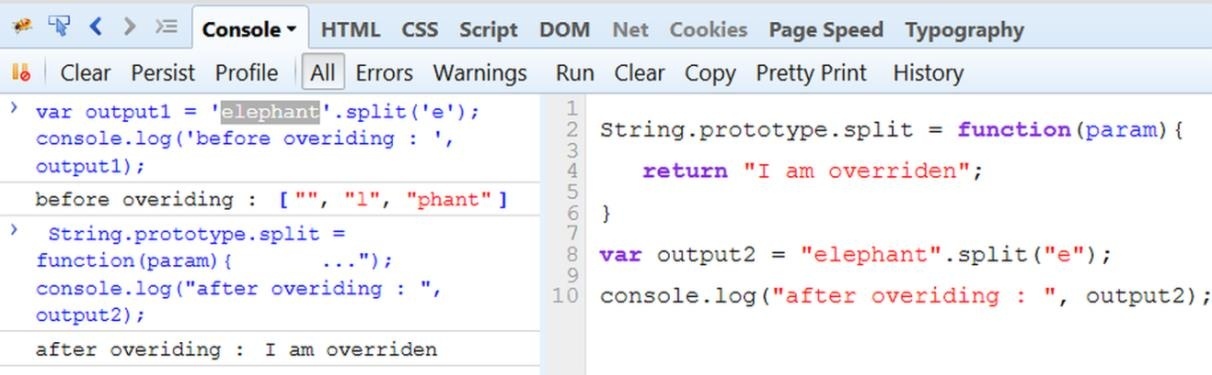
Q. How to inherit from a class?
ANSWER
Inheritance can be achieved in JavaScript using prototype property.
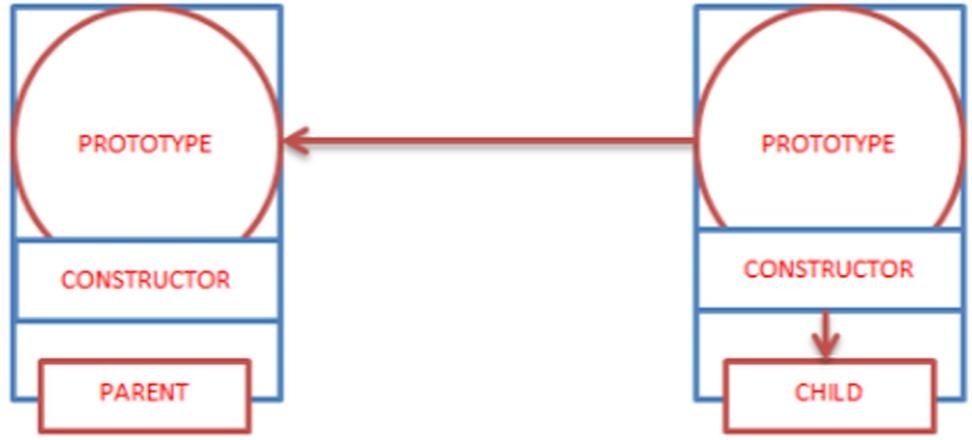
We need to follow 2 steps to create an inheritance.
Step1: Child class prototype should point to parent class object.
.prototype = new ();
Step2: Reset the child class prototype constructor to point self.