Chris Eidhof - Functional Programming in Swift
Here you can read online Chris Eidhof - Functional Programming in Swift full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2015, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
- Book:Functional Programming in Swift
- Author:
- Genre:
- Year:2015
- Rating:3 / 5
- Favourites:Add to favourites
- Your mark:
- 60
- 1
- 2
- 3
- 4
- 5
Functional Programming in Swift: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Functional Programming in Swift" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Functional Programming in Swift — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Functional Programming in Swift" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
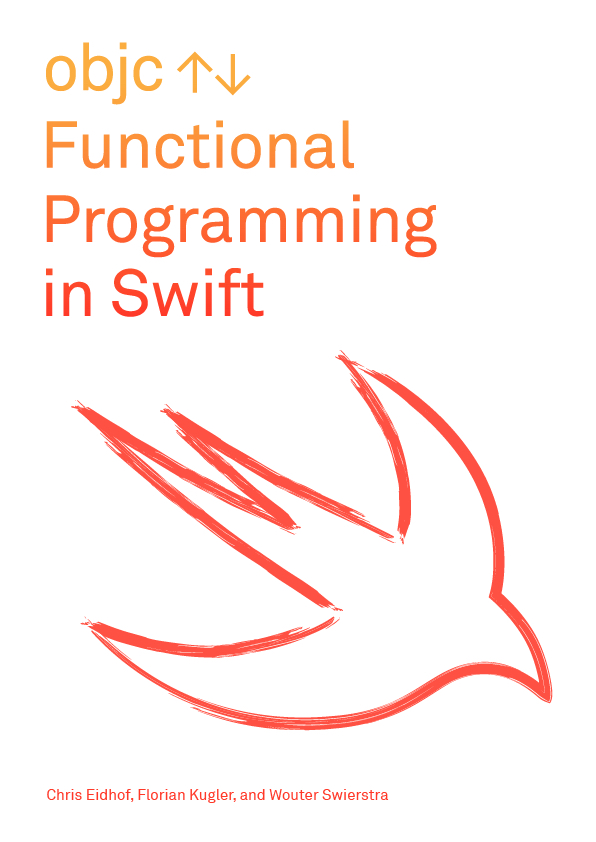
Why write this book? There is plenty of documentation on Swift readily available from Apple, and there are many more books on the way. Why does the world need yet another book on yet another programming language?
This book tries to teach you to think functionally. We believe that Swift has the right language features to teach you how to write functional programs. But what makes a program functional? And why bother learning about this in the first place?
It is hard to give a precise definition of functional programming in the same way, it is hard to give a precise definition of object-oriented programming, or any other programming paradigm for that matter. Instead, we will try to focus on some of the qualities that we believe well-designed functional programs in Swift should exhibit:
Modularity: Rather than thinking of a program as a sequence of assignments and method calls, functional programmers emphasize that each program can be repeatedly broken into smaller and smaller pieces; all these pieces can be assembled using function application to define a complete program. Of course, this decomposition of a large program into smaller pieces only works if we can avoid sharing state between the individual components. This brings us to our next point.
A Careful Treatment of Mutable State: Functional programming is sometimes (half-jokingly) referred to as value-oriented programming. Object-oriented programming focuses on the design of classes and objects, each with their own encapsulated state. Functional programming, on the other hand, emphasizes the importance of programming with values, free of mutable state or other side effects. By avoiding mutable state, functional programs can be more easily combined than their imperative or object-oriented counterparts.
Types: Finally, a well-designed functional program makes careful use of types. More than anything else, a careful choice of the types of your data and functions will help structure your code. Swift has a powerful type system that, when used effectively, can make your code both safer and more robust.
We feel these are the key insights that Swift programmers may learn from the functional programming community. Throughout this book, we will illustrate each of these points with many examples and case studies.
In our experience, learning to think functionally is not easy. It challenges the way weve been trained to decompose problems. For programmers who are used to writing for loops, recursion can be confusing; the lack of assignment statements and global state is crippling; and closures, generics, higher-order functions, and monads are just plain weird.
Throughout this book, we will assume that you have previous programming experience in Objective-C (or some other object-oriented language). We wont cover Swift basics or teach you to set up your first Xcode project, but we will try to refer to existing Apple documentation when appropriate. You should be comfortable reading Swift programs and familiar with common programming concepts, such as classes, methods, and variables. If youve only just started to learn to program, this may not be the right book for you.
In this book, we want to demystify functional programming and dispel some of the prejudices people may have against it. You dont need to have a PhD in mathematics to use these ideas to improve your code! Functional programming is not the only way to program in Swift. Instead, we believe that learning about functional programming adds an important new tool to your toolbox that will make you a better developer in any language.
As Swift evolves, well continue to make updates and enhancements to this book. Should you encounter any mistakes, or if you would like to send any other kind of feedback our way, please file an issue in our GitHub repository.
Wed like to thank the numerous people who helped shape this book. We wanted to explicitly mention some of them:
Natalye Childress is our copy editor. She has provided invaluable feedback, not only making sure the language is correct and consistent, but also making sure things are understandable.
Sarah Lincoln designed the cover and gave us feedback on the design and layout of the book.
Wouter would like to thank Utrecht University for letting him take time to work on this book.
We would like to thank the beta readers for their feedback during the writing of this book (listed in alphabetical order):
Adrian Kosmaczewski, Alexander Altman, Andrew Halls, Bang Jun-young, Daniel Eggert, Daniel Steinberg, David Hart, David Owens II, Eugene Dorfman, f-dz-v, Henry Stamerjohann, J Bucaran, Jamie Forrest, Jaromir Siska, Jason Larsen, Jesse Armand, John Gallagher, Kaan Dedeoglu, Kare Morstol, Kiel Gillard, Kristopher Johnson, Matteo Piombo, Nicholas Outram, Ole Begemann, Rob Napier, Ronald Mannak, Sam Isaacson, Ssu Jen Lu, Stephen Horne, TJ, Terry Lewis, Tim Brooks, Vadim Shpakovski.
Chris, Florian, and Wouter
Functions in Swift are first-class values, i.e. functions may be passed as arguments to other functions, and functions may return new functions. This idea may seem strange if youre used to working with simple types, such as integers, booleans, or structs. In this chapter, we will try to explain why first-class functions are useful and provide our first example of functional programming in action.
Well introduce first-class functions using a small example: a non-trivial function that you might need to implement if you were writing a Battleship-like game. The problem well look at boils down to determining whether or not a given point is in range, without being too close to friendly ships or to us.
As a first approximation, you might write a very simple function that checks whether or not a point is in range. For the sake of simplicity, we will assume that our ship is located at the origin. We can visualize the region we want to describe in Figure .
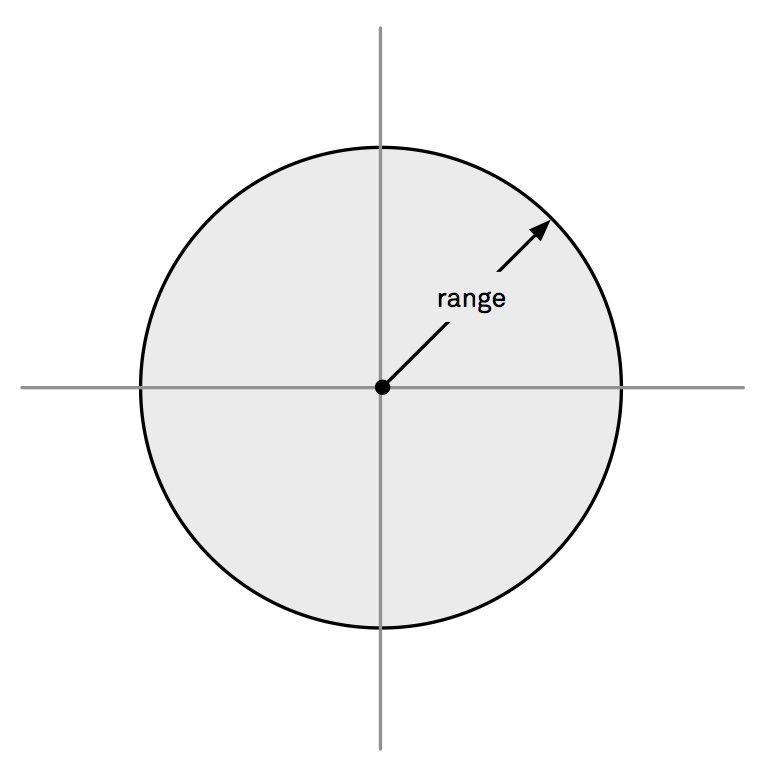
The points in range of a ship located at the origin
The first function we write, inRange1
, checks that a point is in the grey area in Figure . Using some basic geometry, we can write this function as follows:
Note that we are using Swifts typealias construct, which allows us to introduce a new name for an existing type. From now on, whenever we write Position
, feel free to read CGPoint
, a pairing of an x
and y
coordinate.
Now this works fine, if you assume that we are always located at the origin. But suppose the ship may be at a location, ownposition
, other than the origin. We can update our visualization in Figure .
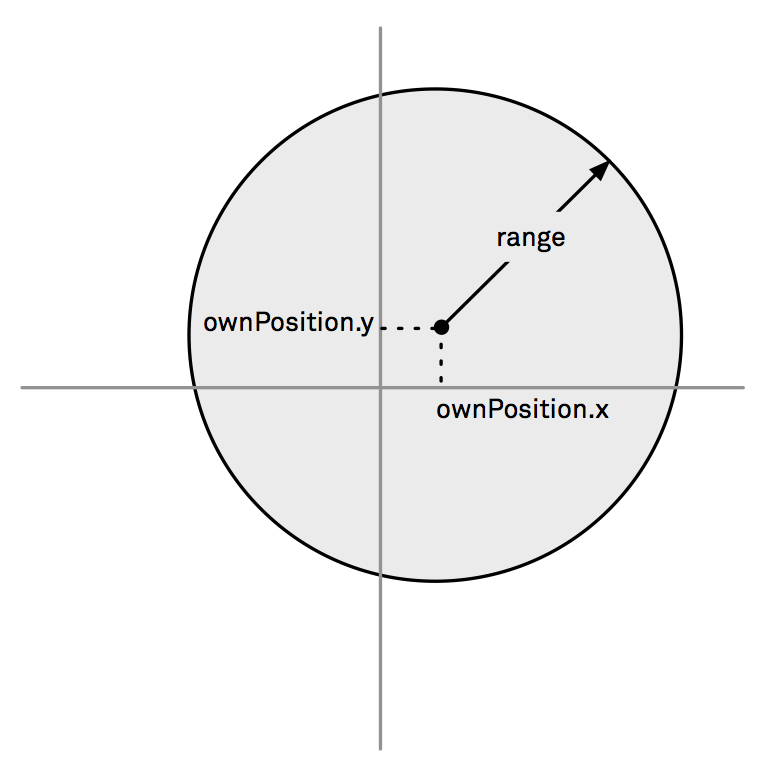
Allowing the ship to have its ownposition
Font size:
Interval:
Bookmark:
Similar books «Functional Programming in Swift»
Look at similar books to Functional Programming in Swift. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Functional Programming in Swift and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.