Pavel Yosifovich - Windows 10 System Programming, Part 1
Here you can read online Pavel Yosifovich - Windows 10 System Programming, Part 1 full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2019, publisher: leanpub.com, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
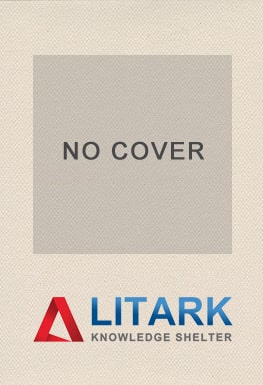
- Book:Windows 10 System Programming, Part 1
- Author:
- Publisher:leanpub.com
- Genre:
- Year:2019
- Rating:3 / 5
- Favourites:Add to favourites
- Your mark:
- 60
- 1
- 2
- 3
- 4
- 5
Windows 10 System Programming, Part 1: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Windows 10 System Programming, Part 1" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Windows 10 System Programming, Part 1 — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Windows 10 System Programming, Part 1" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
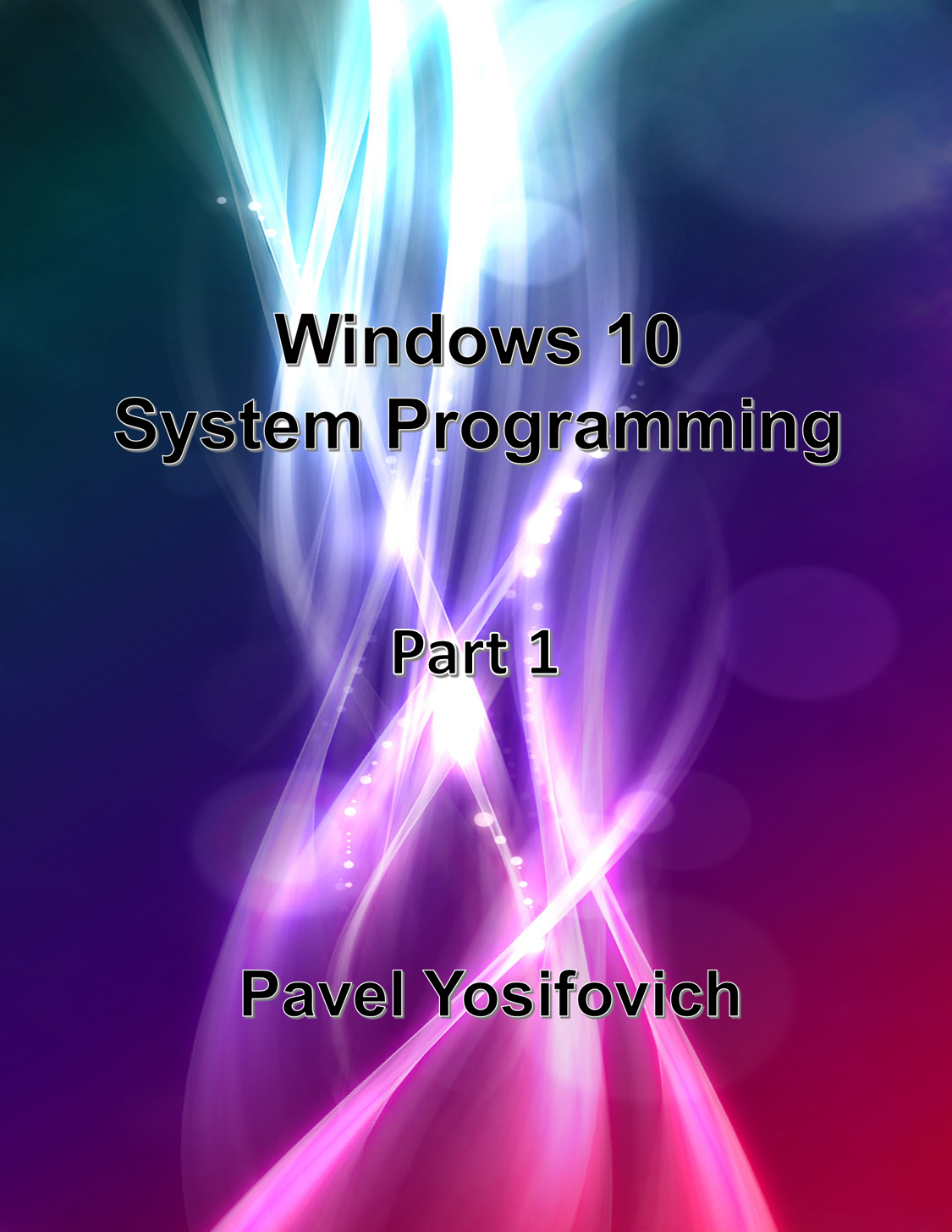
This book is for sale at http://leanpub.com/windows10systemprogramming
This version was published on 2020-08-09
* * * * *
This is a Leanpub book. Leanpub empowers authors and publishers with the Lean Publishing process. Lean Publishing is the act of publishing an in-progress ebook using lightweight tools and many iterations to get reader feedback, pivot until you have the right book and build traction once you do.
* * * * *
The term System Programming refers to programming close to an operating system level. Windows 10 System Programming provides guidance for system programmers targetting modern Windows systems, from Windows 7 up to the latest Windows 10 versions.
The book uses the documented Windows Application Programming Interface (API) to leverage system-level facilities, including processes, threads, synchronization primitives, virtual memory and I/O. The book is presented in two parts, due to the sheer size of the Windows API and the Windows system facilities breadth. Youre holding in your hands (or your screen of choice) part 1.
The book is intended for software developers that target the Windows platform, and need to have a level of control not achievable by higher-level frameworks and libraries. The book uses C and C++ for code examples, as the Windows API is mostly C-based. C++ is used where it makes sense, where its advantages are obvious in terms of maintenance, clarity, resource management, and any combination of the above. The book does not use non-trivial C++ constructs, such as template metaprogramming. The book is not about C++, its about Windows.
That said, other languages can be used to target the Windows API through their specialized interoperability mechanisms. For example, .NET languages (C#, VB, F#, etc.) can use Platform Invoke (P/Invoke) to make calls to the Windows API. Other languages, such as Python, Rust, Java, and many others have their own equivalent facilities.
Readers should be very comfortable with the C programming language, especially with pointers, structures, and its standard library, as these occur very frequently in the Windows APIs. Basic C++ knowledge is highly recommended, although it is possible to traverse the book with C proficiency only.
All the sample code from the book is freely available in the books Github repository at https://github.com/zodiacon/Win10SysProgBookSamples. Updates to the code samples will be pushed to this repository. Its recommended the reader clone the repository to the local machine, so its easy to experiment with the code directly.
All code samples have been compiled with Visual Studio 2019. Its possible to compile most code samples with earlier versions of Visual Studio if desired. There might be few features of the latest C++ standards that may not be supported in earlier versions, but these should be easy to fix.
Happy reading!
Pavel Yosifovich
June 2019
The Windows NT line of operating systems has quite a bit of history, starting with version 3.1 launched in 1993. Todays Windows 10 is the latest successor to that initial NT 3.1. The fundamental concepts of current Windows systems is essentially the same as it was back in 1993. This shows the strength of the initial OS design. That said, Windows grew significantly since its inception, with many new features and enhancements to existing ones.
This book is about system programming, typically regarded as low-level programming of the operating systems core services, without which no significant work can be accomplished. System programming uses low level APIs to use and manipulate core objects and mechanisms in Windows, such as processes, threads and memory.
In this chapter, well take a look at the foundations of Windows system programming, starting from the core concepts and APIs.
In this chapter:
- Windows Architecture Overview
- Windows Application Development
- Working with Strings
- 32-bit vs. 64-bit Development
- Coding Conventions
- Handling API Errors
- The Windows Version
Well start with a brief description of some core concepts and components in Windows. These will be elaborated on in the relevant chapters that follow.
A process is a containment and management object that represents a running instance of a program. The term process runs which is used fairly often, is inaccurate. Processes dont run - processes manage. Threads are the ones that execute code and technically run. From a high-level perspective, a process owns the following:
- An executable program, which contains the initial code and data used to execute code within the process.
- A private virtual address space, used for allocating memory for whatever purposes the code within the process needs it.
- An access token (sometimes referred to as primary token), which is an object that stores the default security context of the process, used by threads executing code within the process (unless a thread assumes a different token by using impersonation).
- A private handle table to Executive (kernel) objects, such as events, semaphores, and files.
- One or more threads of execution. A normal user-mode process is created with one thread (executing the main entry point for the process). A user-mode process without threads is mostly useless and under normal circumstances will be destroyed by the kernel.
These elements of a process are depicted in figure 1-1.
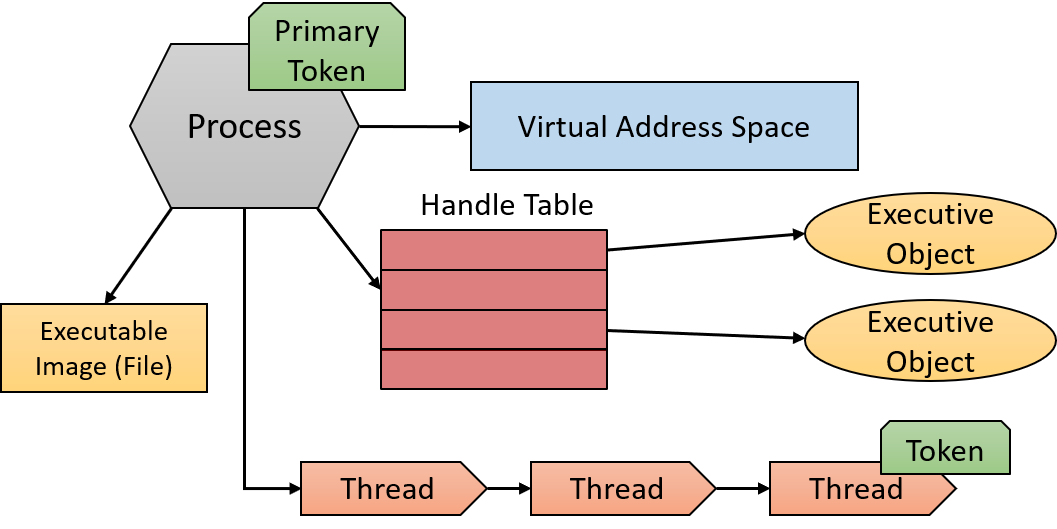
A process is uniquely identified by its Process ID, which remains unique as long as the kernel process object exists. Once its destroyed, the same ID may be reused for new processes. Its important to realize that the executable file itself is not a unique identifier of a process. For example, there may be five instances of notepad.exe running at the same time. Each process has its own address space, its own threads, its own handle table, its own unique process ID, etc. All those five processes are using the same image file (notepad.exe
) as their initial code and data. Figure 1-2 shows a screen shot of Task Managers Details tab showing five instances of Notepad.exe
, each with its own attributes.
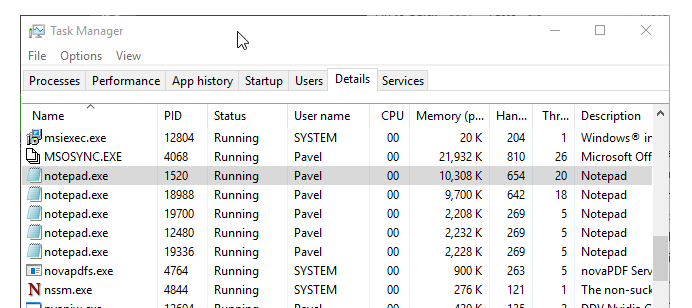
Dynamic Link Libraries (DLLs) are executable files that can contain code, data and resources (at least one of these). DLLs are loaded dynamically into a process either at process initialization time (called static linking) or later when explicitly requested (dynamic linking). Well look at DLLs in more detail in chapter 15. DLLs dont contain a standard
Font size:
Interval:
Bookmark:
Similar books «Windows 10 System Programming, Part 1»
Look at similar books to Windows 10 System Programming, Part 1. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Windows 10 System Programming, Part 1 and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.