Pavel Yosifovich - Windows Kernel Programming, Second Edition
Here you can read online Pavel Yosifovich - Windows Kernel Programming, Second Edition full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2021, publisher: leanpub.com, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
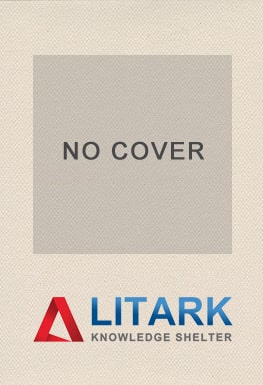
- Book:Windows Kernel Programming, Second Edition
- Author:
- Publisher:leanpub.com
- Genre:
- Year:2021
- Rating:3 / 5
- Favourites:Add to favourites
- Your mark:
- 60
- 1
- 2
- 3
- 4
- 5
Windows Kernel Programming, Second Edition: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Windows Kernel Programming, Second Edition" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Windows Kernel Programming, Second Edition — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Windows Kernel Programming, Second Edition" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
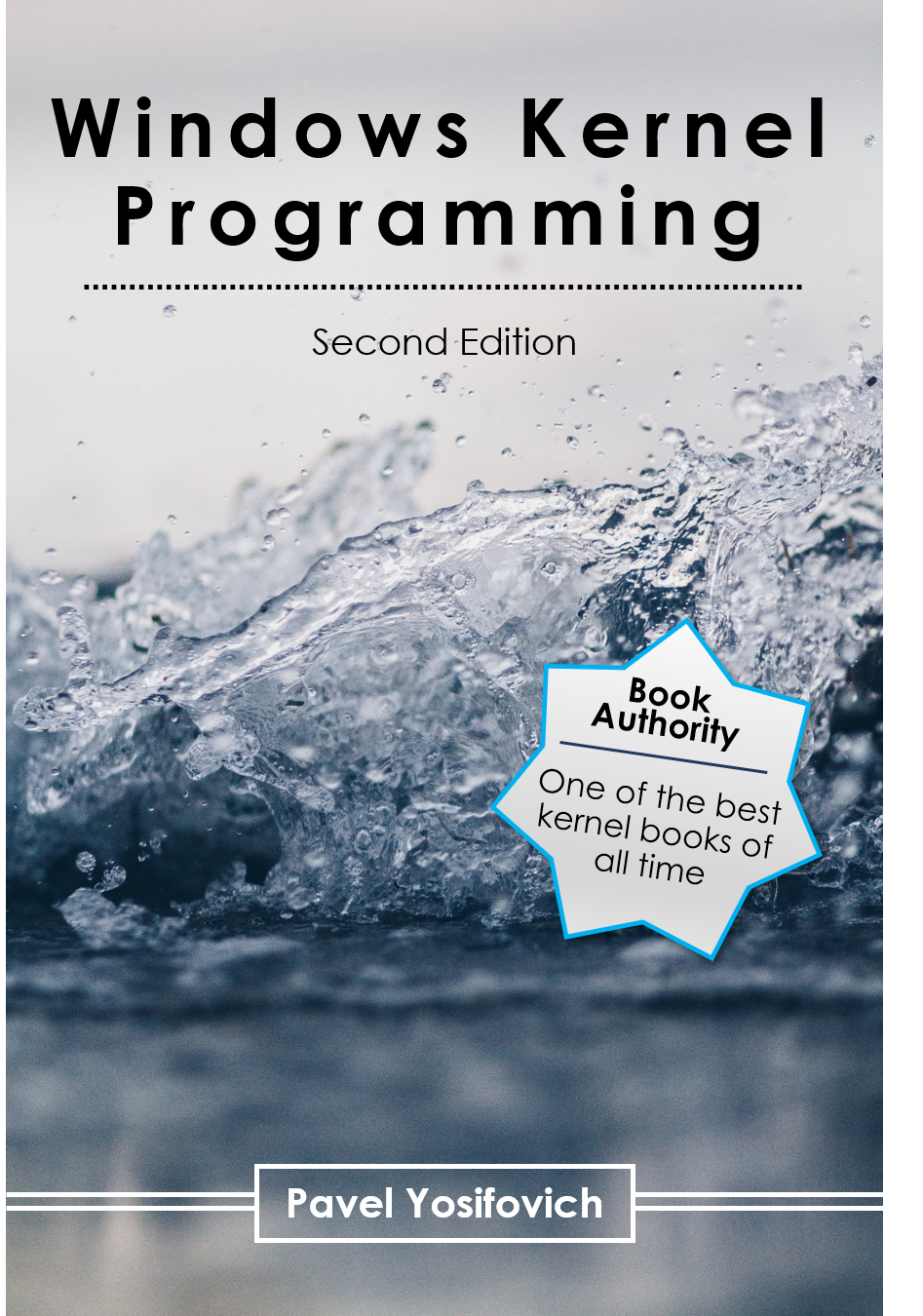
This book is for sale at http://leanpub.com/windowskernelprogrammingsecondedition
This version was published on 2022-01-22
* * * * *
This is a Leanpub book. Leanpub empowers authors and publishers with the Lean Publishing process. Lean Publishing is the act of publishing an in-progress ebook using lightweight tools and many iterations to get reader feedback, pivot until you have the right book and build traction once you do.
* * * * *
Windows kernel programming is considered by many a dark art, available to select few that manage to somehow unlock the mysteries of the Windows kernel. Kernel development, however, is no different than user-mode development, at least in general terms. In both cases, a good understanding of the platform is essential for producing high quality code.
The book is a guide to programming within the Windows kernel, using the well-known Visual Studio integrated development environment (IDE). This environment is familiar to many developers in the Microsoft space, so that the learning curve is restricted to kernel understanding, coding and debugging, with less friction from the development tools.
The book targets software device drivers, a term I use to refer to drivers that do not deal with hardware. Software kernel drivers have full access to the kernel, allowing these to perform any operation allowed by the kernel. Some software drivers are more specific, such as file system mini filters, also described in the book.
The book is intended for software developers that target the Windows kernel, and need to write kernel drivers to achieve their goals. Common scenarios where kernel drivers are employed are in the Cyber Security space, where kernel drivers are the chief mechanism to get notified of important events, with the power to intercept certain operations. The book uses C and C++ for code examples, as the kernel API is all C. C++ is used where it makes sense, where its advantages are obvious in terms of maintenance, clarity, resource management, or any combination of these. The book does not use complex C++ constructs, such as template metaprogramming. The book is not about C++, its about Windows kernel drivers.
Readers should be very comfortable with the C programming language, especially with pointers, structures, and its standard library, as these occur very frequently when working with kernel APIs. Basic C++ knowledge is highly recommended, although it is possible to traverse the book with C proficiency only.
Here is a quick rundown of the chapters in the book:
- Chapter 1 (Windows Internals Overview) provides the fundamentals of the internal workings of the Windows OS at a high level, enough to get the fundamentals without being bogged down by too many details.
- Chapter 2 (Getting Started with Kernel Development) describes the tools and procedures needed to set up a development environment for developing kernel drivers. A very simple driver is created to make sure all the tools and procedures are working correctly.
- Chapter 3 (Kernel Programming Basics) looks at the fundamentals of writing drivers, including bssic kernel APIs, handling of common programming tasks involving strings, linked lists, dynamic memory allocations, and more.
- Chapter 4 (Driver from Start to Finish) shows how to build a complete driver that performs some useful functionality, along with a client application to drive it.
If you are new to Windows kernel development, you should read chapters 1 to 7 in order. Chapter 8 contains some advanced material you may want to go back to after you have built a few simple drivers. Chapters 9 onward describe specialized techniques, and in theory at least, can be read in any order.
All the sample code from the book is freely available on the books Github repository at https://github.com/zodiacon/windowskernelprogrammingbook2e. Updates to the code samples will be pushed to this repository. Its recommended the reader clone the repository to the local machine, so its easy to experiment with the code directly.
All code samples have been compiled with Visual Studio 2019. Its possible to compile most code samples with earlier versions of Visual Studio if desired. There might be few features of the latest C++ standards that may not be supported in earlier versions, but these should be easy to fix.
Happy reading!
Pavel Yosifovich
June 2022
This chapter describes the most important concepts in the internal workings of Windows. Some of the topics will be described in greater detail later in the book, where its closely related to the topic at hand. Make sure you understand the concepts in this chapter, as these make the foundations upon any driver and even user mode low-level code, is built.
In this chapter:
- Processes
- Virtual Memory
- Threads
- System Services
- System Architecture
- Handles and Objects
A process is a containment and management object that represents a running instance of a program. The term process runs which is used fairly often, is inaccurate. Processes dont run processes manage. Threads are the ones that execute code and technically run. From a high-level perspective, a process owns the following:
- An executable program, which contains the initial code and data used to execute code within the process. This is true for most processes, but some special ones dont have an executable image (created directly by the kernel).
- A private virtual address space, used for allocating memory for whatever purposes the code within the process needs it.
- An access token (called primary token), which is an object that stores the security context of the process, used by threads executing in the process (unless a thread assumes a different token by using impersonation).
- A private handle table to executive objects, such as events, semaphores, and files.
- One or more threads of execution. A normal user-mode process is created with one thread (executing the classic
main
/WinMain
function). A user mode process without threads is mostly useless, and under normal circumstances will be destroyed by the kernel.
These elements of a process are depicted in figure 1-1.
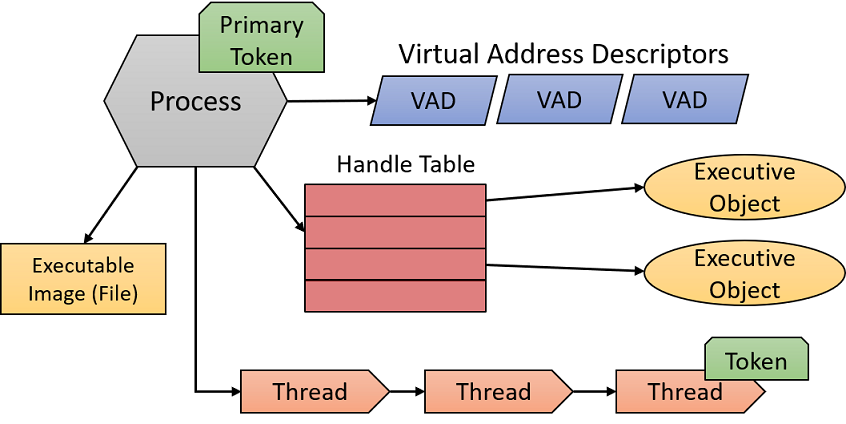
A process is uniquely identified by its Process ID, which remains unique as long as the kernel process object exists. Once its destroyed, the same ID may be reused for new processes. Its important to realize that the executable file itself is not a unique identifier of a process. For example, there may be five instances of notepad.exe running at the same time. Each of these Notepad instances has its own address space, threads, handle table, process ID, etc. All those five processes are using the same image file (
Font size:
Interval:
Bookmark:
Similar books «Windows Kernel Programming, Second Edition»
Look at similar books to Windows Kernel Programming, Second Edition. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Windows Kernel Programming, Second Edition and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.