75 Python Object Oriented Programming Exercises Volume 1 75 Python Object Oriented Programming Exercises Volume 1 Edcorner Learning
Table of Contents
Introduction
Python is a general-purpose interpreted, interactive, object- oriented, and a powerful programming language with dynamic semantics. It is an easy language to learn and become expert. Python is one among those rare languages that would claim to be both easy and powerful. Python's elegant syntax and dynamic typing alongside its interpreted nature makes it an ideal language for scripting and robust application development in many areas on giant platforms. Python helps with the modules and packages, which inspires program modularity and code reuse. The Python interpreter and thus the extensive standard library are all available in source or binary form for free of charge for all critical platforms and can be freely distributed.
Learning Python doesn't require any pre- requisites. However, one should have the elemental understanding of programming languages. This Book consist of 75 python Object Oriented Programming coding exercises to practice different topics. In each exercise we have given the exercise coding statement you need to complete and verify your answers. We also attached our own input output screen of each exercise and their solutions. Learners can use their own python compiler in their system or can use any online compilers available.
We have covered all level of exercises in this book to give all the learners a good and efficient Learning method to do hands on python different scenarios.
Module 1 Local Enclosed Global Built-In Rules
1. The stock_info( ) function is defined. Using the appropriate attribute of the stock_info( ) function, display the names of all arguments to this function to the console. An example of calling the function: print(stock_info('ABC', 'USA', 115, '$')) Company: ABC Country: USA Price: $ 115 Tip: Use the code attribute of the function. Expected result: ('company', 'country', 'price', 'currency') def stock_info(company, country, price, currency): return f'Company: {company}\nCountry: {country}\nPrice: {currency} {price}'
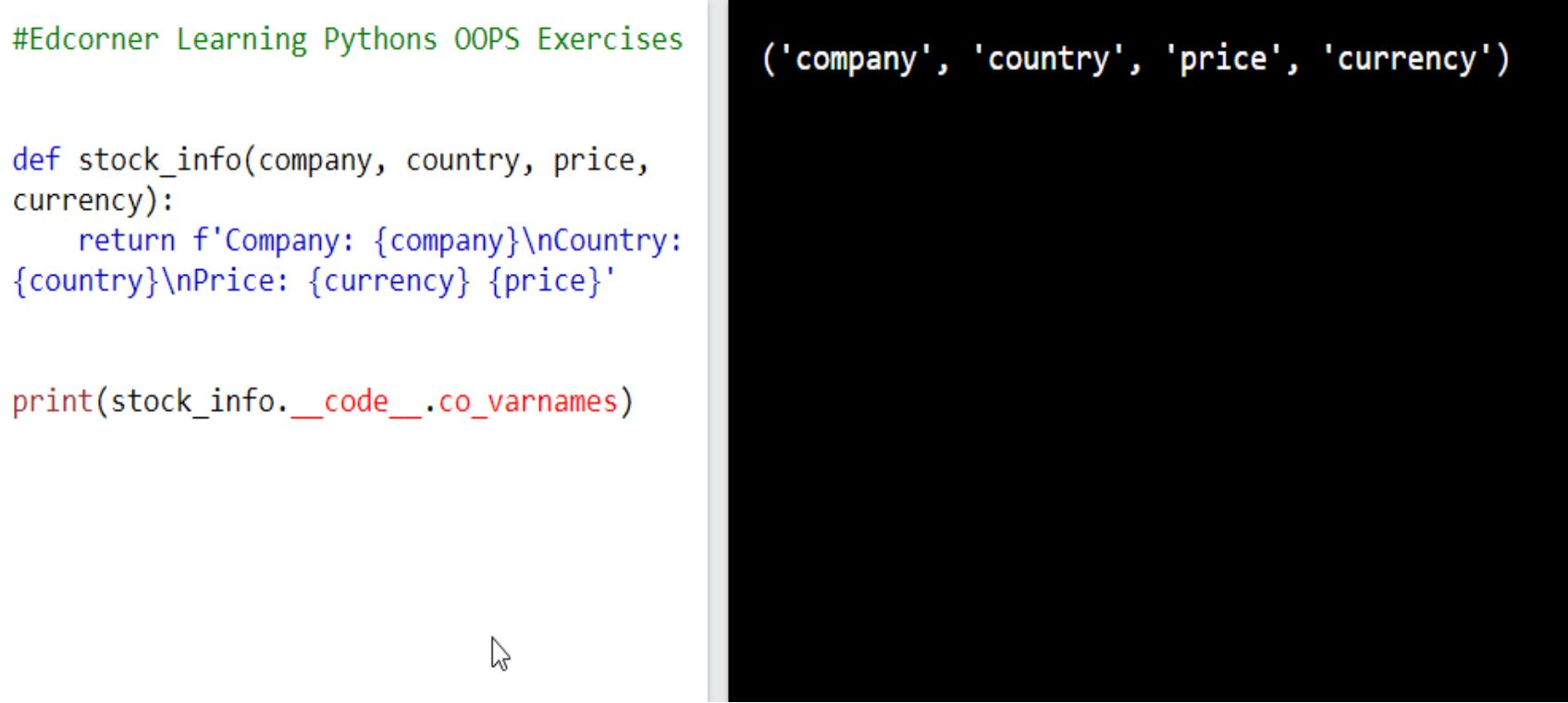
2.
Using the built-ins module import the sum( ) function. Then display its documentation of this function. Call the function on the list below and print the result to the console. [-4, 3, 2] Expected result: Help on built-in function sun in nodule built-ins: sum(iterable, /, start=0) Return the sun of a 'start' value (default: 0) plus an iterable of numbers When the Iterable is empty, return the start value. This function is intended specifically for use with numeric values and may reject non-numeric types. Solution: import builtins help(builtins.sum) print(builtins.sum([-4, 3, 2])) 3.
A global variable counter is given with an incorrectly implemented update_counter() function. Correct the implementation of the update_counter ( ) function so that you can modify the counter variable from this function. Then call the update_counter ( ) function. Tip: Use the global statement. Expected result: counter = 1 def update_counter(): counter += 1
print(counter) 4. The following global variables are given: counter dot_counter and incorrectly implemented update_counters ( ) function.
Correct the implementation of the update_counters ( ) function so that you can modify the values of the given global variables from this function. Then call update_counters() 40 times. In response, print the value of the counter and dot_counter global variables to the console as shown below. Tip: Use the global statement. Expected result: ........................................ counter = 0 dot_counter = '' def update_counter(): counter += 1 dot_counter += '.' Solution:
5.
A display_info() function was implemented. This function has an incorrectly implemented internal update_counter( ) function. Correct the implementation of this function so that you can modify non-local variables: counter and dot_counter from the internal function update_counter() . In response, call dispiay_info( ) with the number_of_updates argument set to 10. Tip: Use the nonlocal statement. def display_info(number_of_updates=1): counter = 100 dot_counter = '' def update_counter(): counter += 1 dot_counter += '.' [update_counter() for _ in range(number_of_updates)] print(counter) print(dot_counter) Solution: 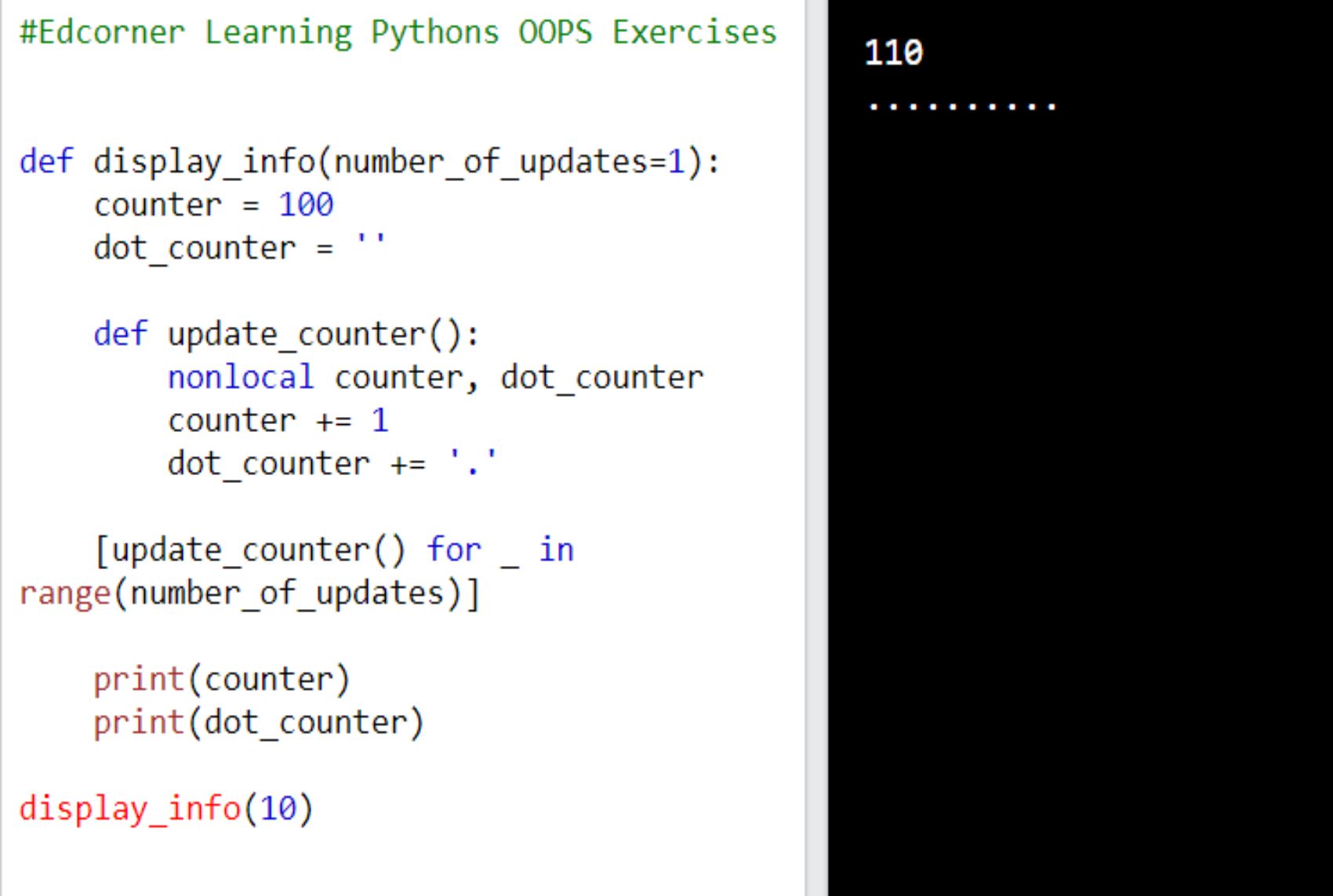
Module 2 Namespaces and Scopes
6. def display_info(number_of_updates=1): counter = 100 dot_counter = '' def update_counter(): counter += 1 dot_counter += '.' [update_counter() for _ in range(number_of_updates)] print(counter) print(dot_counter) Solution:
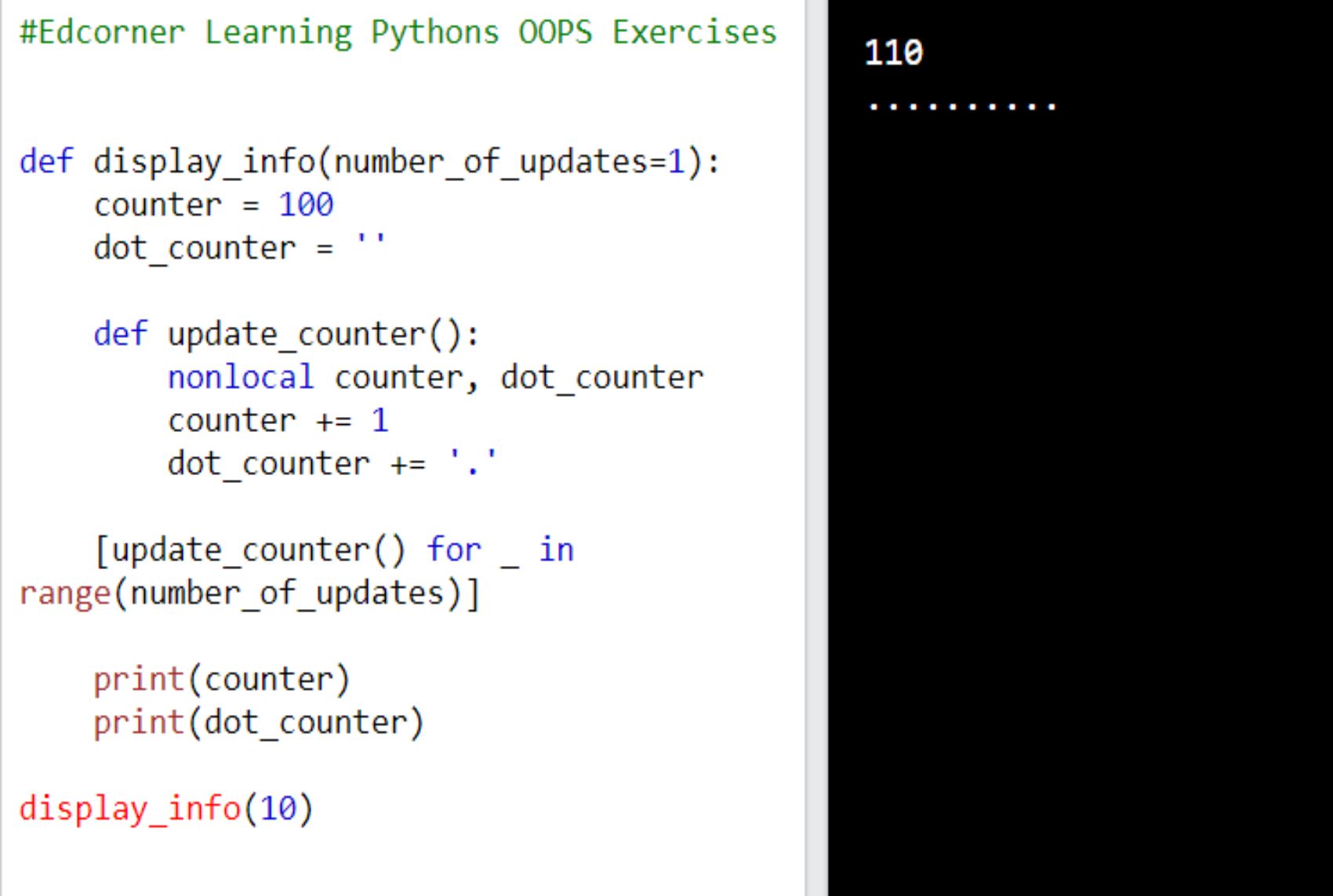
Module 2 Namespaces and Scopes
6.
Import the built-in datetime module and display the namespace of this module (sorted alphabetically) as given below. Tip: Use the _dict_ attribute of the datetime module. Expected result: MAXYEAR MINYEAR _builtlns_ _cached_ _doc_ _file_ _loader_ _name_ _package_ _spec_ date datetime datetime_CAPI sys time timedelta timezone tzinfo Solution:
7. The Product class is given below. Display the namespace (value of the _dict_ attribute) of this class as shown below. Expected result: __module__ __init__ __repr__ get_id __dict__ __weakref__ __doc__ import uuid class Product: def __init__(self, product_name, price): self.product_id = self.get_id() self.product_name = product_name self.price = price def __repr__(self): return f"Product(product_name='{self.product_name}', price={self.price})" @staticmethod def get_id(): return str(uuid.uuid4().fields[-1])[:6]
8.
The Product class is specified. An instance of this class named product was created. Display the namespace (value of the _dict_ attribute) of this instance as shown below. Expected result: {'product_name': 'Mobile Phone1, 'product_id': '54274', 'price': 2900} import uuid class Product: def __init__(self, product_name, product_id, price): self.product_name = product_name self.product_id = product_id self.price = price def __repr__(self): return f"Product(product_name='{self.product_name}', price={self.price})" product = Product('Mobile Phone', '54274', 2900) Solution: 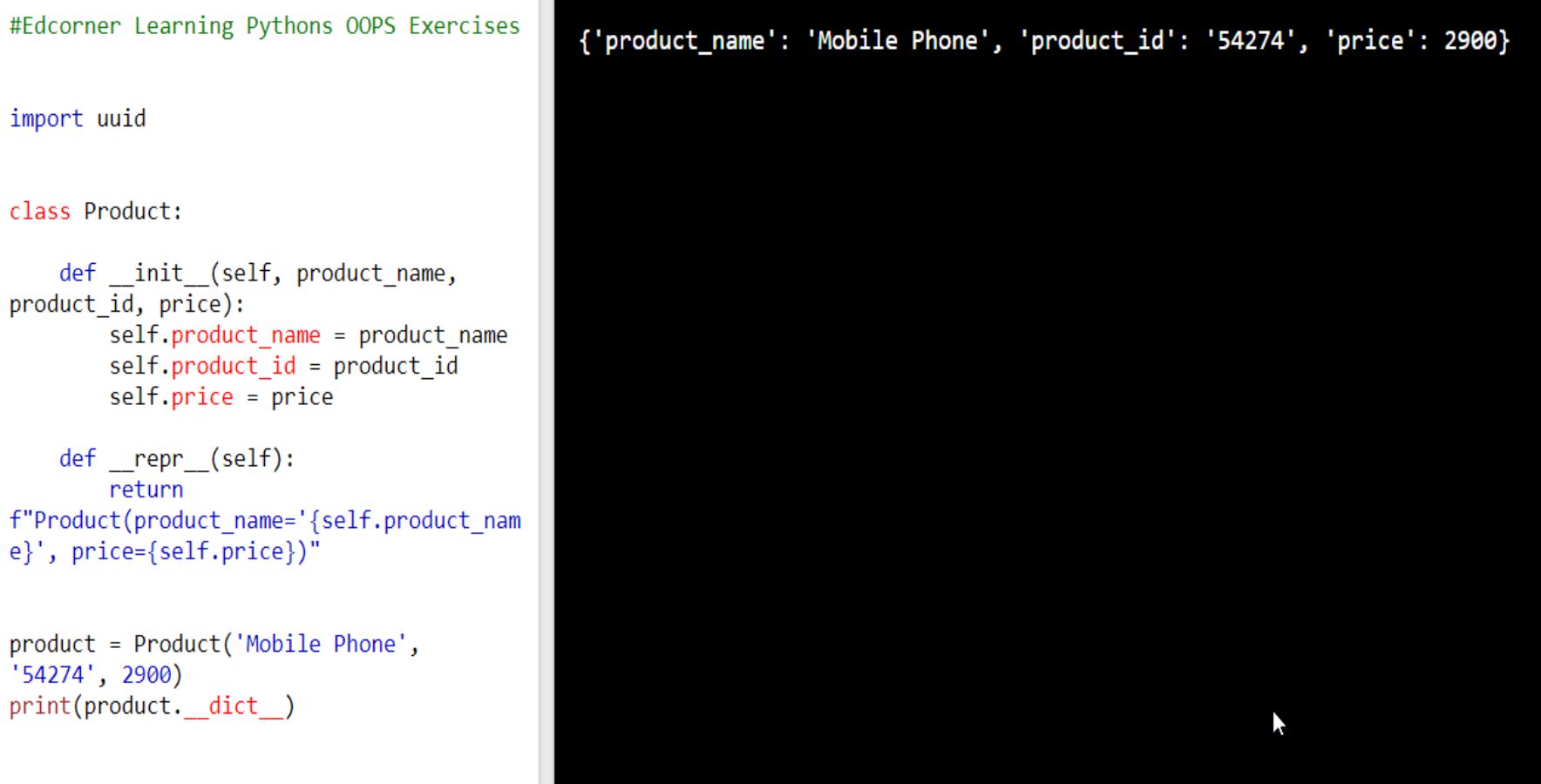
Module 3 Args and Kwargs
9. Implement a function called stick( ) that takes any number of bare arguments and return an object of type str being a concatenation of all arguments of type str passed to the function with the '#' sign (see below). Example: [IN]: stick('sport', 'summer', 4, True) [OUT]: 'sport#summer As an answer call the stick( ) function in the following ways (print the result to the console): stick('sport', 'summer') stick(3, 5, 7) stick(False, 'time'.
True, 'workout', [], 'gym') Expected result: Sport#sumer time#workout#gym Solution: 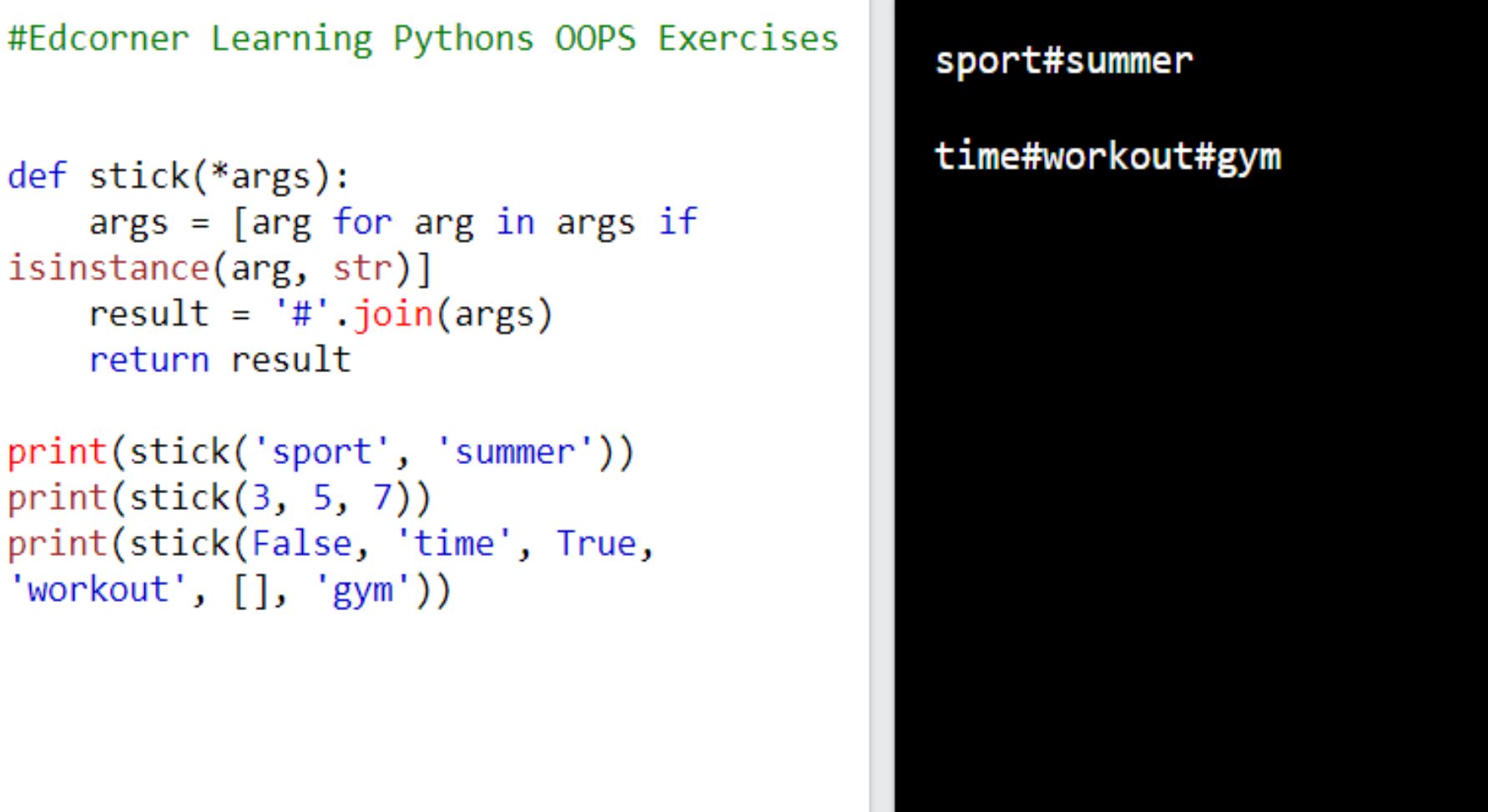
Next page