Kyle Loudon - C++ Pocket Reference
Here you can read online Kyle Loudon - C++ Pocket Reference full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2008, publisher: OReilly Media, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
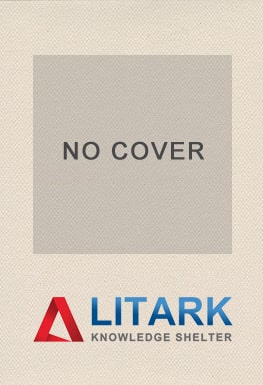
- Book:C++ Pocket Reference
- Author:
- Publisher:OReilly Media
- Genre:
- Year:2008
- Rating:3 / 5
- Favourites:Add to favourites
- Your mark:
C++ Pocket Reference: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "C++ Pocket Reference" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
C++ is a complex language with many subtle facets. This is especially true when it comes to object-oriented and template programming. The C++ Pocket Reference is a memory aid for C++ programmers, enabling them to quickly look up usage and syntax for unfamiliar and infrequently used aspects of the language. The books small size makes it easy to carry about, ensuring that it will always be at-hand when needed. Programmers will also appreciate the books brevity; as much information as possible has been crammed into its small pages.
In the C++ Pocket Reference, you will find:
- Information on C++ types and type conversions
- Syntax for C++ statements and preprocessor directives
- Help declaring and defining classes, and managing inheritance
- Information on declarations, storage classes, arrays, pointers, strings, and expressions
- Refreshers on key concepts of C++ such as namespaces and scope
- More!
Together with its companion STL Pocket Reference, the C++ Pocket Reference forms one of the most concise, easily-carried, quick-references to the C++ language available.
Kyle Loudon: author's other books
Who wrote C++ Pocket Reference? Find out the surname, the name of the author of the book and a list of all author's works by series.