This is a Leanpub book. Leanpub empowers authors and publishers with the Lean Publishing process. Lean Publishing is the act of publishing an in-progress ebook using lightweight tools and many iterations to get reader feedback, pivot until you have the right book and build traction once you do.
Chapter One: Getting Started
In this book we will learn TypeScript, which is a superset of JavaScript. It was built by Microsoft to fix the issues of loose binding in JavaScript.
After learing about TypeScript, we will learn to use it in projects. We will create the below projects
- TypeScript Project
- ReactJS with TypeScript Project
- NodeJS with TypeScript Project
- React Redux TypeScript Project
Now, with TypeScript we add all new features in JavaScript, through which we can avoid type error even before they occur. But the problem with TypeScript is that, Browser cant execute it.
The browser understands only JavaScript, so the TypeScript needs to be compiled to JavaScript.
Project Setup
We will open a new folder in VS Code and create a basic index.html file in it. Here, we are also refering to a JavaScript file of main.js
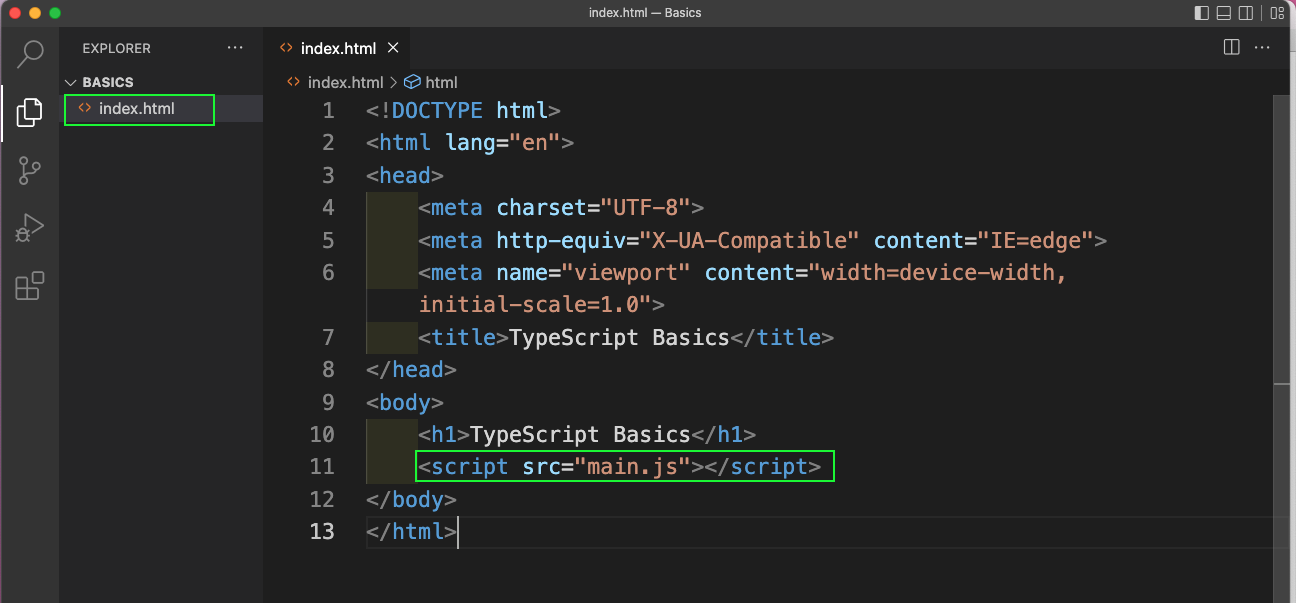
index.html
Next, we will install TypeScript globally in our system with the below command. Please add a sudo if you are using a Mac or linux system.
1
npm i -g typescript
Now, create a file main.ts and add a simple console.log in it. The browser only understands JavaScript, so we will change it to JavaScript with the command tsc main.ts. This command will create a new main.js JavaScript file.

main.ts
We will also be using the awesome extension of Live Server in our project. So, that we dont have to re-run the project after every changes.
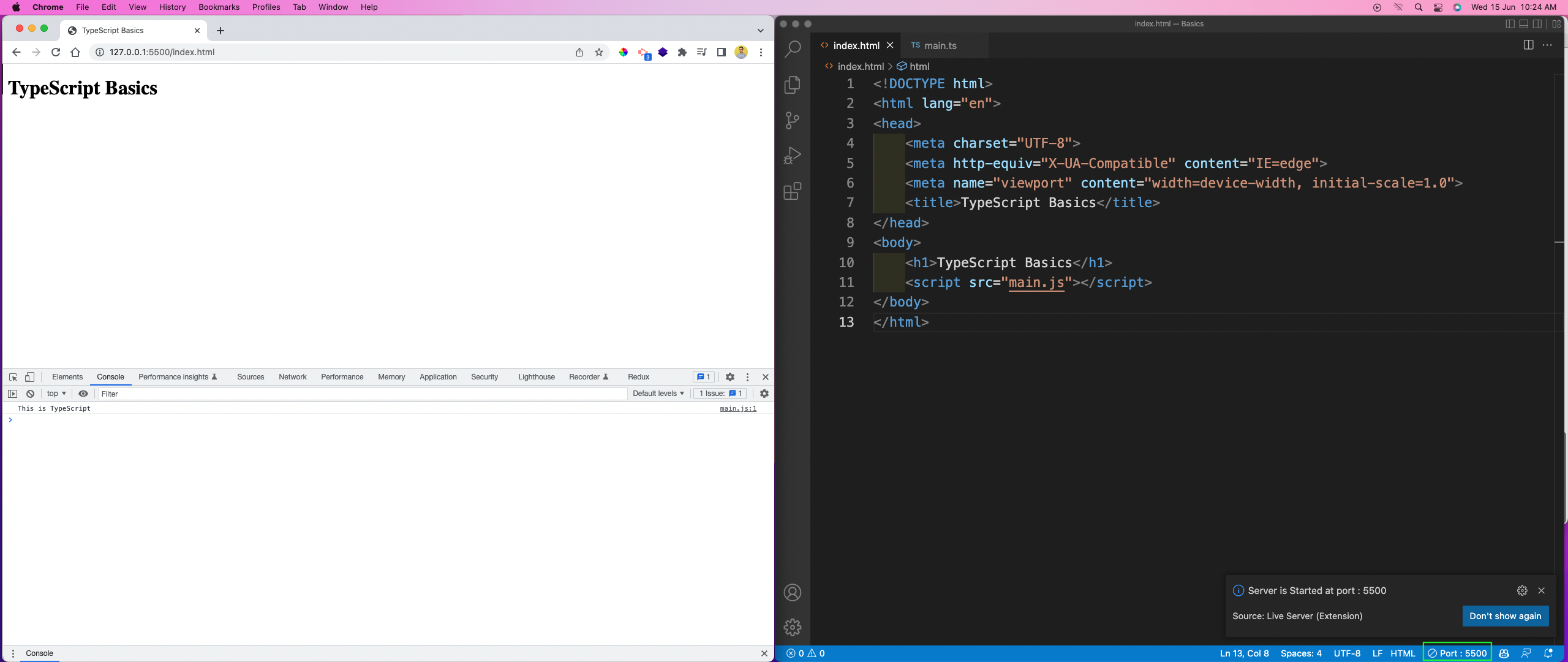
Live Server
Chapter Two - TypeScript Basics
Create a file index.ts in our earlier setup.
Number Type
We will write the below code in it. Here, we have two variables one is myNum and other is anotherNum. We have given anotherNum a type of number.
Now, for both myNum and anotherNum, we cannot take anything else then number.
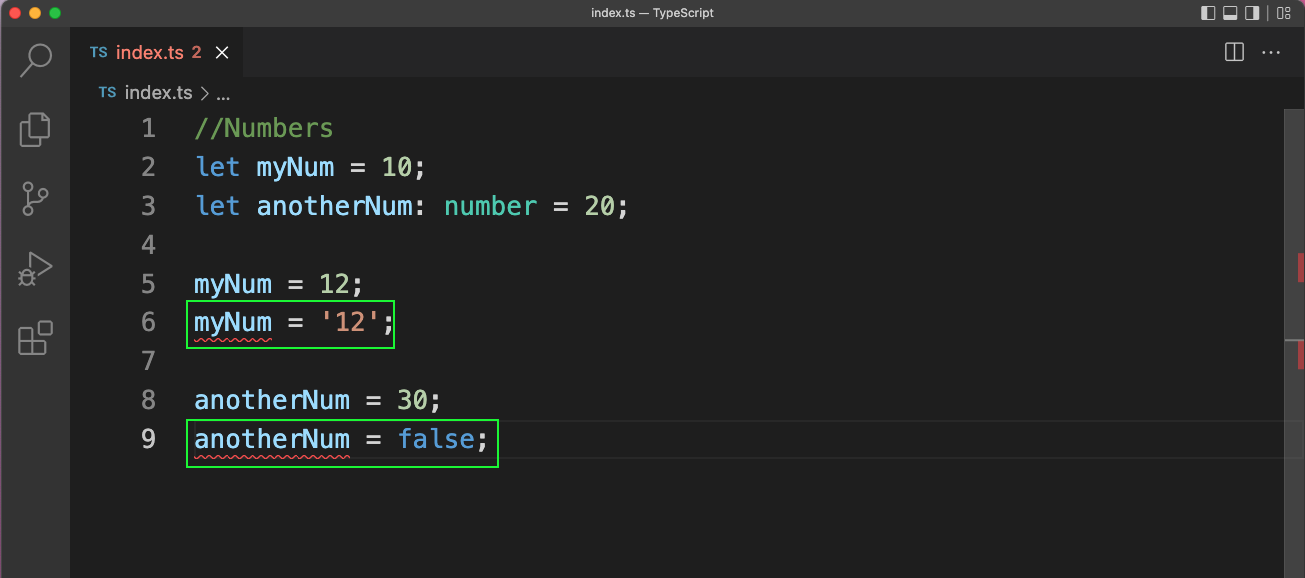
index.ts
If we hover our mouse over the error, we can see the real issue.
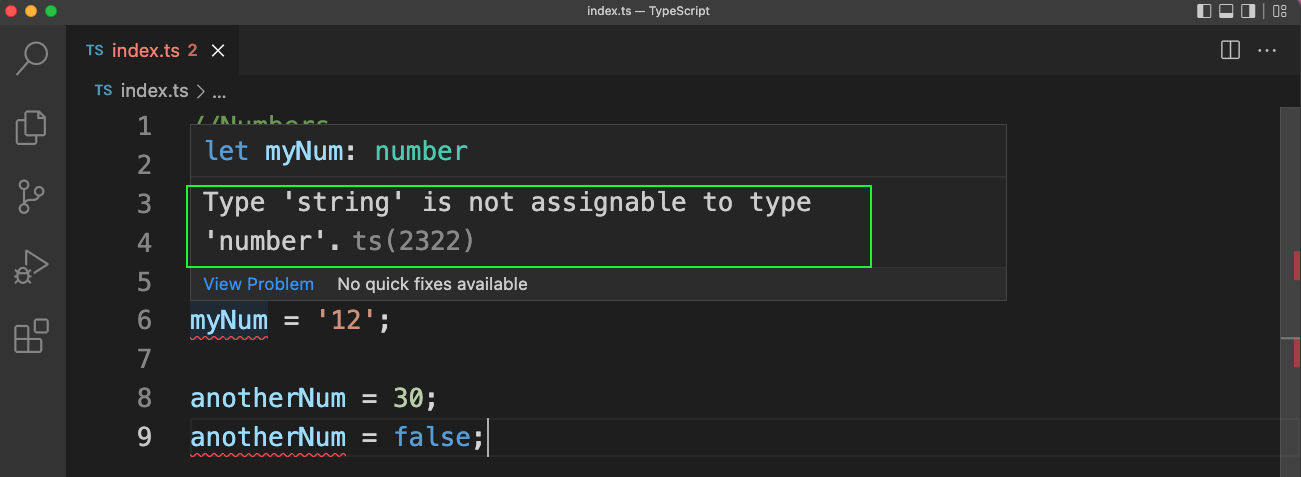
Number Issue
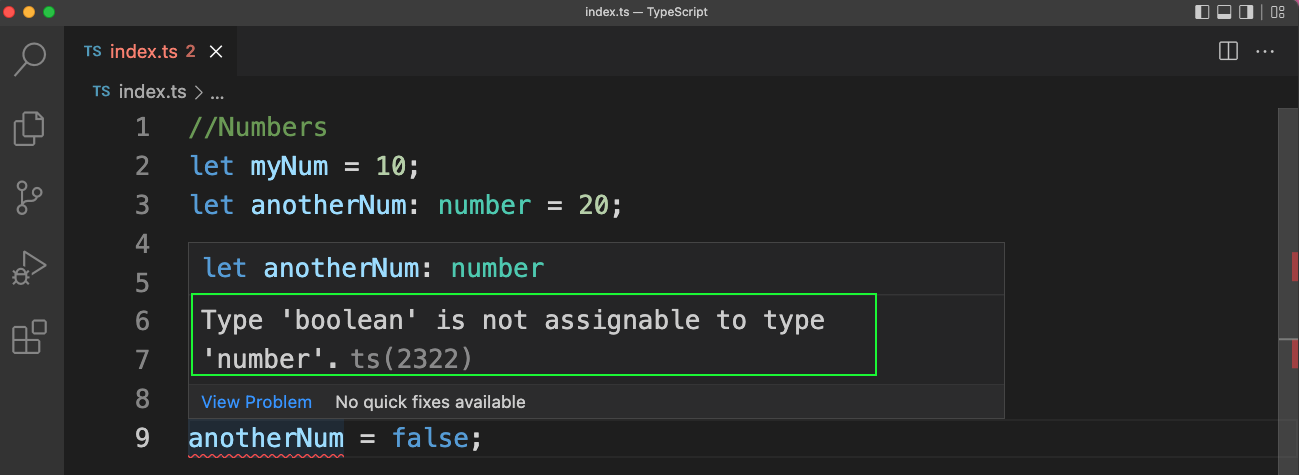
Boolean Issue
As in the above example, even if we dont assign a type, then also TypeScript assigns a type. Hover the mouse over myNum and it will show the type of number.

TypeScript
String & Boolean Types
The same is true for String and Boolean.
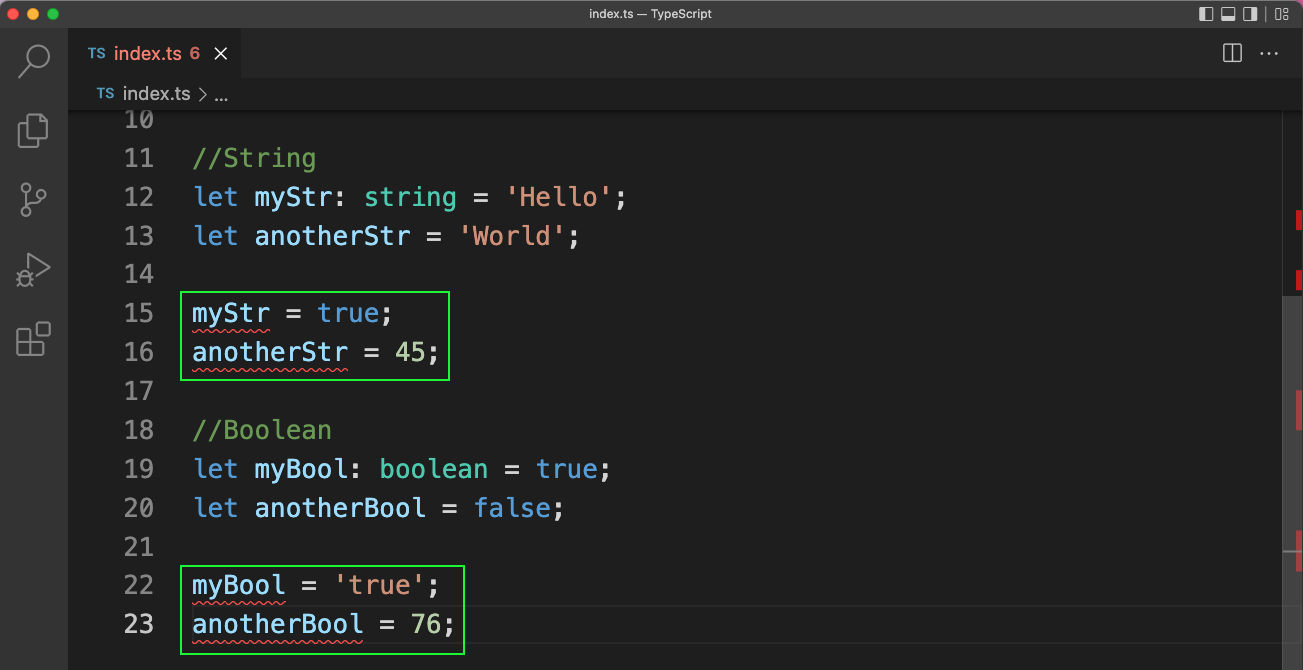
Strings
Inference
Now, the question from above is when do we want to assign a type and when TypeScript automatically assigns it.
In most cases, we should leave it to TypeScript to assign type. In the below case, we have a variable salary in which we didnt assigned a type.
Later on we are assigning a number, string and a boolean to it.
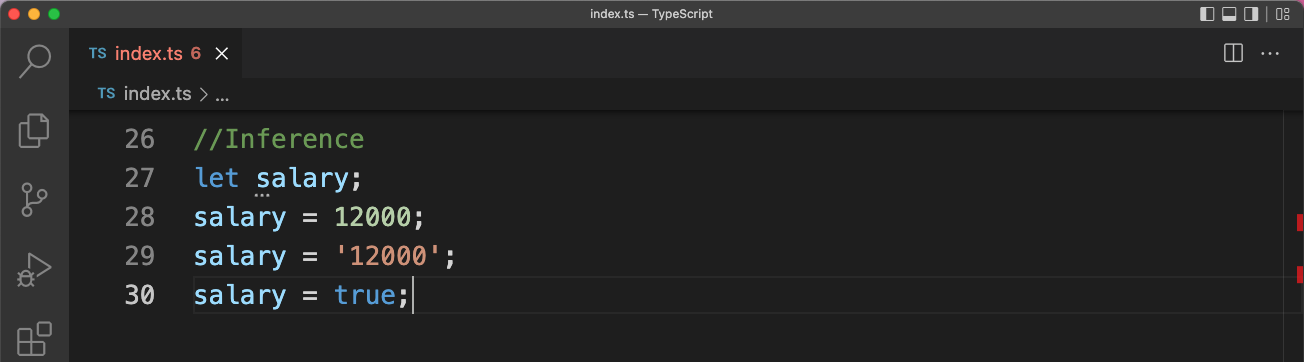
Inference
Now, this is not right and in these scenarios where we want to assign value later on, we give an explicit type.
And now we will start getting the type errors.
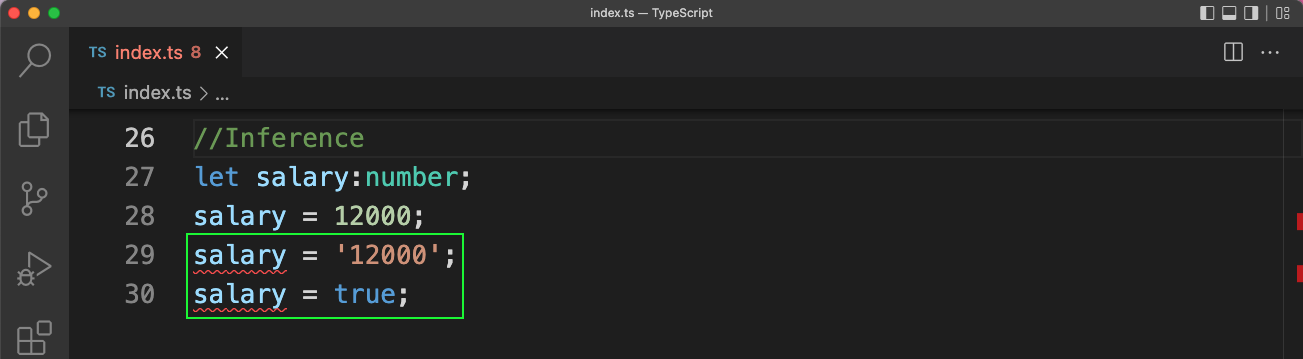
Inference2
Objects
Now, we will learn about Objects in TypeScript. In the below example, we have just given an object, which have two strings, one number and one boolean.
If we hover our mouse over the object, it will tell us the data types.
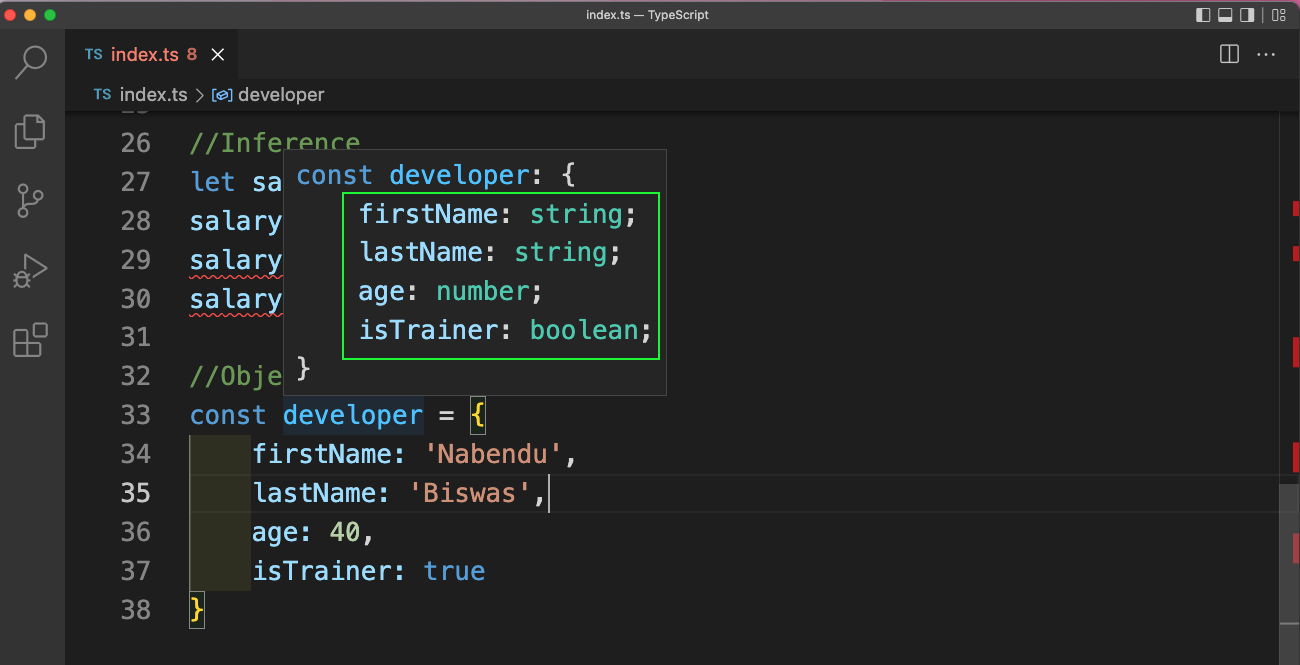
Objects
Now, we will create a new object, where we will give the type of each key. We will get error if we try to assign a different value to a key or a key, which doesnt exists.
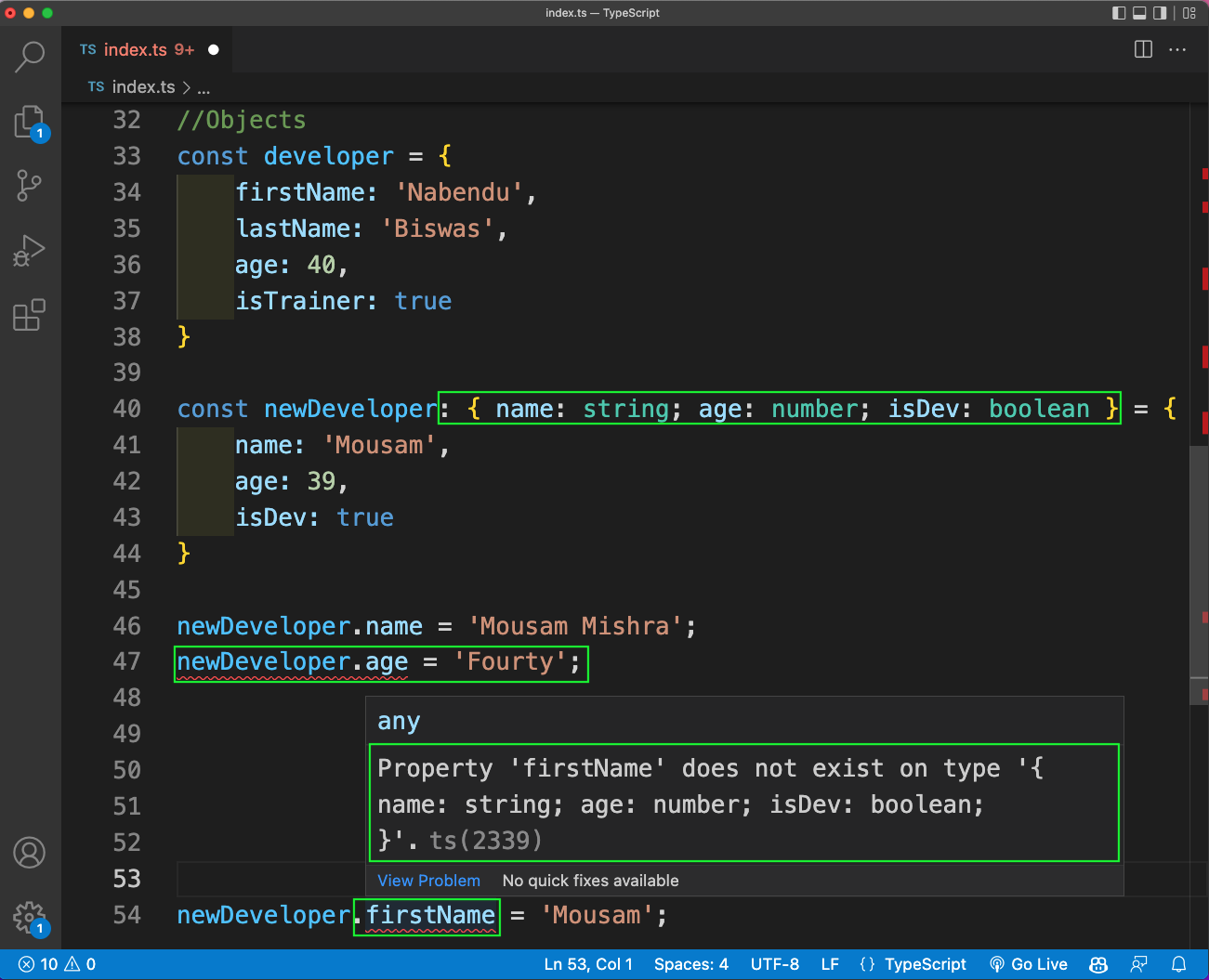
Objects2
Arrays
Now, we will look into Arrays. In typescript, if we give an array of strings like languages in below example. We cannot push a number or boolean to it.
We can also explicitly declare that a we have a array of type, like we have declared an array of type number.
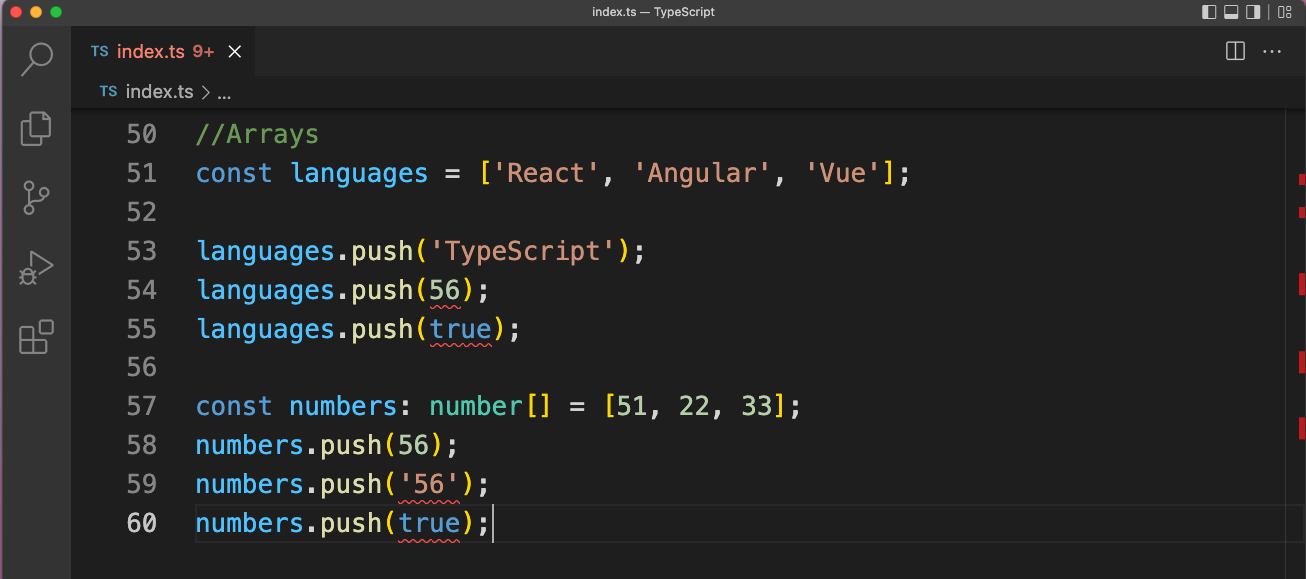
Arrays
Complex Arrays
Now, we will learn to make an array of Object. Here, we are giving the type and in the Object we are mentioning the type of keys in object.
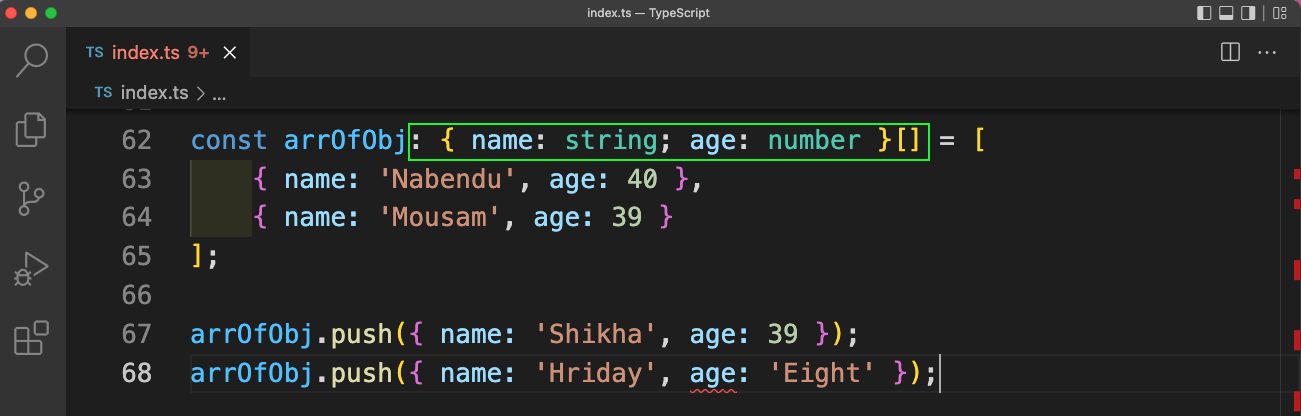
ArrayOfObjects
If we want to write the type of array of arrays, we need to use two [] in the type inference.
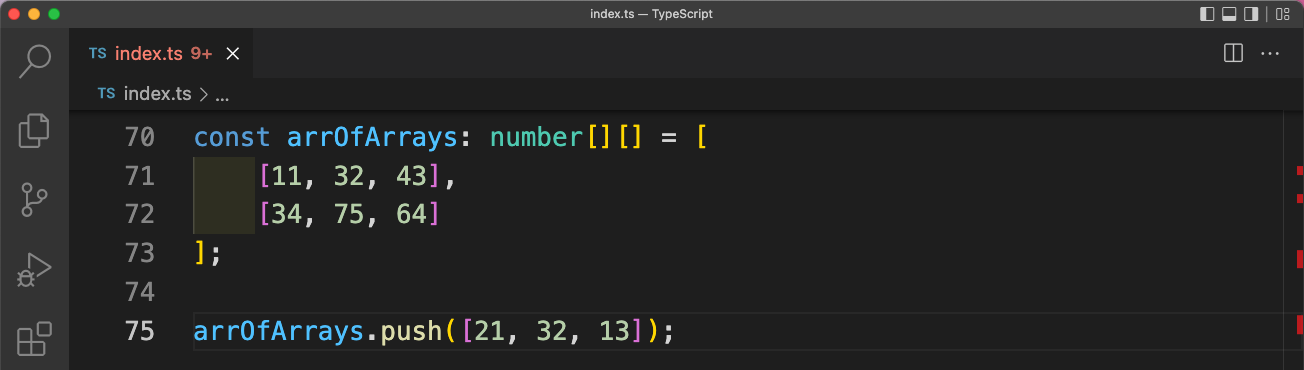
ArrayOfArrays
Functions
Now, we will look into the example of functions. Suppose, we need to add two numbers and have created a function addNums for it.
If we dont give the type, we will be not get the error even if we give one string.
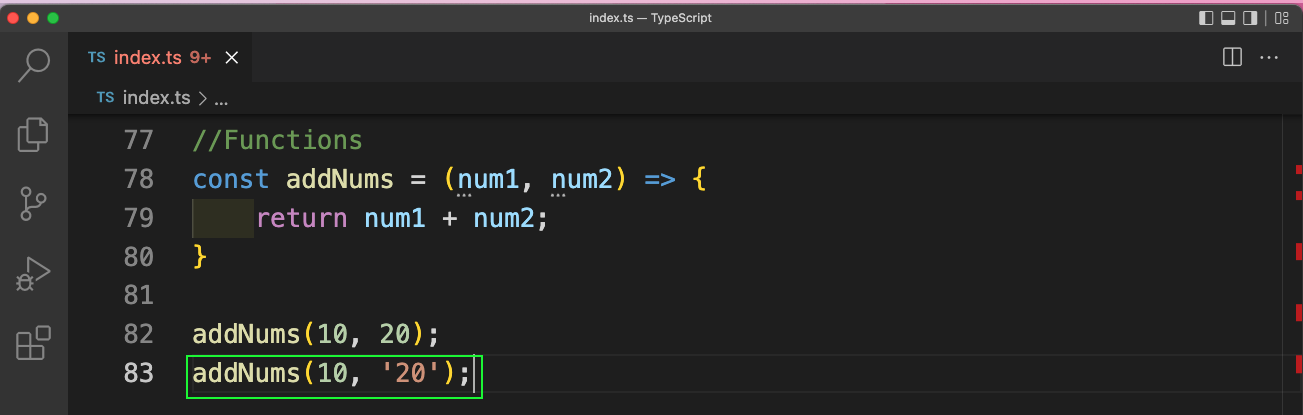
Functions