Library - Design and Analysis of Computer Algorithms
Here you can read online Library - Design and Analysis of Computer Algorithms full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2020, genre: Children. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
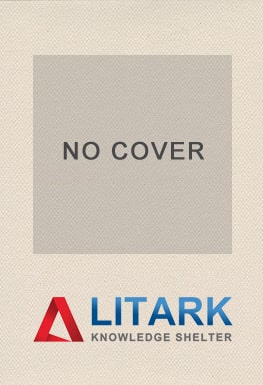
Design and Analysis of Computer Algorithms: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Design and Analysis of Computer Algorithms" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Library: author's other books
Who wrote Design and Analysis of Computer Algorithms? Find out the surname, the name of the author of the book and a list of all author's works by series.
Design and Analysis of Computer Algorithms — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Design and Analysis of Computer Algorithms" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Lecture 1: Course Introduction
Read: (All readings are from Cormen, Leiserson, Rivest and Stein, Introduction to Algorithms , 2nd Edition). Review Chapts. 15 in CLRS.
What is an algorithm? Our text defines an algorithm to be any well-defined computational procedure that takes some values as input and produces some values as output . Like a cooking recipe, an algorithm provides a step-by-step method for solving a computational problem. Unlike programs, algorithms are not dependent on a particular programming language, machine, system, or compiler. They are mathematical entities, which can be thought of as running on some sort of idealized computer with an infinite random access memory and an unlimited word size. Algorithm design is all about the mathematical theory behind the design of good programs.
Why study algorithm design? Programming is a very complex task, and there are a number of aspects of program- ming that make it so complex. The first is that most programming projects are very large, requiring the coor- dinated efforts of many people. (This is the topic a course like software engineering.) The next is that many programming projects involve storing and accessing large quantities of data efficiently. (This is the topic of courses on data structures and databases.) The last is that many programming projects involve solving complex computational problems, for which simplistic or naive solutions may not be efficient enough. The complex problems may involve numerical data (the subject of courses on numerical analysis), but often they involve discrete data. This is where the topic of algorithm design and analysis is important.
Although the algorithms discussed in this course will often represent only a tiny fraction of the code that is generated in a large software system, this small fraction may be very important for the success of the overall project. An unfortunately common approach to this problem is to first design an inefficient algorithm and data structure to solve the problem, and then take this poor design and attempt to fine-tune its performance. The problem is that if the underlying design is bad, then often no amount of fine-tuning is going to make a substantial difference.
The focus of this course is on how to design good algorithms, and how to analyze their efficiency. This is among the most basic aspects of good programming.
Course Overview: This course will consist of a number of major sections. The first will be a short review of some preliminary material, including asymptotics, summations, and recurrences and sorting. These have been covered in earlier courses, and so we will breeze through them pretty quickly. We will then discuss approaches to designing optimization algorithms, including dynamic programming and greedy algorithms. The next major focus will be on graph algorithms. This will include a review of breadth-first and depth-first search and their application in various problems related to connectivity in graphs. Next we will discuss minimum spanning trees, shortest paths, and network flows. We will briefly discuss algorithmic problems arising from geometric settings, that is, computational geometry.
Most of the emphasis of the first portion of the course will be on problems that can be solved efficiently, in the latter portion we will discuss intractability and NP-hard problems. These are problems for which no efficient solution is known. Finally, we will discuss methods to approximate NP-hard problems, and how to prove how close these approximations are to the optimal solutions.
Issues in Algorithm Design: Algorithms are mathematical objects (in contrast to the must more concrete notion of a computer program implemented in some programming language and executing on some machine). As such, we can reason about the properties of algorithms mathematically. When designing an algorithm there are two fundamental issues to be considered: correctness and efficiency.
It is important to justify an algorithms correctness mathematically. For very complex algorithms, this typically requires a careful mathematical proof, which may require the proof of many lemmas and properties of the solution, upon which the algorithm relies. For simple algorithms (BubbleSort, for example) a short intuitive explanation of the algorithms basic invariants is sufficient. (For example, in BubbleSort, the principal invariant is that on completion of the i th iteration, the last i elements are in their proper sorted positions.)
Establishing efficiency is a much more complex endeavor. Intuitively, an algorithms efficiency is a function of the amount of computational resources it requires, measured typically as execution time and the amount of space, or memory, that the algorithm uses. The amount of computational resources can be a complex function of the size and structure of the input set. In order to reduce matters to their simplest form, it is common to consider efficiency as a function of input size. Among all inputs of the same size, we consider the maximum possible running time. This is called worst-case analysis . It is also possible, and often more meaningful, to measure average-case analysis . Average-case analyses tend to be more complex, and may require that some probability distribution be defined on the set of inputs. To keep matters simple, we will usually focus on worst-case analysis in this course.
Throughout out this course, when you are asked to present an algorithm, this means that you need to do three things:
Present a clear, simple and unambiguous description of the algorithm (in pseudo-code, for example). They key here is keep it simple . Uninteresting details should be kept to a minimum, so that the key compu- tational issues stand out. (For example, it is not necessary to declare variables whose purpose is obvious, and it is often simpler and clearer to simply say, Add X to the end of list L than to present code to do this or use some arcane syntax, such as L . insertAtEn d ( X ) .)
Present a justification or proof of the algorithms correctness. Your justification should assume that the reader is someone of similar background as yourself, say another student in this class, and should be con- vincing enough make a skeptic believe that your algorithm does indeed solve the problem correctly. Avoid rambling about obvious or trivial elements. A good proof provides an overview of what the algorithm does, and then focuses on any tricky elements that may not be obvious.
Present a worst-case analysis of the algorithms efficiency, typically it running time (but also its space, if space is an issue). Sometimes this is straightforward, but if not, concentrate on the parts of the analysis that are not obvious.
Note that the presentation does not need to be in this order. Often it is good to begin with an explanation of how you derived the algorithm, emphasizing particular elements of the design that establish its correctness and efficiency. Then, once this groundwork has been laid down, present the algorithm itself. If this seems to be a bit abstract now, dont worry. We will see many examples of this process throughout the semester.
Lecture 2: Mathematical Background
Read: Review Chapters 15 in CLRS.
Algorithm Analysis: Today we will review some of the basic elements of algorithm analysis, which were covered in previous courses. These include asymptotics, summations, and recurrences.
Asymptotics: Asymptotics involves O-notation (big-Oh) and its many relatives, , , o (little-Oh), . Asymp- totic notation provides us with a way to simplify the functions that arise in analyzing algorithm running times by ignoring constant factors and concentrating on the trends for large values of n . For example, it allows us to reason that for three algorithms with the respective running times
Next pageFont size:
Interval:
Bookmark:
Similar books «Design and Analysis of Computer Algorithms»
Look at similar books to Design and Analysis of Computer Algorithms. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Design and Analysis of Computer Algorithms and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.