TAM - C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS
Here you can read online TAM - C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2020, genre: Children. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
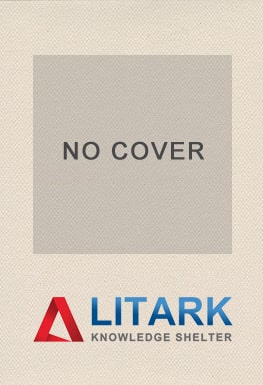
C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
TAM: author's other books
Who wrote C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS? Find out the surname, the name of the author of the book and a list of all author's works by series.
C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
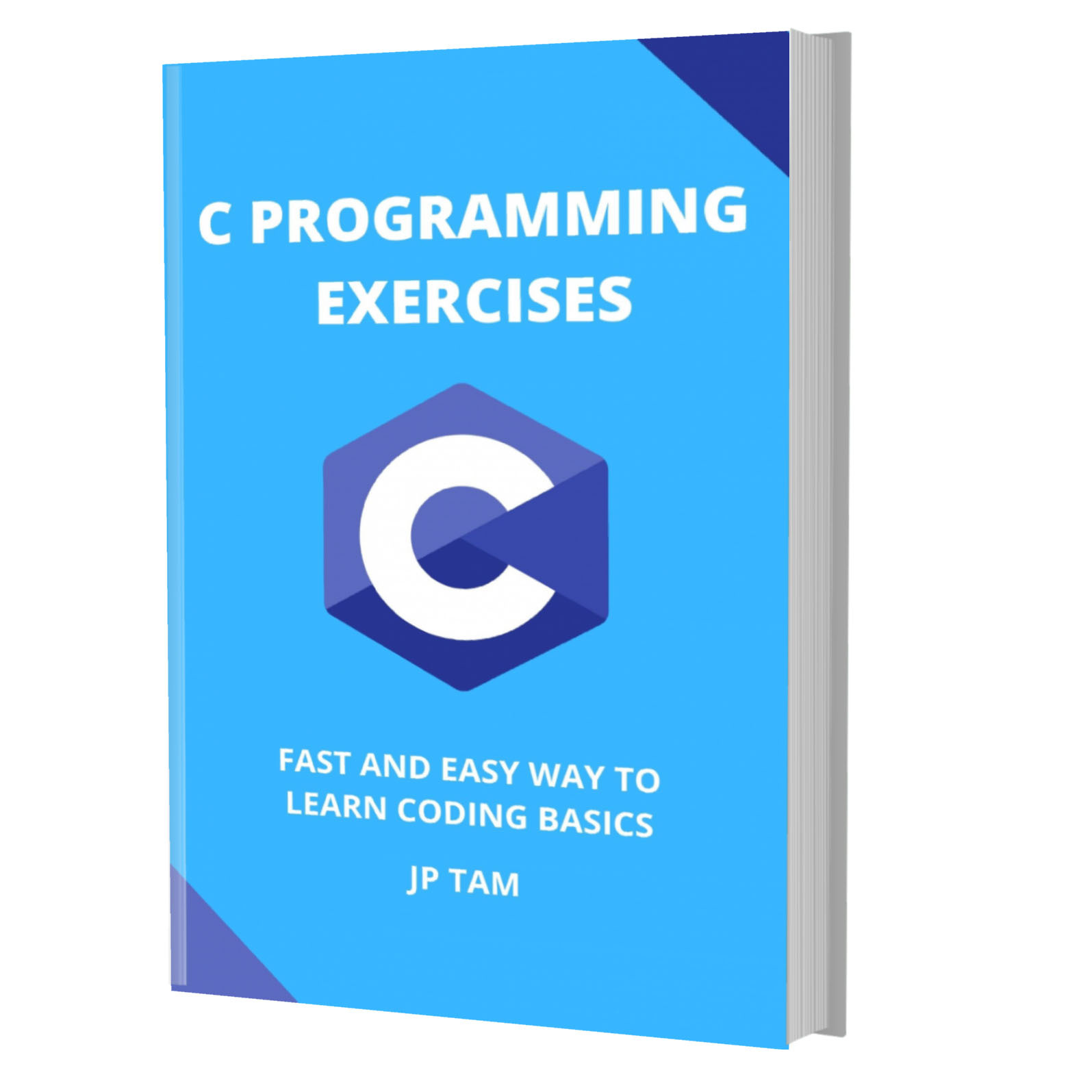
Font size:
Interval:
Bookmark:
Similar books «C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS»
Look at similar books to C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book C PROGRAMMING EXERCISES: FAST AND EASY WAY TO LEARN CODING BASICS and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.