Clinton W. Brownley - Working with Excel files in Python
Here you can read online Clinton W. Brownley - Working with Excel files in Python full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2018, publisher: OReilly Media, Inc., genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
- Book:Working with Excel files in Python
- Author:
- Publisher:OReilly Media, Inc.
- Genre:
- Year:2018
- Rating:5 / 5
- Favourites:Add to favourites
- Your mark:
- 100
- 1
- 2
- 3
- 4
- 5
Working with Excel files in Python: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Working with Excel files in Python" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Working with Excel files in Python — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Working with Excel files in Python" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Microsoft Excel is ubiquitous. We use Excel to store data on customers, inventory, and employees. We use it to track operations, sales, and financials. The list of ways people use Excel in business is long and diverse. Because Excel is such an integral tool in business, knowing how to process Excel files in Python will enable you to add Python into your data processing workflows, receiving data from other people and sharing results with them in ways theyre comfortable with.
Unlike Pythons csv
module, there is not a standard module in Python for processing Excel files (i.e., files with the .xls or .xlsx extension). To complete the examples in this section, you need to have the xlrd
and xlwt
packages. The xlrd
and xlwt
packages enable Python to process Excel files on any operating system, and they have strong support for Excel dates. If you installed Anaconda Python, then you already have the packages because theyre bundled into the installation.
A few words on terminology: when I refer to an Excel file thats the same thing as an Excel workbook. An Excel workbook contains one or more Excel worksheets. In this lesson, Ill be using the words file and workbook interchangeably, and Ill refer to the individual worksheets within a workbook as worksheets.
Well go through each of the examples here in base Python, so you can see every logical step in the data processing, and then using pandas, so you can have a (usually) shorter and more concise examplethough one thats a bit more abstractif you want to copy and modify it for use in your work.
To get started with the examples in this lesson, we need to create an Excel workbook:
Open Microsoft Excel.
Add three separate worksheets to the workbook and name them january_2013, february_2013, and march_2013. Then add the data as shown in , respectively.
Save the workbook as sales_2013.xlsx.
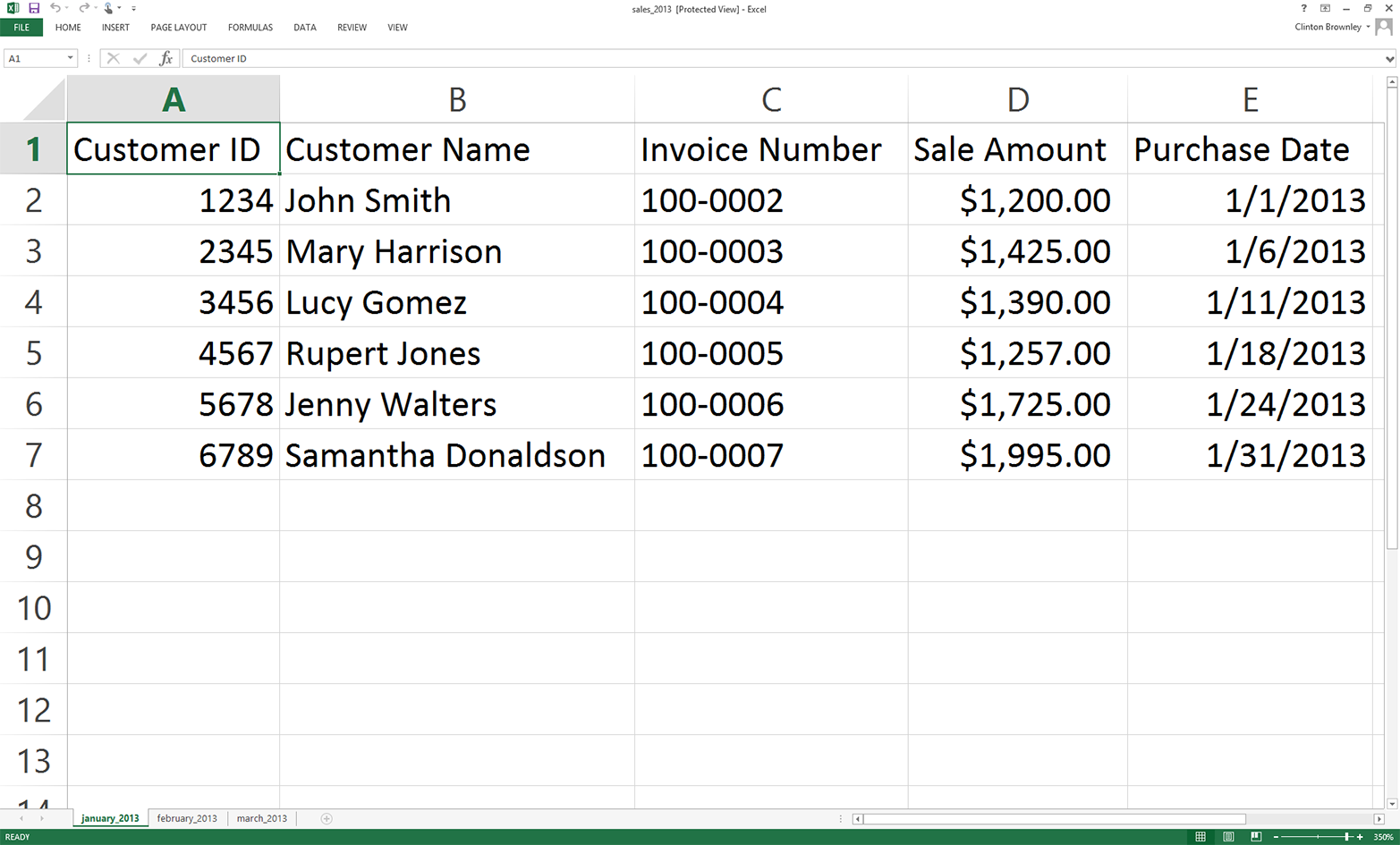
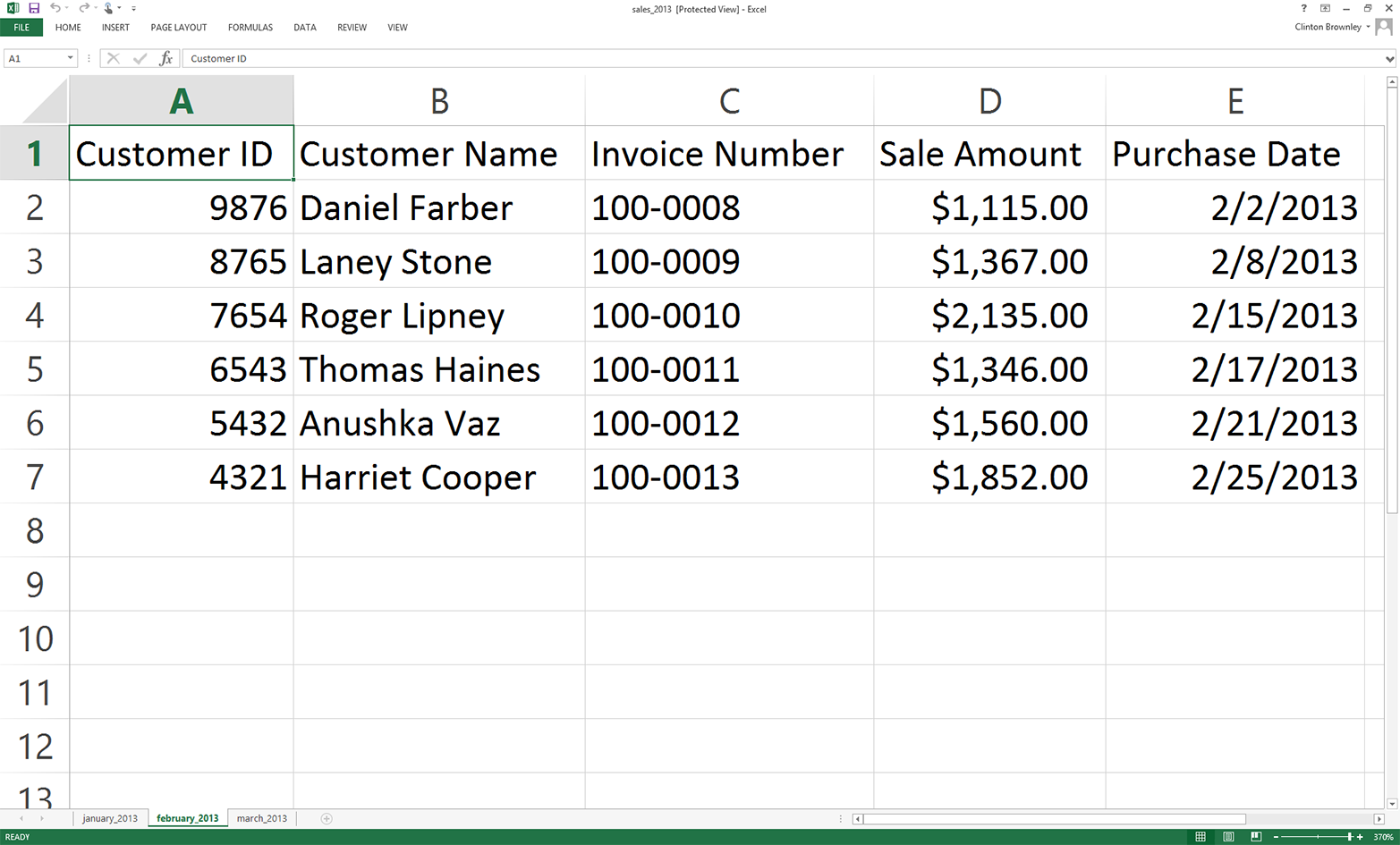
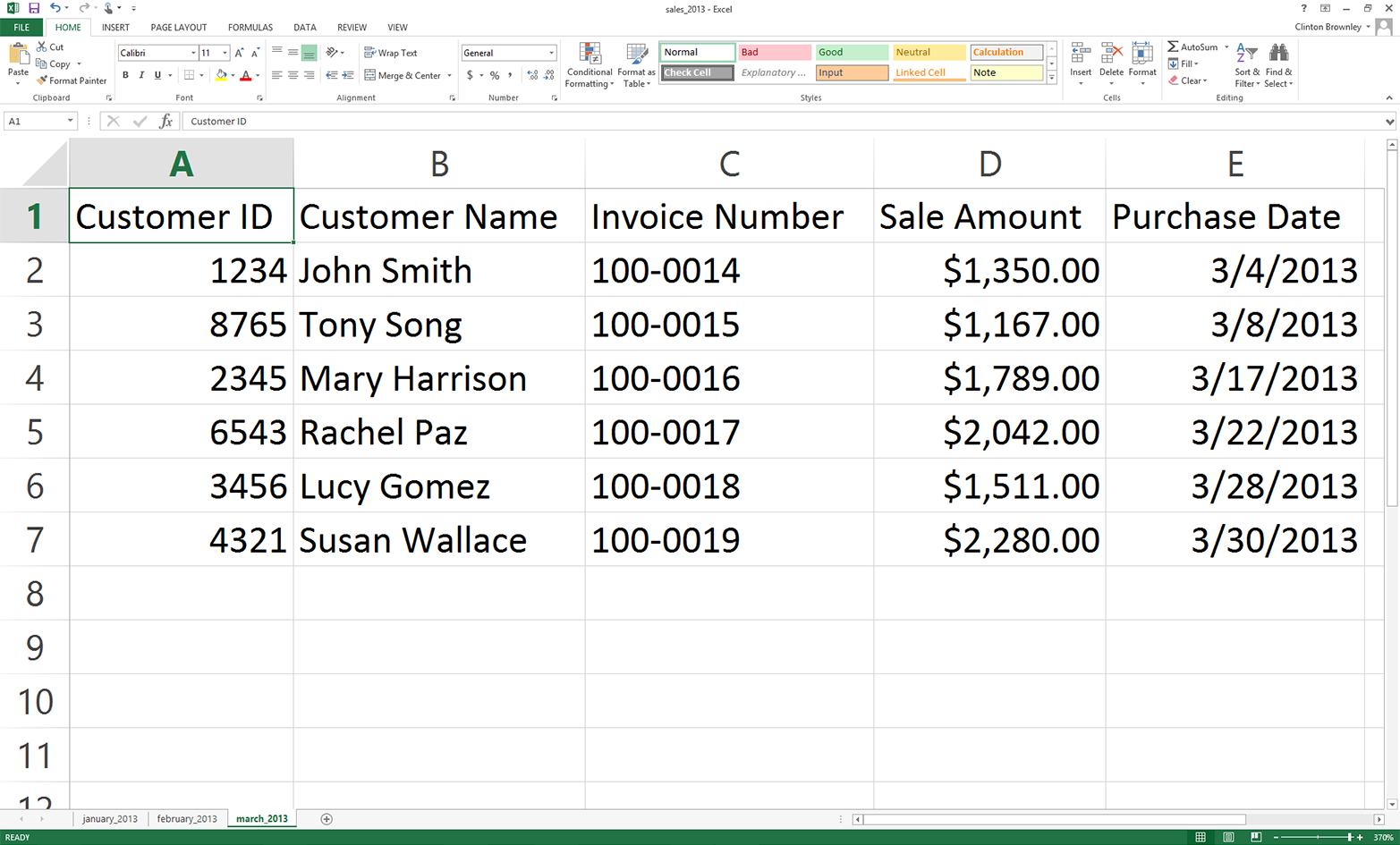
Now that we have an Excel workbook that contains three worksheets, lets learn how to process an Excel workbook in Python. As a reminder, we are using the xlrd
and xlwt
packages in this lesson, so make sure you have already downloaded and installed these add-in packages.
As you are probably already aware, Excel files are different from CSV files in at least two important respects. First, unlike a CSV file, an Excel file is not a plain-text file, so you cannot open it and view the data in a text editor. You can see this by right-clicking on the Excel workbook you just created and opening it in a text editor like Notepad or TextWrangler. Instead of legible data, you will see a mess of special characters.
Second, unlike a CSV file, an Excel workbook is designed to contain multiple worksheets. Because a single Excel workbook can contain multiple worksheets, we need to learn how to introspect (i.e., look inside and examine) all of the worksheets in a workbook without having to manually open the workbook. By introspecting a workbook, we can examine the number of worksheets and the types and amount of data on each worksheet before we actually process the data in the workbook.
Introspecting Excel files is useful to make sure that they contain the data you expect, and to do a quick check for consistency and completeness. That is, understanding the number of input files and the number of rows and columns in each file will give you some idea about the size of the processing job as well as the potential consistency of the file layouts.
Once you understand how to introspect the worksheets in a workbook, we will move on to parsing a single worksheet, iterating over multiple worksheets, and then iterating over multiple workbooks.
To determine the number of worksheets in the workbook, the names of the worksheets, and the number of rows and columns in each of the worksheets, type the following code into a text editor and save the file as 1excel_introspect_workbook.py:
#!/usr/bin/env python3
import
sys
from
xlrd
import
open_workbook
input_file
=
sys
.
argv
[
1
]
workbook
=
open_workbook
(
input_file
)
print
(
'Number of worksheets:'
,
workbook
.
nsheets
)
for
worksheet
in
workbook
.
sheets
():
print
(
"Worksheet name:"
,
worksheet
.
name
,
"
\t
Rows:"
,
\ worksheet
.
nrows
,
"
\t
Columns:"
,
worksheet
.
ncols
)
show what the script looks like in Anaconda Spyder, Notepad++ (Windows), and TextWrangler (macOS), respectively.
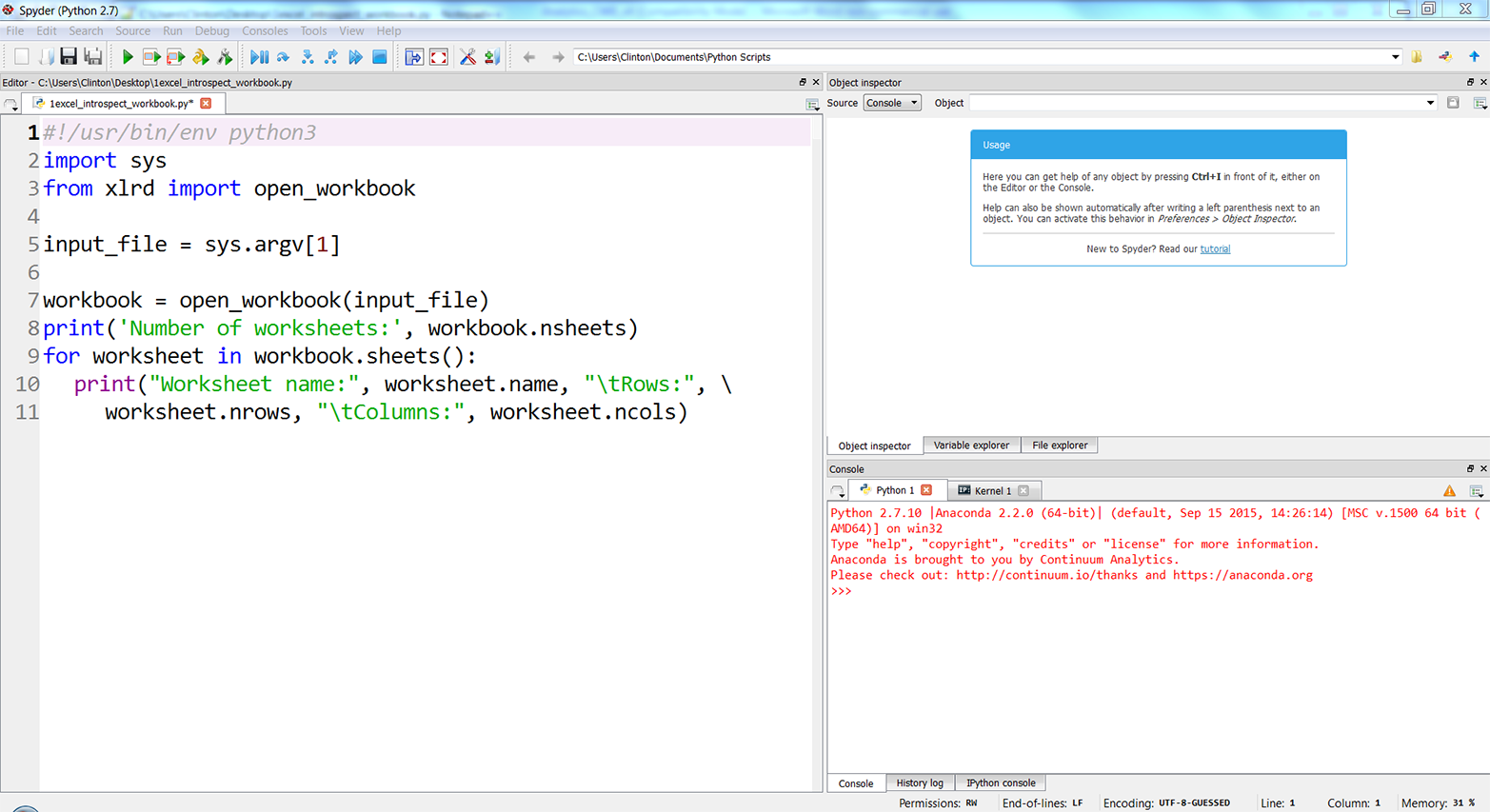
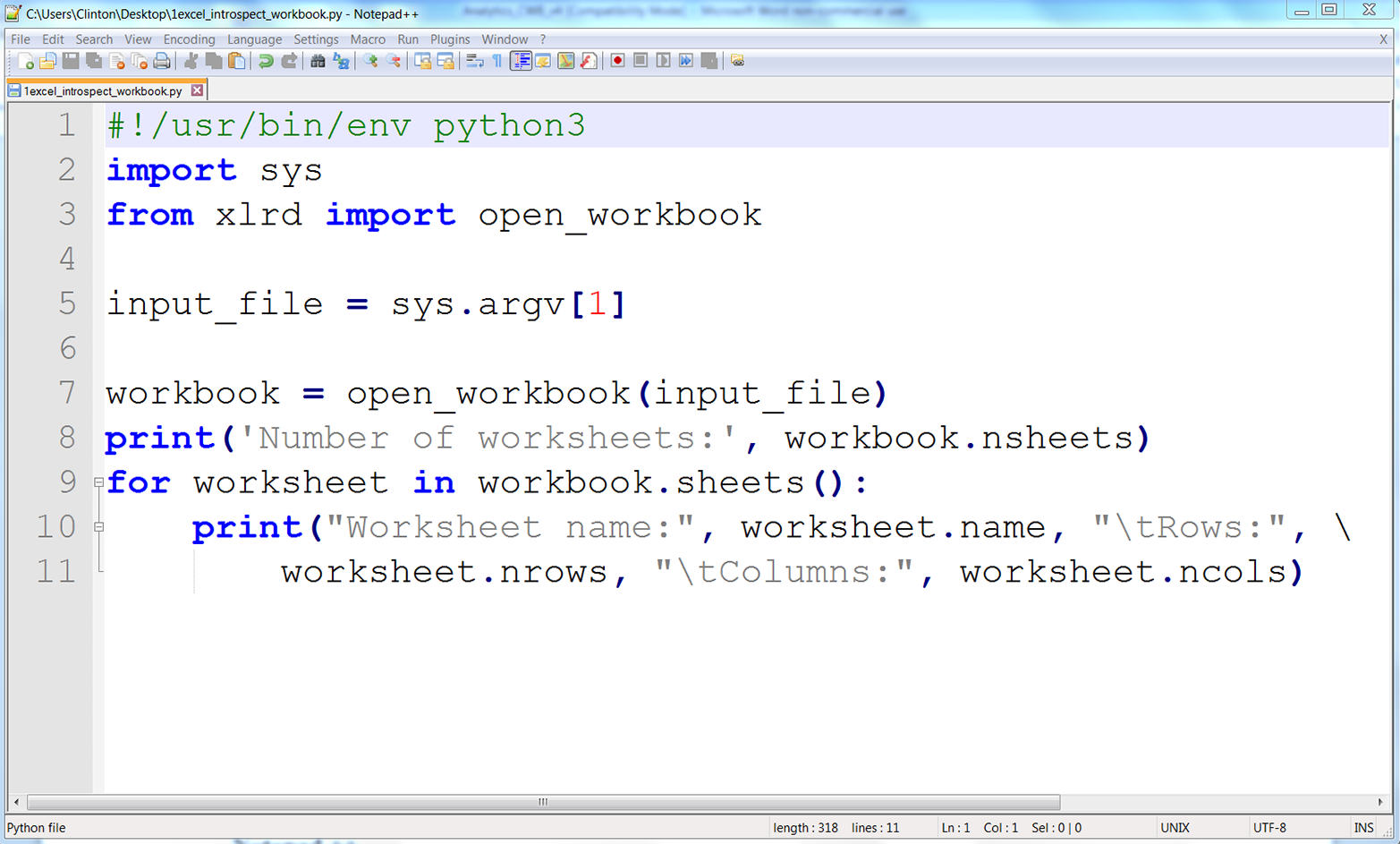
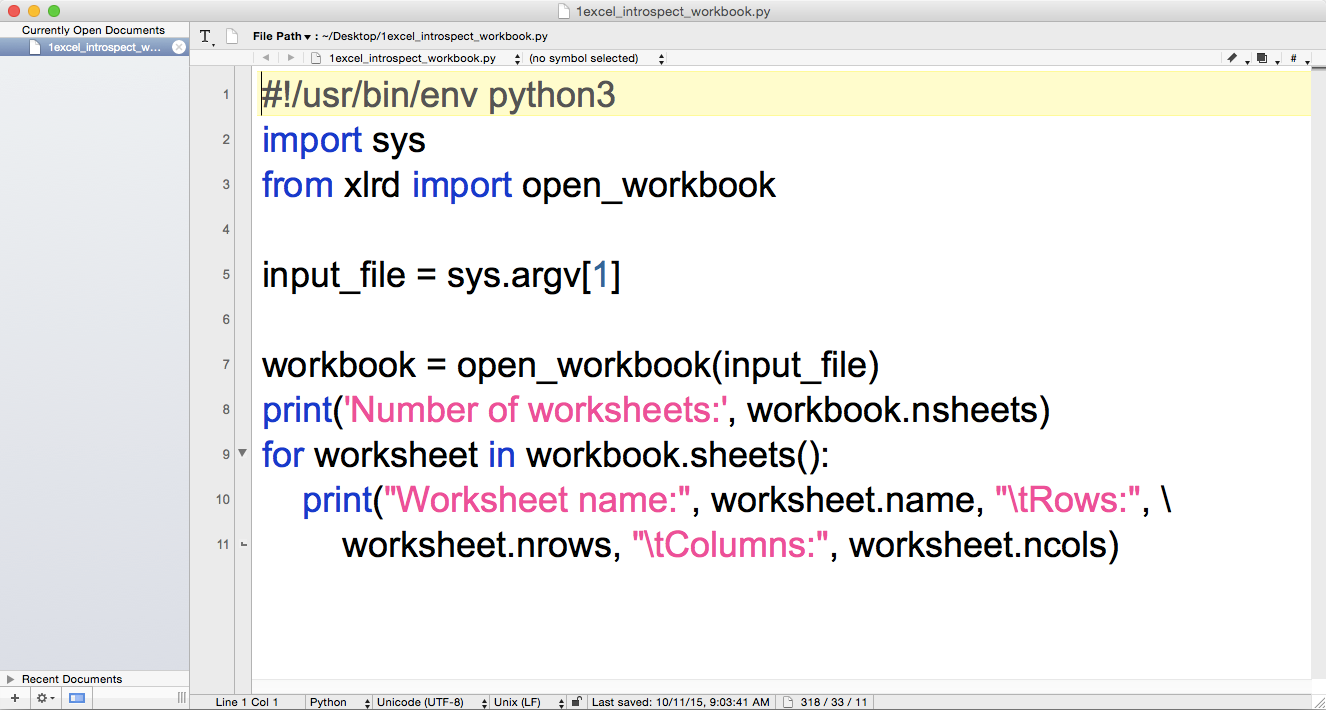
Line 3 imports the xlrd
modules open_workbook
function so we can use it to read and parse an Excel file.
Line 7 uses the open_workbook
function to open the Excel input file into an object Ive named workbook
. The workbook
object contains all of the available information about the workbook, so we can use it to retrieve individual worksheets from the workbook.
Line 8 prints the number of worksheets in the workbook.
Line 9 is a for
loop that iterates over all of the worksheets in the workbook. The workbook
objects sheets
method identifies all of the worksheets in the workbook.
Line 10 prints the name of each worksheet and the number of rows and columns in each worksheet to the screen. The print
statement uses the worksheet
objects name
attribute to identify the name of each worksheet. Similarly, it uses the nrows
and ncols
attributes to identify the number of rows and columns, respectively, in each worksheet.
Font size:
Interval:
Bookmark:
Similar books «Working with Excel files in Python»
Look at similar books to Working with Excel files in Python. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Working with Excel files in Python and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.