it-ebooks - Full Speed Python
Here you can read online it-ebooks - Full Speed Python full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2018, publisher: iBooker it-ebooks, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
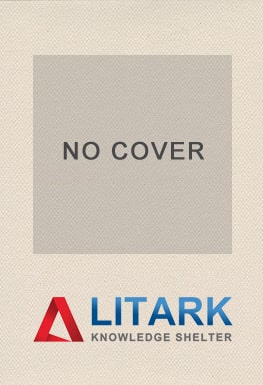
Full Speed Python: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Full Speed Python" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Full Speed Python — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Full Speed Python" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
v0.4.3
Joo Ventura
This book aims to teach the Python programming language using a practical approach. Its method is quite simple: after a short introduction to each topic, the reader is invited to learn more by solving the proposed exercises.
These exercises have been used extensively in my web development and distributed computing classes at the Superior School of Technology of Setbal. With these exercises, most students are up to speed with Python in less than a month. In fact, students of the distributed computing course, taught in the second year of the software engineering degree, become familiar with Pythons syntax in two weeks and are able to implement a distributed client-server application with sockets in the third week.
Please note that this book is a work in progress and, as such, may contain a few spelling errors that may be corrected in the future. However it is made available now as it is so it can be useful to anyone who wants to use it. I sincerely hope you can get something good through it.
The source of this book is available on github (https://github.com/joaoventura/full-speed-python). I welcome any pull requests to correct misspellings, suggest new exercises or to provide clarification of the current content.
All the best,
Joo Ventura - Adjunct Professor at the Escola Superior de Tecnologia de Setbal.
In this chapter we will install and run the Python interpreter in your local computer.
Download the latest Python 3 release for Windows on https://www.python.org/downloads/windows/ and execute the installer. At the time of writing, this is Python 3.6.4.
Make sure that the Install launcher for all users and Add Python to PATH settings are selected and choose Customize installation.
Windows installation
In the next screen Optional Features, you can install everything, but it is essential to install pip and pylauncher (for all users). Pip is the Python package manager that allows you to install several Python packages and libraries.
In the Advanced Options, make sure that you select Add Python to environment variables. Also, I suggest that you change the install location to something like C:\Python36\ as it will be easier for you to find the Python installation if something goes wrong.
Windows installation
Finally, allow Python to use more than 260 characters on the file system by selecting Disable path length limit and close the installation dialog.
Windows installation
Now, open the command line (cmd) and execute python or python3. If everything was correctly installed, you should see the Python REPL. The REPL (from Read, Evaluate, Print and Loop) is a environment that you can use to program small snippets of Python code. Run exit() to leave the REPL.
Python REPL
You can download the latest macOS binary releases from https://www.python.org/downloads/mac-osx/. Make sure you download the latest Python 3 release (3.6.4 at the time of writing). You can also use Homebrew, a package manager for macOS (https://brew.sh/). To install the latest Python 3 release with Homebrew, just do brew install python3
on your terminal. Another option is to use the MacPorts package manager (https://www.macports.org/) and command port install python36
.
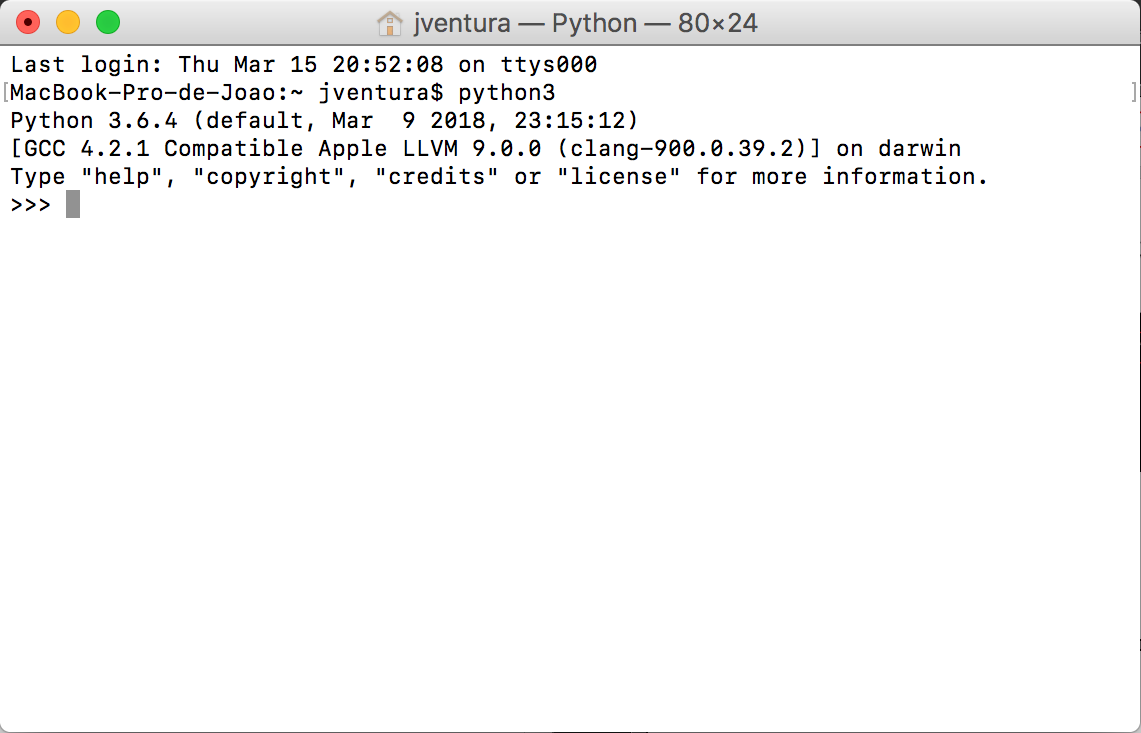
Finally, open the terminal, execute python3
and you should see the Python REPL as above. Press Ctrl+D or write exit()
to leave the REPL.
To install Python on Linux, you can download the latest Python 3 source releases from https://www.python.org/downloads/source/ or use your package manager (apt-get, aptitude, synaptic and others) to install it. To make sure you have Python 3 installed on your system, run python3 --version
in your terminal.
Finally, open the terminal, execute python3
and you should see the Python REPL as in the following image. Press Ctrl+D or write exit()
to leave the REPL.
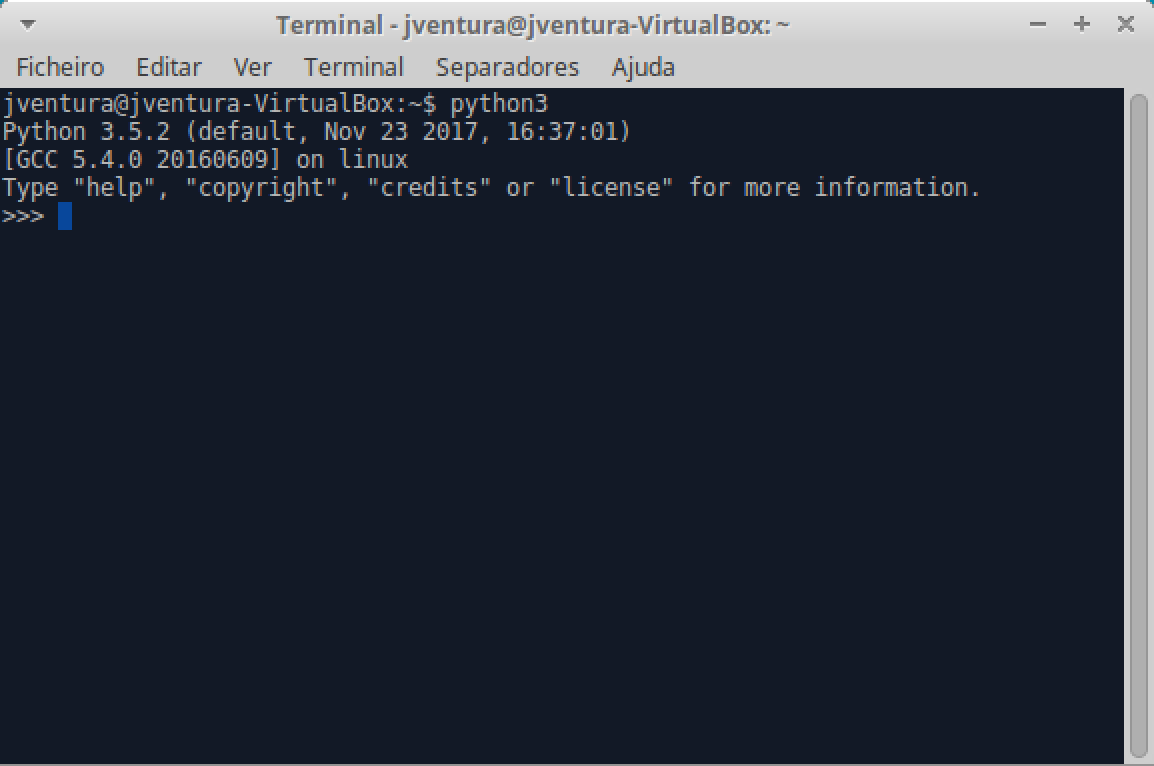
In this chapter we will work with the most basic datatypes, numbers and strings. Start your Python REPL and write the following:
>>> a = >>> type (a) < class 'int' > >>> b = 2.5 >>> type (b) < class 'float' >
Basically, you are declaring two variables (named a and b) which will hold some numbers: variable a is an integer number while variable b is a real number. We can now use our variables or any other numbers to do some calculations:
>>> a + b 4.5 >>> (a + b) * 9.0 >>> + + - / 7.333333333333333
Python also has support for string datatypes. Strings are sequences of characters (like words) and can be defined using single or double quotes:
>>> hi = "hello" >>> hi 'hello' >>> bye = 'goodbye' >>> bye 'goodbye'
You can add strings to concatenate them but you can not mix different datatypes, such as strings and integers.
>>> hi + "world" 'helloworld' >>> "Hello" + Traceback (most recent call last): File "" , line , in < module > TypeError : must be str , not int
However, multiplication works as repetition:
>>> "Hello" * 'HelloHelloHello'
Try the following mathematical calculations and guess what is happening: ((3 / 2)), ((3 // 2)), ((3 % 2)), ((3**2)).
Suggestion: check the Python library reference at https://docs.python.org/3/library/stdtypes.html#numeric-types-int-float-complex.
Calculate the average of the following sequences of numbers: (2, 4), (4, 8, 9), (12, 14/6, 15)
The volume of a sphere is given by (4/3 * pi * r^3). Calculate the volume of a sphere of radius 5. Suggestion: create a variable named pi with the value of 3.1415.
Use the modulo operator (%) to check which of the following numbers is even or odd: (1, 5, 20, 60/7).
Suggestion: the remainder of (x/2) is always zero when (x) is even.
Find some values for (x) and (y) such that (x < 1/3 < y) returns True on the Python REPL. Suggestion: try (0 < 1/3 < 1) on the REPL.
Using the Python documentation on strings (https://docs.python.org/3/library/stdtypes.html?#text-sequence-type-str), solve the following exercises:
Initialize the string abc on a variable named s:
Use a function to get the length of the string.
Font size:
Interval:
Bookmark:
Similar books «Full Speed Python»
Look at similar books to Full Speed Python. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Full Speed Python and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.