it-ebooks - Tiny Python & ES6 Notebook
Here you can read online it-ebooks - Tiny Python & ES6 Notebook full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2017, publisher: iBooker it-ebooks, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
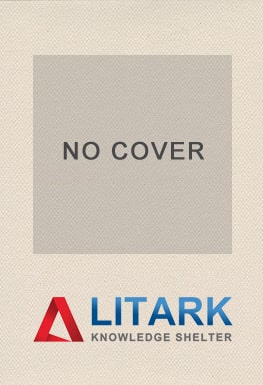
Tiny Python & ES6 Notebook: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "Tiny Python & ES6 Notebook" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Tiny Python & ES6 Notebook — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "Tiny Python & ES6 Notebook" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
From: mattharrison/Tiny-Python-2.7-Notebook
From: mattharrison/Tiny-Python-3.6-Notebook
From: mattharrison/Tiny-es6-Notebook
To check if Python is installed, run the following from a terminal:
python --versionOtherwise, install Python from the website .
http://python.org |
The Python executable will behave differently depending on the command line options you give it:
Start the Python REPL:
pythonExecute the
python file.pyfile.py
file:Execute the
python -i file.pyfile.py
file, and drop into REPL with namespace offile.py
:Execute the
python -m json.tooljson/tool.py
module:Execute
python -c "print 'hi'""print 'hi'"
- Use the
help
function to read the documentation for a module/class/function. As a standalone invocation,you enter the help system and can explore various topics. - Use the
dir
function to list contents of the namespace, or attributes of an object if you pass one in
Note
The majority of code in this book is written as if it where executed in a REPL. If youare typing it in, ignore the primary and secondary prompts (>>>
and ...
).
This is not so much an instructional manual, but rather notes, tables, andexamples for Python syntax. It was created by the author as an additionalresource during training, meant to be distributed as a physical notebook.Participants (who favor the physical characteristics of dead tree material)could add their own notes, thoughts, and have a valuable referenceof curated examples.
Run the following in an interpreter to get an easter egg that describes some of the ethos behind Python. This is also codified in PEP 20:
>>> import thisThe Zen of Python, by Tim PetersBeautiful is better than ugly.Explicit is better than implicit.Simple is better than complex.Complex is better than complicated.Flat is better than nested.Sparse is better than dense.Readability counts.Special cases aren't special enough to break therules.Although practicality beats purity.Errors should never pass silently.Unless explicitly silenced.In the face of ambiguity, refuse the temptationto guess.There should be one --and preferably only one--obvious way to do it.Although that way may not be obvious at firstunless you're Dutch.Now is better than never.Although never is often better than *right* now.If the implementation is hard to explain, it's abad idea.If the implementation is easy to explain, it maybe a good idea.Namespaces are one honking great idea -- let'sdo more of those!These might just seem like silly one liners, but there is a lot of wisdompacked in here. It is good for Python programmers to review theseevery once in a while and see if these hold true for their code. (Or tojustify their code reviews)
In the default namespace you have access to various callables:
Built in callablesOperation | Result |
---|---|
abs(x) | Absolute value protocol (call x.__abs__() ) |
all(seq) | Boolean check if all items in seq are truthy |
any(seq) | Boolean check if at least one item in seq is truthy |
apply(callable, [args, [kwargs]) | Call callable(*args, **kwargs) |
bin(i) | String containing binary version of number (int(bin(i), 2) to reverse) |
bool(x) | Boolean protocol (call x.__nonzero__() then x.__len__() ) |
buffer(obj, [offset, [size]) | Create buffer object from obj |
bytearray(x) | Create a mutable bytearray from iterable of ints, text string, bytes, an integer, or pass nothing for an empty bytearray |
bytes(x) | Create an immutable bytes from iterable of ints, text string, bytes, an integer, or pass nothing for an empty bytes |
callable(x) | Boolean check if you can do x() (ie x.__call__ exists) |
chr(i) | Convert integer codepoint to Unicode string (ord(chr(i)) to reverse) |
@classmethod | Use to decorate a method so you can invoke it on the class |
cmp(a, b) | -1, 0, or 1 if a <, == or > b |
coerce(num1, num2) | Coerce nums to common type |
compile(source, fname, mode) | Compile source to code (fname used for error, mode is exec : module, single : statement, eval : expression). Can run eval(code) on expression, exec(code) on statement |
complex(i, y) | Create complex number |
copyright | Python copyright string |
credits | Python credits string |
delattr(obj, attr) | Remove attribute from obj (del obj.attr ) |
dict([x]) | Create a dictionary from a mapping, iterable of k,v tuples, named parameters, or pass nothing for an empty dictionary |
dir([obj]) | List attributes of obj , or names in current namespace if no obj provided |
divmod(num, denom) | Return tuple pair of num//denom and num%denom |
enumerate(seq, [start]) | Return iterator of index, item tuple pairs. Index begins at start or 0 (default) |
eval(source, globals=None, locals=None) | Run source (expression string or result of compile ) with globals and locals |
execfile(filename, globals=None, locals=None) | Run code in filename with globals and locals |
exit(code) | Exit Python interpreter and return code |
file(name, [mode, [buffering]]) | Open a file with mode |
filter([function], seq) | Return iterator of items where function(item) is truthy (or item is truthy if function is missing) |
float(x) | Convert string or number to float (call x.__float__() ) |
format(obj, fmt) | Format protocol (call obj.__format__(fmt) ) |
frozenset([seq]) | Create frozenset from seq (empty if missing) |
getattr(obj, attr) | Get attribute from obj (obj.attr ) |
globals() | Return mutable dictionary with current global variables |
hasattr(obj, attr) | Check if attribute on obj (obj.attr doesn't throw AttributeError ) |
hash(x) |
Font size:
Interval:
Bookmark:
Similar books «Tiny Python & ES6 Notebook»
Look at similar books to Tiny Python & ES6 Notebook. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book Tiny Python & ES6 Notebook and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.