About the Author
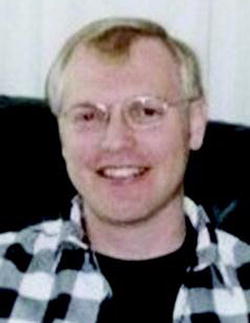
Jeff Friesen is a freelance tutor and software developer with an emphasis on Java (and now Android). In addition to writing this book, Jeff has written numerous articles on Java and other technologies for JavaWorld ( www.javaworld.com ), informIT ( www.informit.com ), java.net, DevSource ( www.devsource.com ), SitePoint ( www.sitepoint.com ), BuildMobile ( www.buildmobile.com ), and JSPro ( www.jspro.com ). Jeff can be contacted via his web site at tutortutor.ca .
About the Technical Reviewers
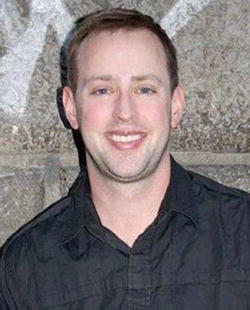
Paul Connolly is the Director of Engineering for Atypon Systems RightSuite product line. RightSuite is an enterprise access-control and commerce solution used by many of the worlds largest publishing and media companies. Paul enjoys designing and implementing high-performance, enterprise-class software systems. He is also an active contributor in the open-source community.
Prior to joining Atypon Systems, Paul worked as a senior software engineer at Standard & Poors where he designed and developed key communications systems. Paul is a Sun Certified Java Programmer, Sun Certified Business Component Developer, and a Sun Certified Web Component Developer. Paul lives in Rochester, NY, with his wife Marina and daughter Olivia.
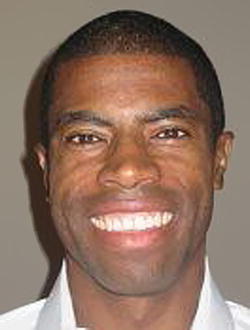
Chd Darby is an author, instructor and speaker in the Java development world. As a recognized authority on Java applications and architectures, he has presented technical sessions at software development conferences worldwide. In his 15 years as a professional software architect, hes had the opportunity to work for Blue Cross/Blue Shield, Merck, Boeing, Northrop Grumman, and a handful of startup companies.
Chd is a contributing author to several Java books, including Professional Java E-Commerce (Wrox Press), Beginning Java Networking (Wrox Press), and XML and Web Services Unleashed (Sams Publishing). Chd has Java certifications from Sun Microsystems and IBM. He holds a B.S. in Computer Science from Carnegie Mellon University.
You can read Chds blog at www.luv2code.com and follow him on Twitter @darbyluvs2code.
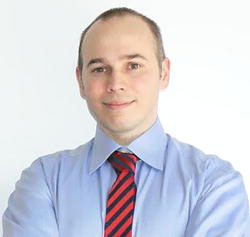
Onur Cinar is the author of Android Apps with Eclipse, and Pro Android C++ with the NDK books from Apress. He has over 17 years of experience in design, development, and management of large scale complex software projects, primarily in mobile and telecommunication space. His expertise spans VoIP, video communication, mobile applications, grid computing, and networking technologies on diverse platforms. He has been actively working with the Android platform since its beginning. He has a B.S. degree in Computer Science from Drexel University in Philadelphia, PA. He is currently working at the Skype division of Microsoft as the Sr. Product Engineering Manager for the Skype client on Android platform.
Acknowledgments
I thank Steve Anglin for contacting me to write this book; Katie Sullivan for guiding me through the various aspects of this project; Tom Welsh and Matthew Moodie for helping me with the development of my chapters; and Paul Connolly, Chd Darby, and Onur Cinar for their diligence in catching various flaws that would otherwise have made it into this book.
Appendix A
Solutions to Exercises
Each of closes with an Exercises section that tests your understanding of the chapters material. Solutions to these exercises are presented in this appendix.
Chapter 1: Getting Started with Java
- Java is a language and a platform. The language is partly patterned after the C and C++ languages to shorten the learning curve for C/C++ developers. The platform consists of a virtual machine and associated execution environment.
- A virtual machine is a software-based processor that presents its own instruction set.
- The purpose of the Java compiler is to translate source code into instructions (and associated data) that are executed by the virtual machine.
- The answer is true: a classfiles instructions are commonly referred to as bytecode.
- When the virtual machines interpreter learns that a sequence of bytecode instructions is being executed repeatedly, it informs the virtual machines Just In Time (JIT) compiler to compile these instructions into native code.
- The Java platform promotes portability by providing an abstraction over the underlying platform. As a result, the same bytecode runs unchanged on Windows-based, Linux-based, Mac OS Xbased, and other platforms.
- The Java platform promotes security by providing a secure environment in which code executes. It accomplishes this task in part by using a bytecode verifier to make sure that the classfiles bytecode is valid.
- The answer is false: Java SE is the platform for developing applications and applets.
- The JRE implements the Java SE platform and makes it possible to run Java programs.
- The difference between the public and private JREs is that the public JRE exists apart from the JDK, whereas the private JRE is a component of the JDK that makes it possible to run Java programs independently of whether or not the public JRE is installed.
- The JDK provides development tools (including a compiler) for developing Java programs. It also provides a private JRE for running these programs.
- The JDKs javac tool is used to compile Java source code.
- The JDKs java tool is used to run Java applications.
- Standard I/O is a mechanism consisting of Standard Input, Standard Output, and Standard Error that makes it possible to read text from different sources (keyboard or file), write nonerror text to different destinations (screen or file), and write error text to different destinations (screen or file).
- You specify the main() methods header as public static void main(String[] args) .
- An IDE is a development framework consisting of a project manager for managing a projects files, a text editor for entering and editing source code, a debugger for locating bugs, and other features. The IDE that Google supports for developing Android apps is Eclipse.
Chapter 2: Learning Language Fundamentals
- Unicode is a computing industry standard for consistently encoding, representing, and handling text thats expressed in most of the worlds writing systems.
- A comment is a language feature for embedding documentation in source code.
- The three kinds of comments that Java supports are single-line, multiline, and Javadoc.
- An identifier is a language feature that consists of letters (AZ, az, or equivalent uppercase/lowercase letters in other human alphabets), digits (09 or equivalent digits in other human alphabets), connecting punctuation characters (e.g., the underscore), and currency symbols (e.g., the dollar sign $).This name must begin with a letter, a currency symbol, or a connecting punctuation character; and its length cannot exceed the line in which it appears.
- The answer is false: Java is a case-sensitive language.
- A type is a language feature that identifies a set of values (and their representation in memory) and a set of operations that transform these values into other values of that set.
Next page