Gupta - 100+ Most Important C Programs with Output: for Students & Teachers
Here you can read online Gupta - 100+ Most Important C Programs with Output: for Students & Teachers full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2016, publisher: R.K.G, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
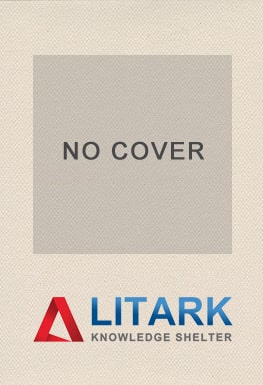
100+ Most Important C Programs with Output: for Students & Teachers: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "100+ Most Important C Programs with Output: for Students & Teachers" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
Gupta: author's other books
Who wrote 100+ Most Important C Programs with Output: for Students & Teachers? Find out the surname, the name of the author of the book and a list of all author's works by series.
100+ Most Important C Programs with Output: for Students & Teachers — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "100+ Most Important C Programs with Output: for Students & Teachers" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Copyright 2016 by Rohit Gupta- All rights reserved. This book is copyright protected. This is only for personal use. You cannot amend, distribute, sell, use, quote or paraphrase any part or the content within this book without the consent of the author or copyright owner. Except as permitted under India Copyright Law, no part of this book may be reprinted, reproduced, transmitted, or utilized in any form by any electronic, mechanical, or other means, now known or hereafter invented, including photocopying, microfilming, and recording, or in any information storage or retrieval system, without written permission from the publishers. This book is a work of pure practical knowledge.
Any resemblance to other book is entirely coincidental.
Table of Contents
1. Write a program to perform addition, subtraction, multiplication and division.
2. How to add two numbers without using the plus operator?
In C ~ is 1's complement operator. This is equivalent to: ~b = -(b + 1) So, a - ~b -1 = a-[-(b + 1)] -1 = a + b + 1 - 1 = a + b
How to subtract two numbers without using the subtraction operator ?
In C ~ is 1's complement operator. This is equivalent to: ~b = -(b + 1) So, a + ~b +1 = a+[-(b + 1)] +1 = a - b - 1 + 1 = a - b
4.
Write a program for swapping of two numbers.
5. Write a program to add digits of a number or calculate sum of digits.
6. Write a program to print complex number.
7. Write a program to check whether input alphabet is a vowel or not.
8.
Write a program to check if given integer is positive or negative.
9. Write a program to find the area of a trapezium.
10. Write a program for swapping of two numbers using function (call by value).
Sample Output: Before swapping: num1 = 10 num2 = 30 After swapping: num1 =30 num2 = 10
11. Write a program to find greatest number between three numbers.
12. Write a program to find greatest number among four numbers by ternary operator.
In computer programming, ?: is a ternary operator that is part of the syntax for a basic conditional expression in several programming languages. Syntax: expression 1 ? expression 2 : expression 3
13. Write a program to find smallest number among four numbers by using ternary operator.
14. Write a program to find largest element using dynamic memory allocation.
Font size:
Interval:
Bookmark:
Similar books «100+ Most Important C Programs with Output: for Students & Teachers»
Look at similar books to 100+ Most Important C Programs with Output: for Students & Teachers. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book 100+ Most Important C Programs with Output: for Students & Teachers and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.