it-ebooks - UIUC Crowd-Sourced System Programming Book
Here you can read online it-ebooks - UIUC Crowd-Sourced System Programming Book full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2017, publisher: iBooker it-ebooks, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
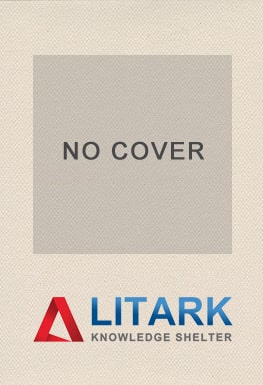
UIUC Crowd-Sourced System Programming Book: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "UIUC Crowd-Sourced System Programming Book" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
UIUC Crowd-Sourced System Programming Book — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "UIUC Crowd-Sourced System Programming Book" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
From: Home
Welcome to Angrave's crowd-sourced System Programming wiki-book!This wiki is being built by students and faculty from the University of Illinois and is a crowd-source authoring experiment by Lawrence Angrave from CS @ Illinois.
Rather than requiring an existing paper-based book this semester, we will build our own set of resources here.
- Printing to Streams
- Parsing Input
- Memory Mistakes
- Logic/Programming Flow
- Other Gotchas
- Strings
- Structs
- In Code Debugging
- Valgrind
- Tsan
- GDB
- Overview
- Process Contents
- Bonus: More Contents
- Introduction
- Waiting and Execing
- The Pattern
- Zombies
- Wait Macros
- Signals
- C Dynamic Memory Allocation
- Introduction to Allocating
- Memory Allocator Tutorial
- Intro to Threads
- Simple Pthreads
- More pthread Functions
- Intro to Race Conditions
- Solving Critical Sections
- Mutex Gotchas
- Thread Safe Stack
- Stack Semaphores
- Candidate Solutions
- Working Solutions
- Hardware Solutions
- Intro To Condition Variables
- Implementing a Counting Semaphore
- Failed Solutions
- Viable Solutions
- What is Virtual Memory?
- Advanced Frames and Protections
- Pipe Gotchas
- Named Pipes
- Thinking about Scheduling
- Measures of Efficiency
- Navigation/Terminology
- What's a File System?
- Reliability with a Single Disk
- Redundancy
- Wait Macros
- Signals
- Signals in Depth
- Disposition in Threads/Children
Warning these are good practice but not comprehensive. The CS241 final assumes you fully understand and can apply all topics of the course. Questions will focus mostly but not entirely on topics that you have used in the lab and programming assignments.
- (todo)
If you are taking CS241 you can submit this homework at this Google Form.
http://cs-education.github.io/sys/
There is also the course wikibook
https://github.com/angrave/SystemProgramming/wiki
Questions? Comments? Use Piazza,https://piazza.com/illinois/spring2017/cs241/home
The in-browser virtual machine runs entirely in Javascript and is fastest in Chrome. Note the VM and any code you write is reset when you reload the page So copy your code to a separate document. The post-video challenges (e.g. Haiku poem) are not part of homework 0.
- Hello World (System call style)
- Write a program that uses
write()
to print out "Hi! My name is ".
- Write a program that uses
- Hello Standard Error Stream
- Write a program that uses
write()
to print out a triangle of heightn
to Standard Error- n should be a variable and the triangle should look like this for n = 3
******
- Write a program that uses
- Writing to files
- Take your program from "Hello World" and have it write to a file
- Make sure to to use some interesting flags and mode for
open()
man 2 open
is your friend
- Make sure to to use some interesting flags and mode for
- Take your program from "Hello World" and have it write to a file
- Not everything is a system call
- Take your program from "Writing to files" and replace it with
printf()
(Make sure to print to the file!) - Name some differences from
write()
andprintf()
- Take your program from "Writing to files" and replace it with
- Not all bytes are 8 bits?
- How many bits are there in a byte?
- How many bytes is a
char
? - Tell me how many bytes the following are on your machine:
int, double, float, long, long long
- Follow the int pointer
- On a machine with 8 byte integers:
int main (){ int data[];}If the address of data is
0x7fbd9d40
, then what is the address ofdata+2
?- What is
data[3]
equivalent to in C?
sizeof
character arrays, incrementing pointersRemember the type of a string constant
"abc"
is an array.- Why does this segfault?
char *ptr = " hello " ;*ptr = ' J ' ;- What does
sizeof("Hello\0World")
return? - What does
strlen("Hello\0World")
return? - Give an example of X such that
sizeof(X)
is 3 - Give an example of Y such that
sizeof(Y)
might be 4 or 8 depending on the machine.
- Program arguments
argc
argv
- Name me two ways to find the length of
argv
- What is
argv[0]
- Name me two ways to find the length of
- Environment Variables
Font size:
Interval:
Bookmark:
Similar books «UIUC Crowd-Sourced System Programming Book»
Look at similar books to UIUC Crowd-Sourced System Programming Book. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book UIUC Crowd-Sourced System Programming Book and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.