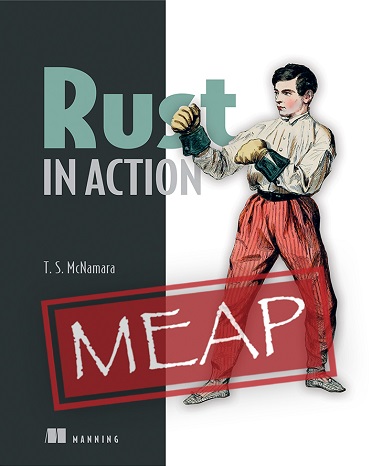
MEAP VERSION 16

About this MEAP
You can download the most up-to-date version of your electronic books from your Manning Account at .
Manning Publications Co. We welcome reader comments about anything in the manuscript - other than typos and other simple mistakes. These will be cleaned up during production of the book by copyeditors and proofreaders.
https://livebook.manning.com/#!/book/rust-in-action/discussion
Welcome
Dear Reader,
Thanks for taking a chance and buying this early release book on the Rust programming language and the internals of computer systems. I hope that you'll be rewarded with a fun, informative read!
Part 1: Rust Language Distinctives will provide a quick-fire introduction to the Rust language by working through projects that begin to introduce concepts that are expanded upon later in the book, such as implementing a File API.
Part 2: Systems Programming from the Ground Up (Almost) will shift your focus towards the computer. You will learn how data is represented at various levels between the application, operating system and hardware. Rust language features will be introduced as required.
A note about Chapter 6: This chapter should be considered a work in progress. It covers lots of ground. Readers progress from learning what a pointer is to benchmarking memory allocations with system utilities for Linux. Some of the explanations are terse... perhaps too terse.
We believe that the content is interesting and relevant to professional programmers, but are considering how it should be expanded and refactored to be most relevant to the book's readers. The author and the editorial team are very interested in your views about what works and what needs more work.
Part 3: Concurrent and Parallel Programming will walk through the tools that computers offer to get work done in parallel. Its primary goal will be to explain how multiple threads can cooperate and coordinate. A large example building a graph that can be distributed in multiple wayswill be extended throughout several chapters.
Finally, Part 4: Extending Applications Up and Out will show Rust being used to build up software components to extend large software projects. These projects demonstrate systems programming with the system rather than systems programming of the system. Part 4 will deal with the complexities of handling multiple CPU/OS pairs from the same code base.
As a MEAP reader, you have a unique opportunity to contribute to the book's development. All feedback is greatfully received, but please remember that there will be a human receiving the message. The best channel is the Manning liveBook's Discussion Forum. I'm also active on Twitter (@timClicks), Reddit (/u/timclicks) and the Rust Discourse forum (https://users.rust-lang.org/u/timclicks).
Thank you once again for choosing to purchase the book. It's a privilege to ride a short way with you along your computing journey.
Tim McNamara

Brief Table of Contents
Part 1: Rust Language Distinctives
Part 2:Demystifying Systems Programming
1 Introducing Rust
This chapter covers:
Introducing Rusts features and goals
Exposing Rusts syntax
Discussing where to use Rust and when to avoid it
Building your first Rust program
Explaining how Rust compares to object-oriented and wider languages
Welcome to Rustthe empowering programming language. Once you scratch its surface, you will not only find a programming language with unparalleled speed and safety, but one that is still comfortable to use. Your investment in learning Rust will be rewarded!
When you begin to program in Rust, its likely that you will want to continue to do so. And this book, Rust in Action , will build your confidence as a Rust programmer. But, it will not teach you how to program from the beginning. This book is intended to be read by people who are considering Rust as their next language, and for those who enjoy implementing practical working examples. Here is a list of some of the larger examples this book covers:
Mandelbrot set renderer
A grep clone
CPU emulator
Generative art
A database
HTTP, NTP, and hexdump clients
LOGO language interpreter
Operating system kernel
As you may gather from scanning through that list, reading this book will teach you more than Rust. It also introduces you to systems programming and low-level programming . Learning some of the concepts from these areas will benefit your Rust programming journey because much of the Rust community assumes that you already have that knowledge. As you work through Rust in Action , youll learn about the role of an operating system (OS), how a CPU works, how computers keep time, what pointers are, and what a data type is. You will gain an understanding of how the computers internal systems interoperate. Learning more than syntax, you will also see why Rust was created and the challenges that it addresses.
1.1 Where is Rust used?
Rust has won the most loved programming language award in Stack Overflows annual developer survey in 2016, 2017, 2018, 2019, and 2020. Perhaps thats why large technology leaders such as the following have adopted Rust:
Amazon Web Services (AWS) runs its serverless computing offerings, AWS Lambda and AWS Fargate, with Rust.
Cloudflare develops many of its services, including its public DNS, serverless computing, and packet inspection offerings with Rust.
Dropbox rebuilt its backend warehouse, which manages exabytes of storage, with Rust.
Google develops portions of the Chrome OS and Fuchsia operating systems in Rust.
Facebook uses Rust to power Facebooks web, mobile, and API services, as well as parts of HHVM, the HipHop virtual machine used by the Hack programming language.
Microsoft writes components of its Azure platform including a security daemon for its Internet of Things (IoT) service in Rust.
Mozilla uses Rust to enhance the Firefox web browser, which contains 15 million lines of code. Mozillas first two Rust-in-Firefox projects, its MP4 metadata parser and text encoder/decoder, led to overall performance and stability improvements.
GitHubs npm, Inc., uses Rust to deliver upwards of 1.3 billion package downloads per day.`"
Oracle developed a container run time with Rust to overcome problems with the Go reference implementation.
Samsung, via its subsidiary SmartThings, uses Rust in its Hub, which is the firmware backend for its Internet of Things (IoT) service.
Rust is also productive enough for fast-moving startups to deploy it. Heres a few examples:
Sourcegraph uses Rust to serve syntax highlighting across all of its languages.
Figma employs Rust in the performance-critical components of its multi-player server.
Parity develops its client to the Ethereum blockchain with Rust.
As well, Rust supports WebAssembly (Wasm), a standard for deploying apps as a first-class citizen into browsers without JavaScript. Wasm allows you to compile and deploy your Rust project to a server, IoT devices, mobile devices, and a browser.
Next page