The Rust Project Developpers - The Rust Programming Language
Here you can read online The Rust Project Developpers - The Rust Programming Language full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2017, publisher: The Rust Project Developpers, genre: Home and family. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
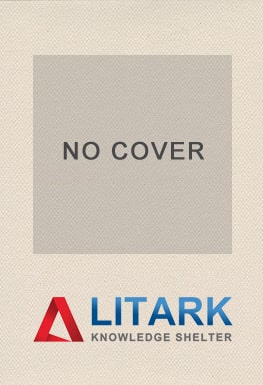
- Book:The Rust Programming Language
- Author:
- Publisher:The Rust Project Developpers
- Genre:
- Year:2017
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
The Rust Programming Language: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "The Rust Programming Language" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
The Rust Programming Language — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "The Rust Programming Language" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
The Rust Programming Language
Welcome! This book will teach you about the Rust Programming Language. Rust is a systems programming language focused on three goals: safety, speed, and concurrency. It maintains these goals without having a garbage collector, making it a useful language for a number of use cases other languages arent good at: embedding in other languages, programs with specific space and time requirements, and writing low-level code, like device drivers and operating systems. It improves on current languages targeting this space by having a number of compile-time safety checks that produce no runtime overhead, while eliminating all data races. Rust also aims to achieve zero-cost abstractions even though some of these abstractions feel like those of a high-level language. Even then, Rust still allows precise control like a low-level language would.
The Rust Programming Language is split into chapters. This introduction is the first. After this:
- - Set up your computer for Rust development.
- - Learn some Rust with a small project.
- - Each bit of Rust, broken down into small chunks.
- - Higher-level concepts for writing excellent Rust code.
- - A reference of terms used in the book.
- - Background on Rusts influences, papers about Rust.
The source files from which this book is generated can be found on GitHub.
There are two editions of The Rust Programming Language, this being the first edition.
The is a complete re-write. It is still under construction, though it is far enough along to learn most of Rust. We suggest reading the second edition and then checking out the first edition later to pick up some of the more esoteric parts of the language.
Getting Started
This first chapter of the book will get us going with Rust and its tooling. First, well install Rust. Then, the classic Hello World program. Finally, well talk about Cargo, Rusts build system and package manager.
Well be showing off a number of commands using a terminal, and those lines all start with $
. You dont need to type in the $
s, they are there to indicate the start of each command. Well see many tutorials and examples around the web that follow this convention: $
for commands run as our regular user, and #
for commands we should be running as an administrator.
Installing Rust
The first step to using Rust is to install it. Generally speaking, youll need an Internet connection to run the commands in this section, as well be downloading Rust from the Internet.
The Rust compiler runs on, and compiles to, a great number of platforms, but is best supported on Linux, Mac, and Windows, on the x86 and x86-64 CPU architecture. There are official builds of the Rust compiler and standard library for these platforms and more. For full details on Rust platform support see the website.
All you need to do on Unix systems like Linux and macOS is open a terminal and type this:
$ curl https:// sh .rustup.rs -sSf | shIt will download a script, and start the installation. If everything goes well, youll see this appear:
Rust is installed now. Great!Installing on Windows is nearly as easy: download and run rustup-init.exe. It will start the installation in a console and present the above message on success.
For other installation options and information, visit the install page of the Rust website.
Uninstalling Rust is as easy as installing it:
$ rustup self uninstallIf weve got Rust installed, we can open up a shell, and type this:
$ rustc --versionYou should see the version number, commit hash, and commit date.
If you do, Rust has been installed successfully! Congrats!
If you dont, that probably means that the PATH
environment variable doesnt include Cargos binary directory, ~/.cargo/bin
on Unix, or %USERPROFILE%\.cargo\bin
on Windows. This is the directory where Rust development tools live, and most Rust developers keep it in their PATH
environment variable, which makes it possible to run rustc
on the command line. Due to differences in operating systems, command shells, and bugs in installation, you may need to restart your shell, log out of the system, or configure PATH
manually as appropriate for your operating environment.
Rust does not do its own linking, and so youll need to have a linker installed. Doing so will depend on your specific system. For Linux-based systems, Rust will attempt to call cc
for linking. On windows-msvc
(Rust built on Windows with Microsoft Visual Studio), this depends on having Microsoft Visual C++ Build Tools installed. These do not need to be in %PATH%
as rustc
will find them automatically. In general, if you have your linker in a non-traditional location you can call rustc linker=/path/to/cc
, where /path/to/cc
should point to your linker path.
If you are still stuck, there are a number of places where we can get help. The easiest is .
This installer also installs a copy of the documentation locally, so we can read it offline. Its only a rustup doc
away!
Hello, world!
Now that you have Rust installed, well help you write your first Rust program. Its traditional when learning a new language to write a little program to print the text Hello, world! to the screen, and in this section, well follow that tradition.
The nice thing about starting with such a simple program is that you can quickly verify that your compiler is installed, and that its working properly. Printing information to the screen is also a pretty common thing to do, so practicing it early on is good.
Note: This book assumes basic familiarity with the command line. Rust itself makes no specific demands about your editing, tooling, or where your code lives, so if you prefer an IDE to the command line, thats an option. You may want to check out SolidOak, which was built specifically with Rust in mind. There are a number of extensions in development by the community, and the Rust team ships plugins for various editors. Configuring your editor or IDE is out of the scope of this tutorial, so check the documentation for your specific setup.
First, make a file to put your Rust code in. Rust doesnt care where your code lives, but for this book, I suggest making a projects directory in your home directory, and keeping all your projects there. Open a terminal and enter the following commands to make a directory for this particular project:
$ mkdir ~ /projects$ cd ~ /projects$ mkdir hello_world$ cd hello_worldNote: If youre on Windows and not using PowerShell, the ~
may not work. Consult the documentation for your shell for more details.
We need to create a source file for our Rust program. Rust files always end in a .rs extension. If you are using more than one word in your filename, use an underscore to separate them; for example, you would use my_program.rs rather than myprogram.rs.
Now, make a new file and call it
Font size:
Interval:
Bookmark:
Similar books «The Rust Programming Language»
Look at similar books to The Rust Programming Language. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book The Rust Programming Language and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.