Dr Antonio Gulli - A Collection of Design Pattern Interview Questions Solved in C++
Here you can read online Dr Antonio Gulli - A Collection of Design Pattern Interview Questions Solved in C++ full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2014, publisher: CreateSpace Independent Publishing Platform, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
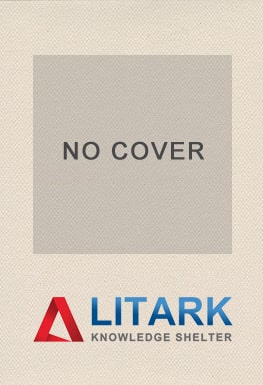
- Book:A Collection of Design Pattern Interview Questions Solved in C++
- Author:
- Publisher:CreateSpace Independent Publishing Platform
- Genre:
- Year:2014
- Rating:5 / 5
- Favourites:Add to favourites
- Your mark:
- 100
- 1
- 2
- 3
- 4
- 5
A Collection of Design Pattern Interview Questions Solved in C++: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "A Collection of Design Pattern Interview Questions Solved in C++" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
A Collection of Design Pattern Interview Questions Solved in C++ — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "A Collection of Design Pattern Interview Questions Solved in C++" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
A collection of Design Pattern Interview Questions Solved in C++ Antonio Gulli Copyright 201 4 Antonio Gulli All rights reserved. ISBN: ISBN-13: Design Patterns is the fourth of a series of 25 Chapters devoted to algorithms, problem solving, and C++ programming. DEDICATION To Aurora for her joy of life ACKNOWLEDGMENTS Thanks to Francesco Nidito for his code review Contents
In this book we will discuss three classes of Design patterns: 1) Creational patterns, which create objects on your behalf rather than instantiating them directly. 2) Structural patterns, which compose interfaces by leveraging inheritance. The composition of objects allows to create new functionalities, simplify interfaces, adapt heterogeneous objects, improve performances and reduce complexity. 3) Behavioural patterns, which are used to describe interaction and communication among objects. Behavioural patterns are also used to handle the internal state and the internal activities of each object.
This pattern allows to exchange concrete implementations with no need of changing the code that uses those implementations. This is because the factory typically returns an abstract pointer and the client does not care about internal details. In this example the class Widget has a pure virtual method. The two classes derived by the Widget are implementing a specific draw() behaviour. In this illustrative situation we just print a different text. class Widget { public : virtual void draw() = 0; // make it pure virtual }; class OSX_Button : public Widget { public : void draw() { std::cout << "\tOSX buttom" << std::endl; } }; class Windows_Button : public Widget { public : void draw() { std::cout << "\tWindows buttom" << std::endl; } }; }; // end Abstract Factory void testAbstractFactory(){ using namespace Creational_Patterns::Abstract_Factory; Widget * w = new OSX_Button (); w->draw(); delete w; }
Instead of using different constructors, the pattern defines a single object: the builder. This object builds the desired object step-by-step according to the configuration parameters defined by the user. The Builder is different from the Abstract factory because the former delegates the construction of the actual object to a specific auxiliary class/object, while the latter uses inheritance for exchanging concrete implementations. In this example the class Builder is implemented according to a pure virtual method configure which is defined in each subclass for setting up the parameters used to drive each subclass building process. A set of specific tests based on different conditions can be added to the skeleton code for driving the specific action items taken by the Simple Builder and by the Advanced Builder respectively. class Build { // base class public : virtual void configure() = 0; // here can add methods to see the internal state protected : int configuration_1_; // all the confs variable int configuration_2_; // all the confs variable //... int configuration_n_; // all the confs variable }; class SimpleBuilder : public Build { // a derived class public : SimpleBuilder() { // here you can add explicit tests on configuration // parameters for driving the simple builder // creation steps std::cout << "\tSimple Builder" << std::endl; }; void configure() { // here you can add additional configuration steps }; }; class AdvancedBuilder : public Build { // a derived class public : AdvancedBuilder() { // here you can add explicit tests on configuration // parameters for driving the advanced builder // creation steps std::cout << "\tAdvanced Builder" << std::endl; }; void configure() { // here you can add additional configuration steps }; }; // the class that use multiple builders class ClientClass { public : ClientClass( Build * builder ) : builder_( builder ){}; private : Build * builder_; // stores the specific builder }; }; // end Builder void testBuilder(){ using namespace Creational_Patterns::Builder; Build * builder = new AdvancedBuilder (); Client c(builder); delete builder; }
Typically the factory pattern leverages the inheritance mechanism with the actual creation process delegated to subclasses. Factory is frequently used for avoiding duplication of code and for abstracting common functionalities. In this sense a superclass specifies generic behaviours by using pure virtual placeholders for creation steps. Then the superclass delegates the creation details to subclasses that are supplied by the client. Factory pattern s can be seen as a simplified version of an Abstract Factory pattern. The Factory Method pattern is responsible of creating products that belong to one family, while the Abstract Factory pattern deals with multiple families of products.
Next pageFont size:
Interval:
Bookmark:
Similar books «A Collection of Design Pattern Interview Questions Solved in C++»
Look at similar books to A Collection of Design Pattern Interview Questions Solved in C++. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book A Collection of Design Pattern Interview Questions Solved in C++ and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.