Dr Antonio Gulli - A Collection of Graph Programming Interview Questions Solved in C++
Here you can read online Dr Antonio Gulli - A Collection of Graph Programming Interview Questions Solved in C++ full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2015, publisher: CreateSpace Independent Publishing Platform, genre: Home and family. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
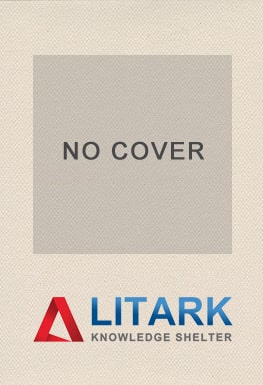
- Book:A Collection of Graph Programming Interview Questions Solved in C++
- Author:
- Publisher:CreateSpace Independent Publishing Platform
- Genre:
- Year:2015
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
A Collection of Graph Programming Interview Questions Solved in C++: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "A Collection of Graph Programming Interview Questions Solved in C++" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
A Collection of Graph Programming Interview Questions Solved in C++ — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "A Collection of Graph Programming Interview Questions Solved in C++" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
A collection of Graph Programming Interview Questions Solved in C++ Antonio Gulli Copyright 2015 Antonio Gulli All rights reserved. ISBN: ISBN-13: Graph is the second of a series of 25 Chapters devoted to algorithms, problem solving and C++ programming. DEDICATION To my father Elio and my mother Maria. For your priceless help during all my life ACKNOWLEDGMENTS Thanks to Gaetano Mendola for code reviewing Table of Contents





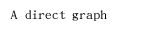
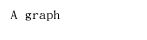


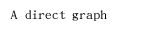
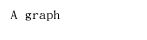
In addition a collection of nodes is memorized in a and all the edges originating from each node are memorized in a
. This representation is known as Adjacency list . In this particular implementation each node has a unique identifier NodeID , a label NodeWeight and another label representing the name of the node. Indeed each edge stores the destination NodeID , a label EdgeWeightI representing the weight of the edge and another label representing the name of the edge. The second direct graph implementation represents a Graph using a matrix of size
where n is the number of nodes.
Font size:
Interval:
Bookmark:
Similar books «A Collection of Graph Programming Interview Questions Solved in C++»
Look at similar books to A Collection of Graph Programming Interview Questions Solved in C++. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book A Collection of Graph Programming Interview Questions Solved in C++ and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.