Steve Klabnik and Carol Nichols - The Rust Programming Language
Here you can read online Steve Klabnik and Carol Nichols - The Rust Programming Language full text of the book (entire story) in english for free. Download pdf and epub, get meaning, cover and reviews about this ebook. year: 2017, genre: Computer. Description of the work, (preface) as well as reviews are available. Best literature library LitArk.com created for fans of good reading and offers a wide selection of genres:
Romance novel
Science fiction
Adventure
Detective
Science
History
Home and family
Prose
Art
Politics
Computer
Non-fiction
Religion
Business
Children
Humor
Choose a favorite category and find really read worthwhile books. Enjoy immersion in the world of imagination, feel the emotions of the characters or learn something new for yourself, make an fascinating discovery.
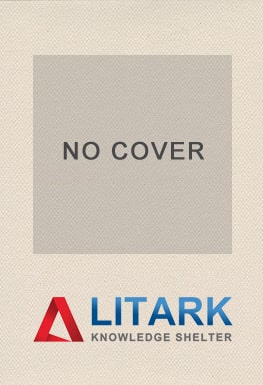
- Book:The Rust Programming Language
- Author:
- Genre:
- Year:2017
- Rating:4 / 5
- Favourites:Add to favourites
- Your mark:
- 80
- 1
- 2
- 3
- 4
- 5
The Rust Programming Language: summary, description and annotation
We offer to read an annotation, description, summary or preface (depends on what the author of the book "The Rust Programming Language" wrote himself). If you haven't found the necessary information about the book — write in the comments, we will try to find it.
The Rust Programming Language — read online for free the complete book (whole text) full work
Below is the text of the book, divided by pages. System saving the place of the last page read, allows you to conveniently read the book "The Rust Programming Language" online for free, without having to search again every time where you left off. Put a bookmark, and you can go to the page where you finished reading at any time.
Font size:
Interval:
Bookmark:
Introduction
Welcome to The Rust Programming Language, an introductory book about Rust. Rust is a programming language thats focused on safety, speed, and concurrency. Its design lets you create programs that have the performance and control of a low-level language, but with the powerful abstractions of a high-level language. These properties make Rust suitable for programmers who have experience in languages like C and are looking for a safer alternative, as well as those from languages like Python who are looking for ways to write code that performs better without sacrificing expressiveness.
Rust performs the majority of its safety checks and memory management decisions at compile time, so that your programs runtime performance isnt impacted. This makes it useful in a number of use cases that other languages arent good at: programs with predictable space and time requirements, embedding in other languages, and writing low-level code, like device drivers and operating systems. Its also great for web applications: it powers the Rust package registry site, crates.io! Were excited to see what you create with Rust.
This book is written for a reader who already knows how to program in at least one programming language. After reading this book, you should be comfortable writing Rust programs. Well be learning Rust through small, focused examples that build on each other to demonstrate how to use various features of Rust as well as how they work behind the scenes.
This book is open source. If you find an error, please dont hesitate to file an issue or send a pull request on GitHub. Please see CONTRIBUTING.md for more details.
The first step to using Rust is to install it. Youll need an internet connection to run the commands in this chapter, as well be downloading Rust from the internet.
Well be showing off a number of commands using a terminal, and those lines all start with $
. You dont need to type in the $
character; they are there to indicate the start of each command. Youll see many tutorials and examples around the web that follow this convention: $
for commands run as a regular user, and #
for commands you should be running as an administrator. Lines that dont start with $
are typically showing the output of the previous command.
If youre on Linux or a Mac, all you need to do is open a terminal and type this:
$ curl https://sh.rustup.rs -sSf | shThis will download a script and start the installation. You may be prompted for your password. If it all goes well, youll see this appear:
Rust is installed now. Great!Of course, if you disapprove of the curl | sh
pattern, you can download, inspect and run the script however you like.
On Windows, go to https://rustup.rs and follow the instructions to download rustup-init.exe. Run that and follow the rest of the instructions it gives you.
The rest of the Windows-specific commands in the book will assume that you are using cmd
as your shell. If you use a different shell, you may be able to run the same commands that Linux and Mac users do. If neither work, consult the documentation for the shell you are using.
If you have reasons for preferring not to use rustup.rs, please see the Rust installation page for other options.
Once you have Rust installed, updating to the latest version is easy. From your shell, run the update script:
$ rustup updateUninstalling Rust is as easy as installing it. From your shell, run the uninstall script:
$ rustup self uninstallIf youve got Rust installed, you can open up a shell, and type this:
$ rustc --versionYou should see the version number, commit hash, and commit date in a format similar to this for the latest stable version at the time you install:
rustc x.y.z (abcabcabc yyyy-mm-dd)If you see this, Rust has been installed successfully! Congrats!
If you dont and youre on Windows, check that Rust is in your %PATH%
system variable.
If it still isnt working, there are a number of places where you can get help. The easiest is .
The installer also includes a copy of the documentation locally, so you can read it offline. Run rustup doc
to open the local documentation in your browser.
Any time theres a type or function provided by the standard library and youre not sure what it does, use the API documentation to find out!
Now that you have Rust installed, lets write your first Rust program. Its traditional when learning a new language to write a little program to print the text Hello, world! to the screen, and in this section, well follow that tradition.
Note: This book assumes basic familiarity with the command line. Rust itself makes no specific demands about your editing, tooling, or where your code lives, so if you prefer an IDE to the command line, feel free to use your favorite IDE.
First, make a directory to put your Rust code in. Rust doesnt care where your code lives, but for this book, wed suggest making a projects directory in your home directory and keeping all your projects there. Open a terminal and enter the following commands to make a directory for this particular project:
Linux and Mac:
$ mkdir ~/projects$ cd ~/projects$ mkdir hello_world$ cd hello_worldWindows:
> mkdir % USERPROFILE % \projects > cd % USERPROFILE % \projects > mkdir hello_world > cd hello_worldNext, make a new source file and call it main.rs. Rust files always end with the .rs extension. If youre using more than one word in your filename, use an underscore to separate them. For example, youd use hello_world.rs rather than helloworld.rs.
Now open the main.rs file you just created, and type the following code:
Filename: main.rs
fn main ( ) { println! ( " Hello, world! " ) ; }Save the file, and go back to your terminal window. On Linux or OSX, enter the following commands:
$ rustc main.rs$ ./mainHello, world!On Windows, run .\main.exe
instead of ./main
. Regardless of your operating system, you should see the string Hello, world!
print to the terminal. If you did, then congratulations! Youve officially written a Rust program. That makes you a Rust programmer! Welcome!
Now, lets go over what just happened in your Hello, world! program in detail. Heres the first piece of the puzzle:
fn main ( ) { }These lines define a function in Rust. The main
function is special: its the first thing that is run for every executable Rust program. The first line says, Im declaring a function named main
that has no parameters and returns nothing. If there were parameters, their names would go inside the parentheses, (
and )
.
Also note that the function body is wrapped in curly braces, {
and }
. Rust requires these around all function bodies. Its considered good style to put the opening curly brace on the same line as the function declaration, with one space in between.
Font size:
Interval:
Bookmark:
Similar books «The Rust Programming Language»
Look at similar books to The Rust Programming Language. We have selected literature similar in name and meaning in the hope of providing readers with more options to find new, interesting, not yet read works.
Discussion, reviews of the book The Rust Programming Language and just readers' own opinions. Leave your comments, write what you think about the work, its meaning or the main characters. Specify what exactly you liked and what you didn't like, and why you think so.